β tRPC failed on profile.getUserByUsername: No "query"-procedure on path "profile.getUserByUsername"
I am a bit lost.
This is the profileRouter.
export const profileRouter = createTRPCRouter({
getUserByUsername: publicProcedure
.input(z.object({ username: z.string() }))
.query(async ({ input }) => {
const [user] = await clerkClient.users.getUserList({
username: [input.username],
});
if (!user) {
// if we hit here we need a unsantized username so hit api once more and find the user.
const users = (
await clerkClient.users.getUserList({
limit: 200,
})
)
const user = users.find((user) => user.externalAccounts.find((account) => account.username === input.username));
if (!user) {
throw new TRPCError({
code: "INTERNAL_SERVER_ERROR",
message: "User not found",
});
}
return filterUserForClient(user)
}
return filterUserForClient(user);
}),
});
and where it's being called.
const ProfilePage: NextPage<{ username: string }> = ({ username }) => {
const { data } = api.profile.getUserByUsername.useQuery({
username,
});
if (!data) return <div>404</div>;
37 Replies
Did you add the router to the
root.ts
file?import { createTRPCRouter } from "npm/server/api/trpc";
import { postsRouter } from "./routers/posts";
import { profileRouter } from "./routers/profile";
/**
* This is the primary router for your server.
*
* All routers added in /api/routers should be manually added here.
*/
export const appRouter = createTRPCRouter({
posts: postsRouter,
profile: profileRouter,
});
// export type definition of API
export type AppRouter = typeof appRouter;
yeah
@Lermatroid
That is weird. Any type errors showing in your IDE?
(assuming the error in the title is from runtime)
no, Idk
in the ssg helper, import { createProxySSGHelpers } from "@trpc/react-query/ssg";
import { appRouter } from "npm/server/api/root";
import { prisma } from "npm/server/db";
import superjson from "superjson";
export const generateSSGHelper = () =>
createProxySSGHelpers({
router: appRouter,
ctx: { prisma, userId: null },
transformer: superjson, // optional - adds superjson serialization
});
the creatProxySSGHelper is deprected
its fine to switch right
to import { createServerSideHelpers } from "@trpc/react-query/server";
import { appRouter } from "npm/server/api/root";
import { prisma } from "npm/server/db";
import superjson from "superjson";
export const generateSSGHelper = () =>
createServerSideHelpers({
router: appRouter,
ctx: { prisma, userId: null },
transformer: superjson, // optional - adds superjson serialization
});
@Lermatroid
Not sure then, sorry :/
is the trpc client pointing to the correct place?
use 3 ` [backticks] to format your code.
otherwise it's too difficult to follow
profile router
profile page
Root
trcp
@Lermatroid @peterp
As I said I am not sure, please stop pinging me, thanks!
oh, sorry
my bad
All good π
still unresolved
Your code looks correct
Can u put it on github
comparing to a cloned version
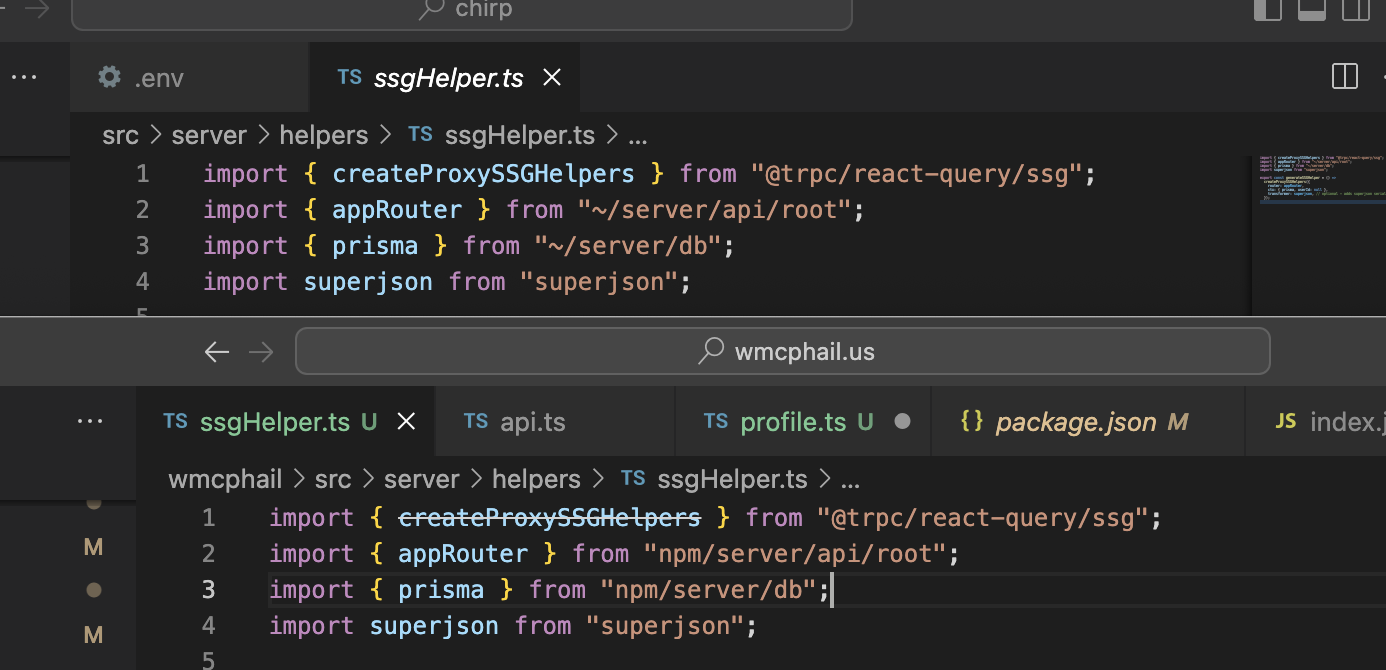
why is mine crossed out?
I think that's the issue
@abcdef
Change it to
import { createServerSideHelpers } from "@trpc/react-query/server";
i did try that
but why would it run on the cloned version and not mine?
What do you mean?
like I cloned theoβs version onto my machine and edited the env variables and it runs
yet mine (which is the exact same) does not work
why would it throw a deprecated error on mine and not on his?
His repo uses the old trpc version probably
createProxySSGHelpers got deprecated on April 6
hm even still though when i make the change it still bugs out
i can unprivate my repo and push if you wanna see
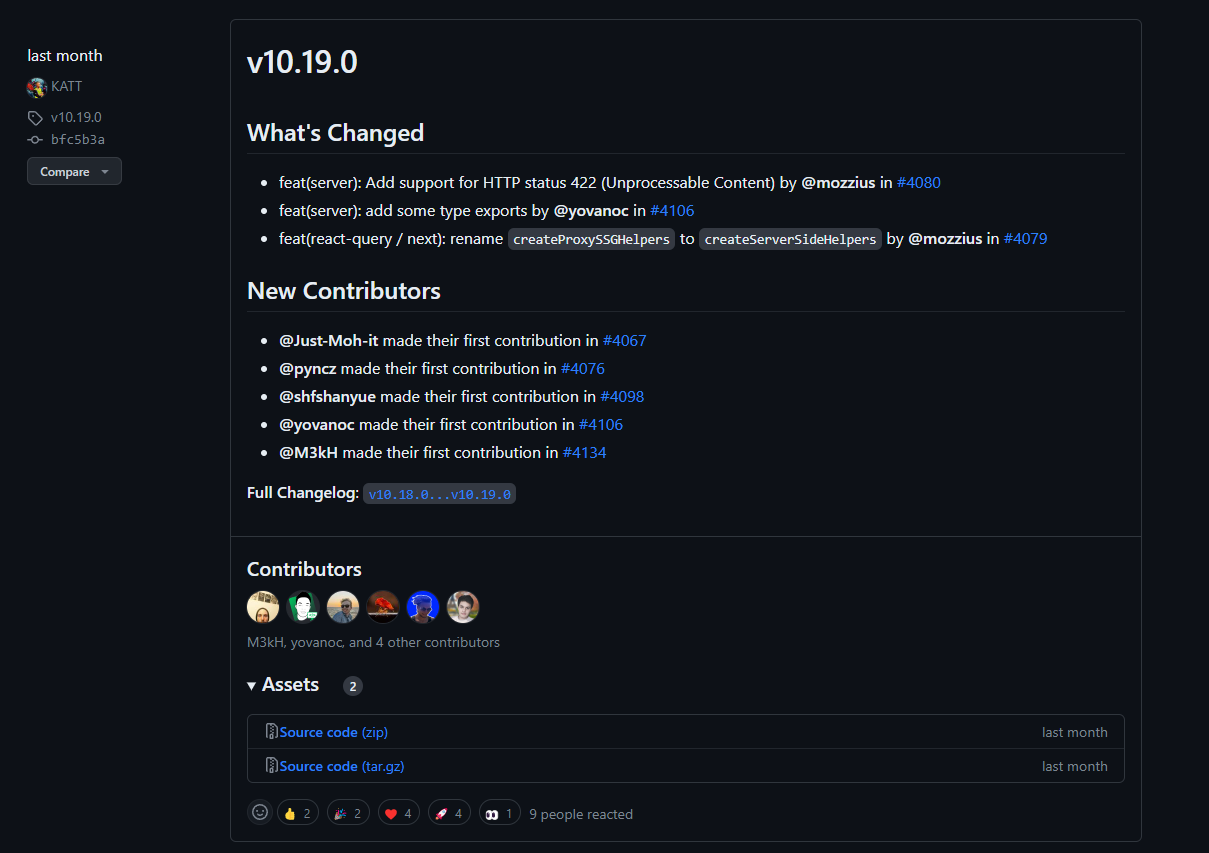
Sure
GitHub
GitHub - W-McPhail/wmcphailWeb
Contribute to W-McPhail/wmcphailWeb development by creating an account on GitHub.
@abcdef
Ok lemme take a look
Ok slight problem, you're not adding profileRouter to appRouter
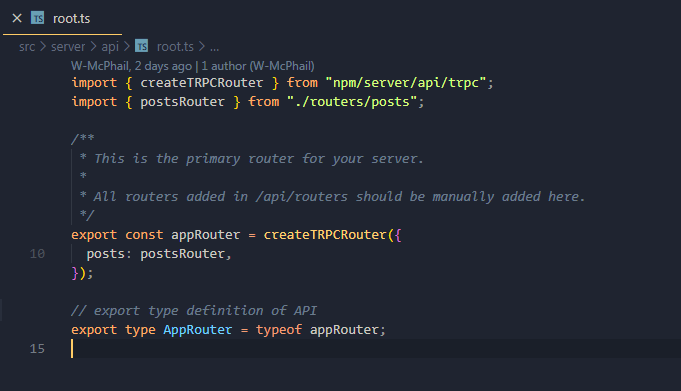
@TheTurtleChief
wait what I swear to god I was
In the code you sent on discord it was added
But not on github
tf?
this is what I have
GitHub
wmcphailWeb/root.ts at main Β· W-McPhail/wmcphailWeb
Contribute to W-McPhail/wmcphailWeb development by creating an account on GitHub.
Oh did you forget to push?
bruh im gonna cry
I didnt hit save
im so fucking dumb
well
Lmao
at least its over
thats 2 days of my life
so it goes
thank you bro
You're welcome
I've done the same mistake many times before too π
any idea how to fix this?
https://recordit.co/DMmGFVkRcR
the css loads normally and then like snaps back to be broken @abcdef