✅ help!
i need to write a program in a winforms app that takes a user input of radius to calculate the area and diameter of a circle. There must be a class separate from the main file with fields, properties and constructor. I have most of the program written, but I am struggling to understand how to pass the calculated area and diameter to the labels on my form. The program runs, but crashes when the button to calculate is pressed. The labels im passing the values too is label6 and label7.
Here is the code for the class file: public class Circle { static double _radius; static double _diameter; static double _area; public static double Area { get { return _area; } } public static double Diameter { get { return _diameter; } } public static double Radius { get { return Radius; } set { Radius = value; } } public Circle() { Radius = 1; } public Circle(double radius) { _radius = Radius; _area = Radius * Radius * Math.PI; _diameter = Radius * 2 }
Here is the code for the class file: public class Circle { static double _radius; static double _diameter; static double _area; public static double Area { get { return _area; } } public static double Diameter { get { return _diameter; } } public static double Radius { get { return Radius; } set { Radius = value; } } public Circle() { Radius = 1; } public Circle(double radius) { _radius = Radius; _area = Radius * Radius * Math.PI; _diameter = Radius * 2 }
382 Replies
And here's the code I have for the form:
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e)
{
}
private void label2_Click(object sender, EventArgs e)
{
}
private void label3_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
Circle.Radius = Convert.ToInt32(textBox1.Text);
Circle c = new Circle();
label6.Text = Convert.ToString(Circle.Area);
label7.Text = Convert.ToString(Circle.Diameter);
}
private void label7_Click(object sender, EventArgs e)
{
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
}
private void label8_Click(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
}
what's the error you're getting?
i would suggest using $code when pasting things in, it makes them easier to read
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/button1_Click is the calculate button, yeah?
yes
there is no error, program runs fine but crashes when i hit the calculate button
right
it crashes with an error, presumably
what's that error
here let me run it
it doesnt give me any errors, the program just crashes. the window pops up like its going to give me an error but nothing shows
here let me put my code into that thing you sent so its easier to read
what does it mean that it "pops up like it's going to give an error"
can you send a picture of what you are seeing
with the program running, hit up "Debug" => "Windows" => "Exception Settings"
make sure "Common Language Runtime Exceptions" is checked
then replicate a crash again
this is the form file. it doesnt show all of the code but i havent messed with anything except for button
and heres the error window after the program crashes
doesnt give me anything
nor should it
JakenVeina#1758
with the program running, hit up "Debug" => "Windows" => "Exception Settings"
Quoted by
<@!137791696325836800> from #help! (click here)
React with ❌ to remove this embed.
and just screenshot that window?
no
do what I said
i did lol a window popped up at the bottom
indeed
oh wait sorry
hold on
😉
it was already checked
k
let's set some breakpoints in that click handler and see what happens
wdym?
I mean go to the click handler
set some breakpoints
run the program
replicate a crash
and see which breakpoints get hit
or just set one on the first line and step through
im very new to C# i havent done what youre asking me to do yet. Im pretty sure I just have logic errors in my code or I'm not putting things in the right place
and im unsure of how to fix them
indeed
and we're going to learn how to debug
in order to spot those errors
the simplest way to set breakpoints is to click in the left-hand gutter of the file editor
you can also press F9 with your cursor at the spot where you want to break
and there's also a right-click context menu for breakpoints
is it really necessary to debug an app that displays two multiplication problems? I don't have time to learn a whole new concept right now man. I have to take finals tonight
yes
debugging is how you find logical errors in your code
when you can't spot them at a glance
why Visual Studio isn't debugging ALREADY when the crash occurs, I don't know
it definitely should
probably because the program is so simple
no
Visual Studio does not change its behavior based on how "Simple" it thinks your solution is
look man, im being respectful as possible when i say i do not have time to learn a whole new concept of programming tonight. I simply need someone to tell me how to properly pass values from the class I created to my winforms. Can you show me how to do that properly?
define "pass values"
public Circle(double radius)
{
_radius = Radius;
_area = Radius * Radius * Math.PI;
_diameter = Radius * 2
i need the values produced by these formulas to display in the app when the user inputs the radius
then you need to expose them for use by the consuming class
and then DO something with them, in that consuming class
something that makes them display in your form
all of which you're already doing
im not doing it right though
i know that
yes, you are
this retrieves text from the text box in your form named
textBox1
converts it to an int
implicitly casts it to double
and saves it into Circle.Radius
this accomplishes literally nothingok so there's our first problem
delete that?
this retrieves the value stored in
Circle.Area
, converts it to a string
and sets it to the .Text
property of the label on your form named label6
which means the label will now display that text valueso why doesn't it work?
and similar for
Circle.Diameter
and label7
I don't know
I don't even know what's actually going WRONG
because you're not getting feedback from the debugger
your first problem is that you don't know what your problem isdid you look at the class file? is everything right there?
I could probably spend an hour discussing what's wrong in there, that's a bogus question
you have an application crash
we need to identify it
this doesnt even make sense, youre saying i need to debug a program with 20 lines of code yet you could spend an hour discussing whats wrong with it?
indeed
okay
can i just zip the program and send it to you?
no
no one here is going to do your homework for you
dude the entire fucking thing is written
you're being no help at all you're just being a dick
specific instructions on what to do to move forward doesn't count as help?
youre saying theres all this stuff wrong with my program yet you wont tell me what it is. you're asking me to learn how to debug when this program is probably less than 30 lines of code and ALL i need to do is display TWO calculations from TWO formulas to the user. it really should not be this difficult. i dont need to debug my program. the fix is probably very fucking simple.
probably
putting a breakpoint in your code will show what the problem is
in the time you've spent arguing about it, you could have done the couple of clicks I asked you to do, and we could have identified the problem and be working on solving it
alright, FINE. how and where do i put a breakpoint
you can drop the attitude btw
JakenVeina#1758
the simplest way to set breakpoints is to click in the left-hand gutter of the file editor
Quoted by
<@!137791696325836800> from #help! (click here)
React with ❌ to remove this embed.
remember that we're all helping YOU with your homework
or rather, helping you get a good job
recognize that before you get upset
there should be a little white circle that appears under your cursor when you're in the right spot
this is what you're going for
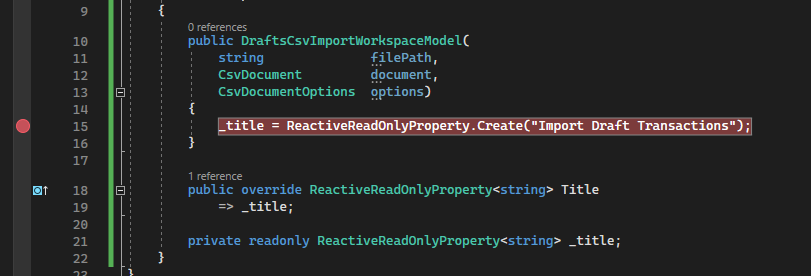
it just seems ridiculous to me that you guys are forcing me to debug when i havent learned how to do that yet and obviously havent needed to do it yet because the programs ive learned to write so far are very simple. each time i had an issue like this, my teacher told me what the issue was in 10 seconds. and this is the same kind of simplistic coding ive always been doing. i was expecting you guys to instantly recognize what the problem was, there's really not that much to look at. im not coding a video game
well
you've come here stating that your application is crashing
and you don't know why
first step is to identify what is causing the crash
we can reasonably assume it's in the click handler, cause that's what triggers the crash
but nothing is obviously wrong with that code
ok, so how many of these should i put?
investigating the non-obvious is best done with a debugger
let's be safe and put one on each line
then run the program and trigger the crash
ok
im running it now
what am i looking for?
Visual Studio should pause, and the line it's paused on should light up yellow
its not giving me anything
everything stays red
does the program just crash?
yes
how are you running the program?
F5
:/
are you building in release mode?
wait
i got one error
oh?
in the Error List?
no a yellow line
aha
it doesnt even make sense though
this is like one of the default lines
i havent even touched
well, is that one of the breakpoints, or is it an exception
what does the toolbar at the top of VS look like?
that's not something that should be breakpointable, I'm not sure why it's being highlighted
okay, yeah, you're definitely paused on SOMETHING
double-click that line in the bottom-right in the "Call Stack" window
the one the yellow arrow is pointing at
that should take you to the line of source code that it's paused on
its this one
well, that's just nonsense, cause that's not an executable line
on the bottom-left, in the "Locals" tab, is there an entry for "$exception"?
no
show me what you're seeing in the Form1 file
no, like, the source file
like, the rest of it, not just that one line
that's really weird
that is what a paused breakpoint looks like
by all rights, it shouldn't let you put a breakpoint there
whatever, click "Continue":
up at the top
like i said, i do not think the fix is very complicated. i think something is just not in the right place or im missing a line of code. you know how to program pretty well it seems, couldn't use just look over the code i sent and see if im missing something or need to move something to a different place?
you**
there's a handful of things I think could be the problem
they all need some amount of debugging to confirm
for example, you could have an exception from
Convert.ToInt32()
if you're passing it bad text
I have no idea what the text being passed in
best way to know for sure is set a breakpoint and checki put a breakpoint on every line of code it let me
right
the ones we're interested in are in the click handler, so
get us to that point
ok
where is the click handler?
the handler method for the click event that triggers the crash
tell me in caveman language please. i have not learned this yet
I mean, you wrote it
the button has a click event
and it calls the method that you attached to that click event when the event occurs
then again, maybe it doesn't
presumably you have that setup in the visual designer?
the click-and-drag UI for the form
yes
thats whats wrong with it. the code there isnt right
in
button1_Click
yes?yes
that's the method that's assigned to the button?
private void button1_Click(object sender, EventArgs e)
{
Circle.Radius = Convert.ToInt32(textBox1.Text);
Circle c = new Circle();
label6.Text = Convert.ToString(Circle.Area);
label7.Text = Convert.ToString(Circle.Diameter);
}
yes, that
assuming you set that method to be the handler for the button click event
yes thats the only button. and theres only one class. one constructor. getters and setters
so, yeah, that's where we wanna be with the breakpoints
i put them on everything
right
so
get us there
both form1 and the class file
like, run the application and trigger a crash
when you click the button, it should take us to the first breakpoint in that method
i already did this like 5 times with breakpoints on every line of code i wrote for this program
the only error it gave me was the one i showed you earlier
there hasn't been an error yet
that was the program pausing on that line
ok you know what i mean though
so what do i do next
I mean, no offense, but no, I don't fully
perils of communicating over text
then why did you immediately point it out?
the pause on
public Form1()
?
I didn't, you didokay
what do i do next
you have a breakpoint on the
Circle.Radius =
line?
it's red?yes i have them on every line of code it let me attach it too
so, we need to get to that line
run the program and get to a point where you can click that button, and do it
that line should be the one that turns yellow
if you want to simplify, go to "Debug" => "Delete All Breakpoints"
and then you can set just that one line
so just set that one line as a breakpoint?
yeah
or at least, all the lines in that one method
you definitely don't need the rest
ok
it highlighted in yellow this time
what niow
lovely
you should be able to see a bunch of interesting stuff in the "Locals" window
you can also hover over certain things to see what their values are
what's the value of
textBox1.Text
for example?you can hover over
textBox1.Text
or you can expand this
it'll be under therewhat am i looking for
what is the value of
textBox1.Text
hover your mouse over it30
great
so, not something that's gonna cause an exception from Convert.ToInt32
up near the top, near the bug run/continue button, there's some buttons with blue arrows
these control the debugger
there's one that points downward, and should say "Step Into" when you hover on it
we're going to click that to step through your code line-by-line until we spot the problem
okay. first thing highlighted is this
eyyyyy
an Exception
an Exception that even explains really weird crashing behavior, where you don't get a normal exception report from VS
do you know how to fix it?
yeah, don't overflow your stack
lol
can you spot what's happening?
you might be able to see it from the "Call Stack" window
no i dont. i assume im missing some code there. the last time i did this i did it in a console app but i wasnt sure how to write it for winforms so i left it out
well, let's walk it manually, then
we start with
Circle.Radius =
which calls the Radius
set
method, within the Circle
class
what does that method do?nothing im guessing how i wrote it. all the formulas are done in the constructor though
oh, it does something, though
what's it doing?
its setting the radius as whatever value inputted by the user and returning it to the function so it can use said value
I mean, that's what you wanted it to do, functionally
what it's actually doing, code-wise, is calling a set function
the
set
function of the Raidus
property on the Circle
class
that's what the syntax Property = value;
doesso can you tell me how to fix it man? look, i really appreciate your wisdom but i have to get up at 7 tomorrow and its super late here
_radius = value;
what you wrote is an infinite recursive loop
you're calling the setter from within the setter
when you're done with this, read up on how properties workProperties - C# Programming Guide
A property in C# is a member that uses accessor methods to read, write, or compute the value of a private field as if it were a public data member.
its giving me another error
or
yellow line
which one?
I mean, that's not new
that's the breakpoint you had set from last time
you're re-running the project now, right?
after changing that line of code?
yes
okay so
its displaying values now where they should but its only giving me 0's
well, that's progress
the labels for the area and diameter values?
theyre on this one
yes, I know
I was asking you if that's what you meant
by "its display values now where they should but its only giving me 0's"
oh yes
sorry
so
either A) your code to display the values in the UI is wrong
or B) your code to calculate the values is wrong or not working
im pretty sure its A
you can pick one to investigate
or you can use a debugger to confirm
if you think it's A, well, you can go look up what the proper way to display text with a label is
and see that it's by setting
.Text
on the label, which is what you're doing
so, perhaps you're setting the wrong labels?
you'd need to debug to investigate thati have .Text on both of them
yes, you do
wait a second
nevermind
the two labels have issues but when i step into them it doesnt take me anywhere
yeah, Step Into won't take you into code that isn't yours, by default
most notably, the debugger doesn't have the source code files for that stuff
that's built-in to .NET
there's ways to set that up, but that's not what you want
you can safely assume the labels are not bugged
do you know what the problem with it is man? im so fucking tired. if the last issue was as simple as missing an underscore then i am SURE that this is REALLY not that complicated
so, you need to confirm what values you're telling them to display
suffice it to say "not A"
the labels are displaying "0" because that's the values you told them to display
okay so how do i fix it
figure out why the values are 0, instead of whatever you want them to be
where are you calculating those values?
in the constructor
and where does that get called?
wait a second
do i have to call them with underscores?
no that doesnt work
where does that get called?
by the labels right?
no
the labels don't call into your code
you call into the labels
via the
.Text
settercan you show me how to do it. this is literally the first winforms app ive ever done ive only done console apps
the code you wrote to do those calculations isn't going to run if you're not running it
good news, this has nothing to do with WinForms
this would be wrong in a Console app
Well
I was using this as a reference
Because I had to write literally the exact same program in a console app
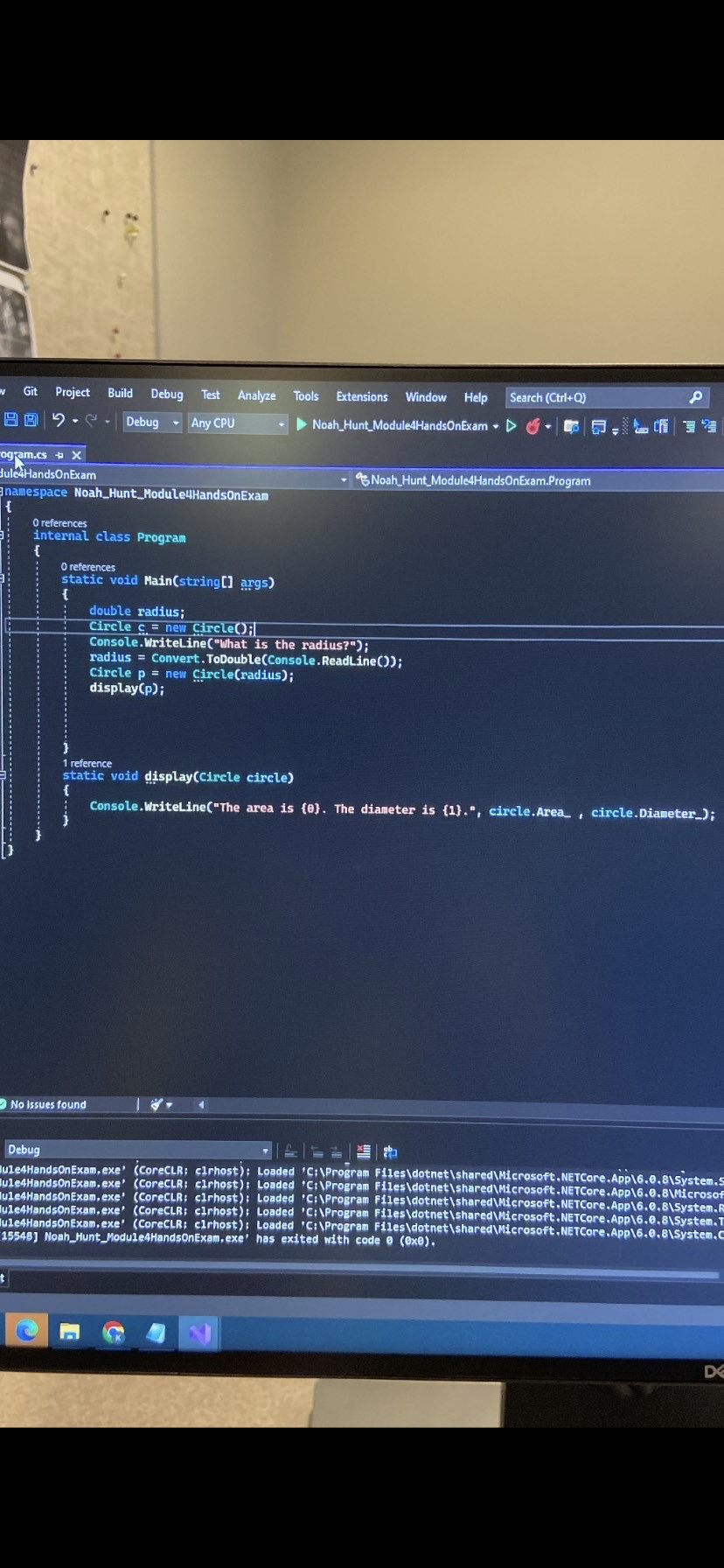
well, that's a great reference point
So what am I missing?
if that one works, what's different between the two?
alternatively, answer my leading question
where is that code called?
IDK MAN OK
okay
how would you call that code?
I’ve been up for 11 hours doing stuff for this class my brain is completely fried
By instantiating
great
you know what that looks like?
where does that happen?
Circle c = new Circle();
and which constructor is that calling?
Do I have to put something in the parentheses
do you?
does the constructor you want to call have parameters?
Idk
Is it Radius?
go look at it and see
which
Radius
?
there are at least 2 identifiers with the name Radius
in your programthis
that is a parameter, yes
so its radius?
Raidus
in the context of that screenshot is a parameter to that constructorIt gives me a red squiggly when I put it in there
put what where?
Radius in the parentheses
in which parentheses?
.
sure, in that context
Radius
is not defined
doesn't exist
what is the value you want to pass to the constructor?
as the Radius
parameter?The user input radius
great
do that
Dude
If I knew how to do it
I wouldn’t be asking for your help
you're doing it already
how do you retrieve the user input?
Can you please just tell me what I need to do
no
Why man
stop asking
because this is for coursework
and that's the #info-and-rules
as it should be
I will lead you to the answer to the best of my ability
spoonfeeding police are here to enforce 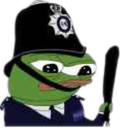
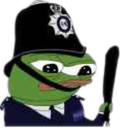
From the text box
why are we instantiating the
Circle
at all if all its members are static? or are we getting to that partsomehow I don't think we're receptive to best practices recommendations right now
Dude that’s literally the only way it would run
I tried messing with everything the main file doesn’t recognize it unless it’s static
incorrect
if it doesn't recognize it when it's not static, you're not using it right
Okay. Let’s just focus on helping me to get it work please
I really need to sleep
i mean this is kind of related, if you try to make 2 different circles they're going to share the same values for everything
and it's also clearly throwing off your other code
I’m assuming I need to put something in the parentheses ^
correct
I tried radius but it doesn’t work
"i tried radius" and "it doesn't work" are too unspecific for me to do anything with
screenshot what you did
to which I responded "what value do you want to pass to the constructor"
The user inputted radius
So there’s something im missing in the constructor
Yes?
and how do you retrieve that
Would it be inside of the constructor?
define "inside of the constructor"
like it would be a line of code inside the constructor to retrieve the value?
why did you declare your constructor to accept an argument?
as in, you want to put the code to retrieve the user input inside the constructor body?
Yes
you could of you wanted to
a circle should not know anything about your user interface
it's a circle
^
your code is 99% of the way there
I’m so confused right now it’s not even funny. I’m trying so hard to figure out what I need to do and I’m just blanking
do you understand why you defined your constructor to accept an argument called
Radius
?
a constructor is practically just like a methodBecause I need to call it somehow in the main file yes? And I assume that it’s something to do with those parentheses
yes, think of it like it's a plain method
So what am I missing? I’m defining radius as the parameter in there and it’s giving me an error. But it won’t tell me why
it is definitely telling you why
but i can't help based on "an error"
what you're missing is actually passing it the value you want to use as the radius
you're not defining radius as a parameter
you're passing in something called radius AS the parameter vLue
*value
It says the name radius does not exist in the current context
but radius isn't a thing you've defined in that context
what value DO you want to pass?
So do I have to declare a whole new variable?
you make it sound like a lot of work
do you?
Like I did in the other one
stop derailing yourself
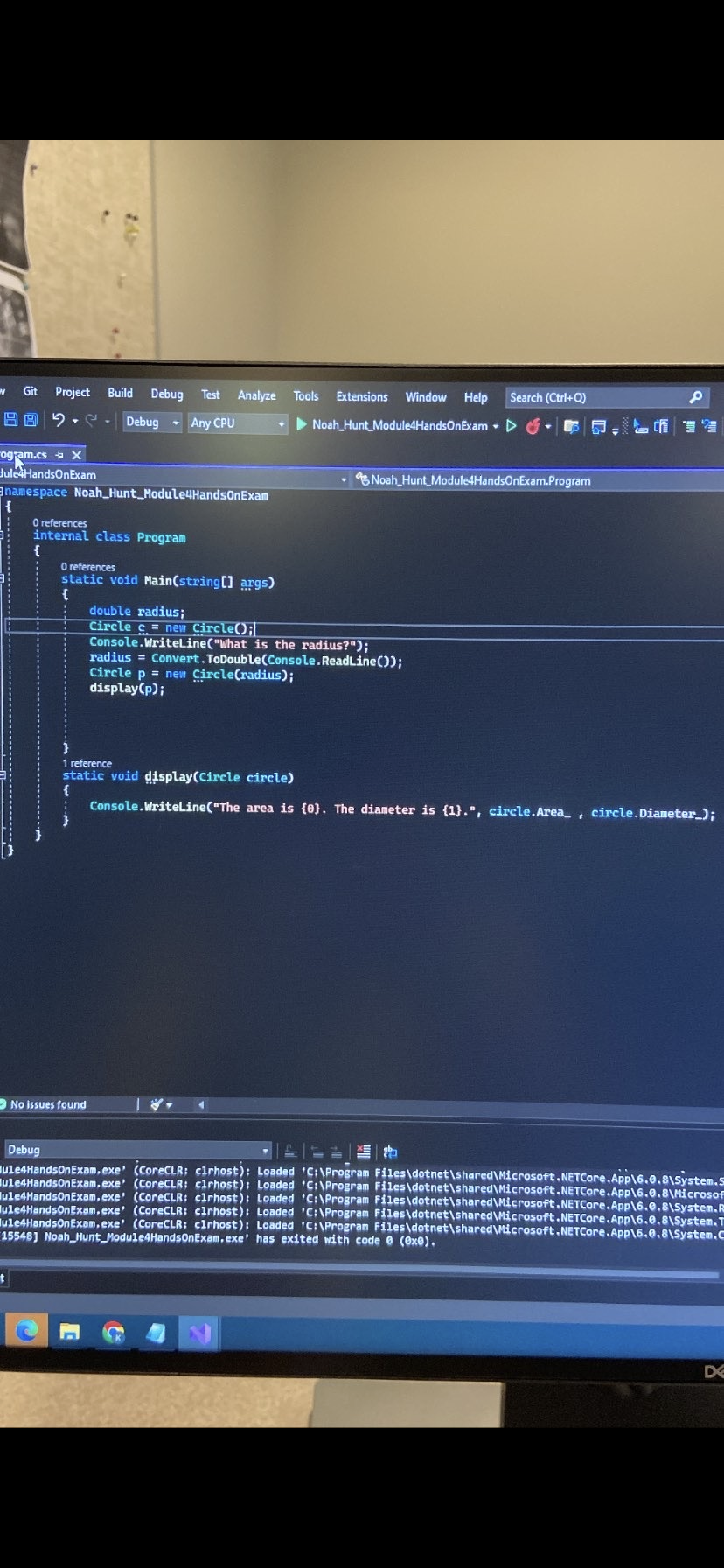
Like in this one?
there's a question on the table
answer it
what value do you want to pass?
Radius!!!;!3?3?3?3?
which radius? from where?
who gave it to you?
I PUT IT IN THE CONSTRUCTOR
I’m gonna see what happens if I do it like this
go for it
Nothing man
as in?
It doesn’t work
How I had it before was right
i can't do anything with "it doesn't work"
I’m missing something fucking stupid like one fucking word somewhere
frankly, yes
yup
that is exactly the problem
Can you atleast tell me
If it’s in the class
Or the main
is what where?
and the reason is that you have very little grasp of basic C# syntax
"it" is wherever you put it, whatever it is
you guys are impossible
you have a working example of the pattern you need to follow
just adapt it to your form and use the value the user is giving you
Yea man I’ve been coding for like 2 months
This vague talk you guys are giving me is just confusing me more. I don’t know how to fix these issues yet because I don’t know what it looks like to be RIGHT in practice with this
you're giving us very little to work with
"it doesn't work" "an error" "it's not telling me what's wrong"
I sent so many screenshots
Scroll up or tell me what you need to see
what do you currently have in your method?
public class Circle
{
static double _radius;
static double _diameter;
static double _area;
public static double Area
{
get { return _area; }
}
public static double Diameter
{
get { return _diameter; }
}
public static double Radius
{
get { return Radius; }
set
{
_radius = value;
}
}
public Circle(double Radius) { _radius = Radius; _area = Radius * Radius * Math.PI; _diameter = Radius * 2;
} public Circle() { Radius = 1; }
public Circle(double Radius) { _radius = Radius; _area = Radius * Radius * Math.PI; _diameter = Radius * 2;
} public Circle() { Radius = 1; }
that's... not a method
i mean the code that isn't working
your circle is fine
can you explain what each of those first 2 lines are doing?
because the answer is literally right there
the first line takes the input from the user as radius
the second one instantiates the circle class
the input from the user as radiusThat thing you keep saying is what you want to pass to the constructor as the parameter?
part of the problem here is that you made all your circle's properties static and it's 100% confusing you
it absolutely is
but you can fix this without changing that for now
static was the only way i could get it to work
incorrect
not saying it wouldnt work otherwise
doesn't matter, just focus on the current issue
so your first line gets the user's radius and puts it in
Circle.Radius
, right?yes
and your constructor needs the radius passed to it, right?
yes
do you see the connection between what you're doing in the first line and what needs to be done in the second line?
no man im just fucking stupid
so, you're getting the user's radius by calling
Convert.ToInt32(textbox1.Text)
do you see another place you could put that chunk of code to make this work?
hint: that place is the part that has an error right nowwait. so
wait i need to convert the radius and double the parentheses
?
i have no idea what that means
you're already converting the user input radius to an integer, that's what that part i quoted does
hold on
im just stumped man
i know its something so fucking easy and its pissing me off so bad
do you agree with me that the expression
Convert.ToInt32(textbox1.Text)
gives you the user's radius as a number?yes
and
new Circle(...)
needs to be passed a radius as a number?so would i do like
Circle c = new Circle(Convert.ToInt32(Radius));
?
try it
you changed something you shouldn't have, and i'll ask you to explain why you changed it once you find out it doesn't work
Radius
is not a variable that exists here
but you know how to get the radius, right?do i declare a new variable
no
let's go back
do you agree with me that the expression Convert.ToInt32(textbox1.Text)
gives you the user's radius as a number?
im following
so why did you change it?
why did i convert it?
no, why did you change
textbox1.Text
to Radius
i didnt
you didn't?
no i put that in the line below it
but you're missing what it means
what does
Convert.ToInt32(textbox1.Text)
do in plain english?it converts the radius into integers so the textbox can read it
or circle
right, and where is it getting this thing that it's converting to an integer?
from circle
no
there is no
Circle
in that snippet of code
you can't have a circle yet, because to make a circle you need a radius firstsoooooooooooooooooooooooooooooooooooooooooooo-p[
i Q31neg4=wf9-
what is
textbox1
?its the user input
so when you write
Convert.ToInt32(textbox1.Text)
, what is it doing?its converting that number to an integer
the input
yes, the user input
it's converting the user input to a number
okay
so what does that tell me
and to make a circle, you need a number
and since you want the number from the user input, how would you do that?
send it back to the circle class
i thought i was already doing that
you were 99.9% of the way there but changed something for no reason
based on what we just talked about, what is the problem with
Circle c = new Circle(Convert.ToInt32(Radius));
?
you just have to change one thing and it will workdoes it need to be converted to something else
what are you converting?
a number
no, you're converting something to a number
so where are you getting that thing from?
it's the user input, right?
is the user input in
Radius
or is it in something else?dude i am trying everything i dont fucking know
i dont
do you know where the text your user typed their number into is?
the textbox
yes
Circle c = new Circle(Convert.ToInt32(textBox1.Text));
yes
this doesnt work
it should
do i need to replace the OTHER one with radius?
the other one is irrelevant
it doesn't actually do anything useful here
it's a side effect of making things static and not understanding why
this works
run it
well, fix the typo and run it
it doesnt work
the program is crashing again
well, it's progress
"it doesn't work" is once again not specific
is there an exception this time?
yes actually
🙂
MORE progress
so it does work, you just have a new problem
welcome to programming
a different error is always better than the same error
the get line
ah
it's the same problem you had with the set line
right
the getter is calling the getter
infinite recursive loop
you're saying that you want to get the value of Radius from Radius from Radius from Radius...
FINALLY
YES
IT
WORKS
HOLY FUCK
I CAN SLEEP
THANK YOU
a word of advice
maybe all of this is being exasperated by you being tired
that ABSOLUTELY is a thing, especially in software design
but it seems to me that there's a good chance you're going to fail this exam and/or class
that does not mean you are a failure
or you are stupid
or anything of the sort
the goal of an exam is to assess how well you have learned the subject material
i already passed the class
i have an 89 in it and i havent needed this level of help until now
even if i flunked the exam i wouldnt fail
my brain is just tired dude
possibly
but I think there's a level of proficiency that you're still lacking, tiredness or no
ive been staring at graphs for the last month working on mocap animations and writing 2000 word essays for my history class
maybe that's enough to get by in this class
im just burnt out man
my brain is out of juice
and that's fine
my HONEST advice should have been "fuck it, go to bed and take care of yourself" hours ago
you lost the fight on this particular assignment a while ago
and even if it meant a failure in the class, a couple hours of us tutoring you isn't going to make that difference
if you're just taking this class for a credit or whatever, godspeed
i dont really get what youre saying
i have a good grade in the class
and i have never asked anyone for help like this
grade != understanding, that's the most valuable thing i got out of college 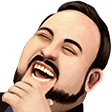
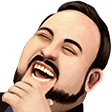
yeah, basically
at 2 months of taking a class you're PAYING for, I would expect you to be a lot more proficient in basic shit than this
i don't know what the class was supposed to teach you but these are pretty introductory concepts to be struggling so much with (could just be from being tired)
like, what are parameters, what is a method, what is the difference between static and non-static
lord knows I had shit classes in college
if you guys dont think i can do it then thats your own thoughts. i want to make video games, programming is part of it. ive struggled with it more than others but after really putting my brain too it for a while i will figure it out. i came for help because, again, i am absolutely burned out on other classes and i just came to a blank. and google was no help
100% not what we're saying
my point is you seem to have stressed the ever living hell out of yourself tonight
over one damn assignment
that ultimately means very little
dude its because of my dad lol
i dont want to disappoint him
but i was so burned out because of other classes today
i just hit a wall with it
after really putting my brain too it for a while i will figure it outthis will only get you so far, and it's a poor use of energy anyway collaboration and learning from others is KEY to basically any creative or technical field
especially with programming there's a point where slamming your head into the wall isn't worth it and you just have to take a break and rest
i do it
okay. i appreciate the advice. i really, truly, honestly, need to sleep
and there's a skill ceiling to how good you can get on your own
and that ceiling is rather low
thank you guys for your help
seriously
come back
im sorry i caught an attitude to start
I appreciate that you took it to heart
many don't
shit is just frustrating
yes, it is
@fixed(void* x = &Jimmacle) @ReactiveVeina thanks again for your help and sorry for catching the attitude
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.