✅ Which of these for-loops is more performant? How can I improve
Not sure if I'm thinking about this correctly or not. Would love to be pointed to resources regarding optimization if you have them. ~skr
23 Replies
benchmark them and find out?
GitHub
GitHub - dotnet/BenchmarkDotNet: Powerful .NET library for benchmar...
Powerful .NET library for benchmarking. Contribute to dotnet/BenchmarkDotNet development by creating an account on GitHub.
I will try that later yes
just trying to understand from a theoretical standpoint rn
i.e.
System.Buffer.BlockCopy
versus multiple array indexes in loop
also, it's not represented in my examples, but is previous element value cached with compiler optimization
/ can I output the IL code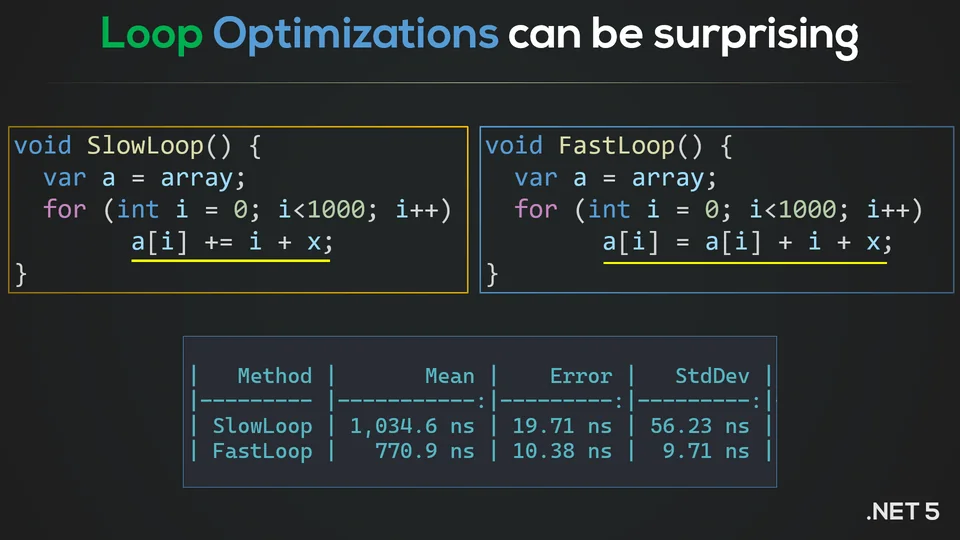
the first is marginally faster, at least on my machine
the reason why is fairly simple, the second version does more work
this is my final submission:
i suppose copying is more expensive than having multiple indexes
i loop hoist myself instead of relying on the compiler to do it
thx all
Don't forget spans
Honestly if you really want to prematurely optimize, you can even go as far as making your decoded array uninitialized as long as you can guarantee you are setting all elements before using any of them
Might score you some nanoseconds lol
but then also
Unsafe.Add
and Unsafe.IsAddressLessThan
and get rid of bound checks ;pthere's this thing called restraint
practice it
100% this
but then it depends on the size again iirc, as u would have to pin it or have it on the stack
i thought of going that way and vectorization for my raytracer, but then i simply threw it on the graphics card via opencl xD
Honestly just throw it on the stack like you said
You’re right
that depends on the actual context, the stack is after all more limited than the heap
there are alternatives after all, simply pre-pinned buffers or unmanaged memory allocation to throw the data into while "generating" it
no? you don't need to pin to have a ref
that's the entire point of having a ref instead of a pointer
but its about having 2 refs and comparing them
hmmm, wait, i think i dont know enough about that
Unsafe.AreEqual?
or Unsafe.IsAddrLessThan
its about using these methods
both are fine without pinning
thats, again, the point of using references instead of pointers for this
if you had to pin, you could just use a pointer instead
ah okay, good to know ;p
never had such hot paths where it was an issue 😂
(it pretty much never is)
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.