โ how to supress warning CS8774
The following code throws a warning, how can i suppress it? Even an ! on the end doesn't affect that warning
i want users of this function to know that they have a non-null Potato once M has completed.
I know that an 'await' returns a task and that during the execution of the task the potato might be null, but that is standard reasoning for an async function - if i call an async anything, the results might not be there until the Task Completes ...
https://sharplab.io/#v2:EYLgtghglgdgPgAQEwEYCwAoBAGABAlAOgBEoIBzGAewGcAXKAYxsIGEqATAUwEEYIANgE8aUGgG5MOfCgCskjFIDM+JLla4A3ply7cOvQHlgAKy6M6AflwB9AA5U6EOlQV79GdwG0AslzDAXABOAHKOIQCuAgIAFPxgXFQAZjH2js5UAJSZALoGuggqCAAc+ABsuD4xmVr57rYOTi4AvPgAnLgAYlx0jAAWAArpLtUAhOK4APSTAO4QQTCw5OoAysUA7OsALCCV/oFBuADkaU1UR7hgEfS4fRAAbly4ELjUMAC0MFECuPeCEU8Zn0uDBcFwAB5QBgwciEOoAXzqdUK+FKCDKAB5jGYLAA+Lo9fpDM7VLQIdavLgzXDY8x0ariREYeFAA===
SharpLab
C#/VB/F# compiler playground.
8 Replies
you probably need to wrap the call
without that, the
!
notation is applying to the Task
being await
ed, not the await
ed result@JakenVeina
adding brackets doesn't fix it ๐ฆ
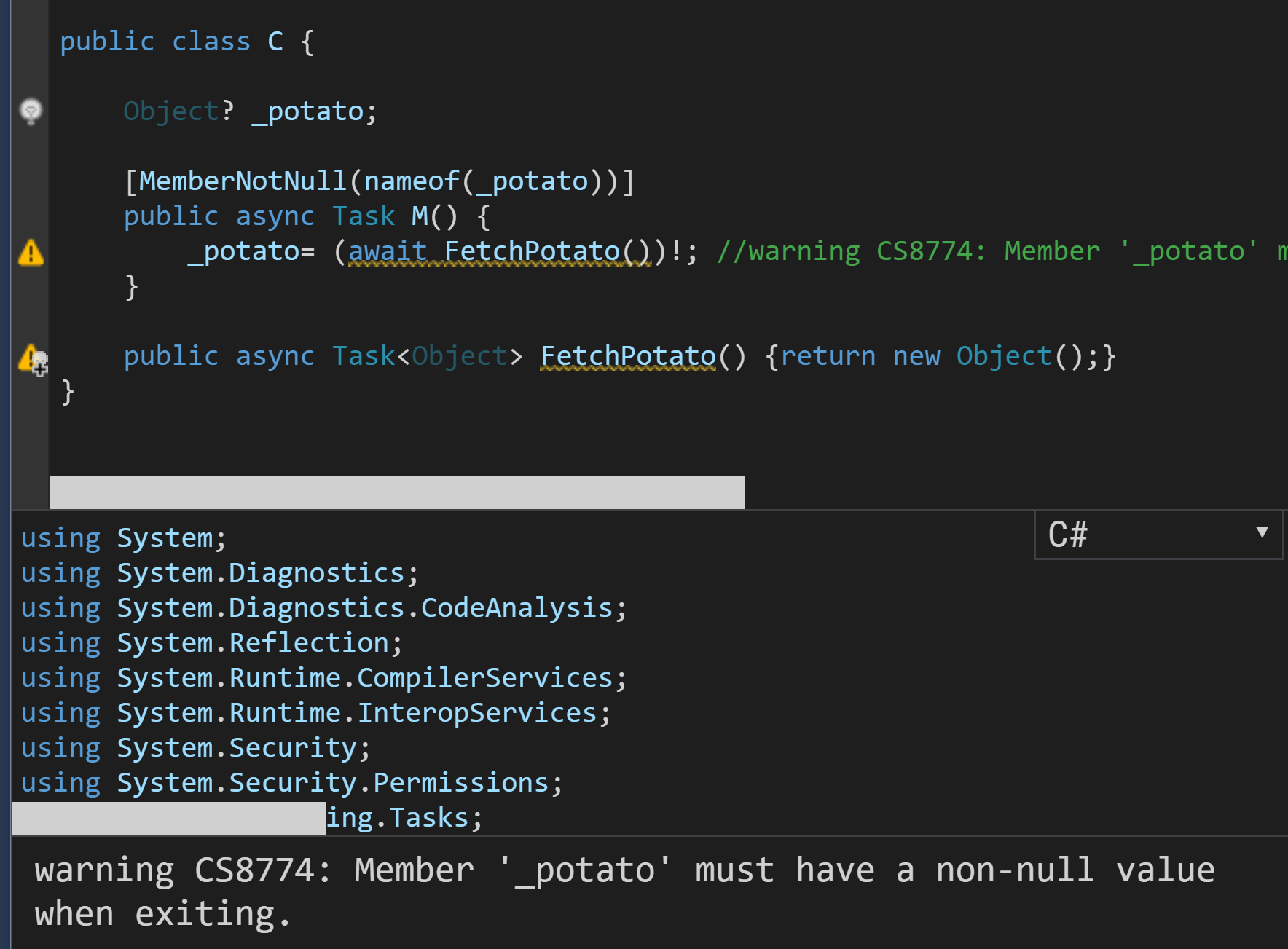
the issue is this is valid
huh?
how is that the same scenario?
ohhhh, right
as in, that's the caller's scenario
[MemberNotNull]
is not interpreted async-lynot the pit of success ๐ฆ
eg; if i checked the Result property of an Task that is not completed, i'm in undefined behavior; of course this could be NRE - i wouldn't expect invariants of the function to be enforced while the Task is incomplete ...
Question still stands then - any way to suppress the warning, or should i just #pragma around it?
what you would be looking for is something like
[MemberNotNull]
except async-aware
which doesn't exist
so, no
if you want to suppress the warnings and risk consumers being able to fatally misuse the API, go for itThere have been some proposals brought up before
https://github.com/dotnet/csharplang/discussions/5657
https://github.com/dotnet/csharplang/issues/6888
Not sure how far it's gotten since then though
GitHub
API Proposal: MemberNotNullWhenCompleteAttribute ยท dotnet csharplan...
Background and Motivation MemberNotNullAttribute indicate that when the method return, the given members are not null. This is perfect when your Initialisation code is fully synchronous. But when u...
GitHub
Require await to apply nullable postconditions to task-returning ca...
Related discussion: #5657 Summary Assume that nullable postcondition attributes such as MemberNotNull only apply when Task-returning calls are directly awaited. Adjust analysis of implementations t...
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.