❔ Maximum Subarray Leetcode Help
I'm getting the wrong answer with test case
Im not sure what I should be doing differently but I think I may be misunderstanding something with Kadane's Algorithm and negative numbers
11 Replies
I have exceptionally vague memories of Kadane's algo
should do the trick though
max should be 6 if I'm reading the array right
4, -1, 2, 1
that code should produce that result
Doombox#1389
REPL Result: Success
Console Output
Compile: 572.059ms | Execution: 67.100ms | React with ❌ to remove this embed.
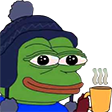
can you explain a bit about why you reset currSum to 0 if it's negative?
uhh, good question, did the algorithm actually specify that the sum should be non-negative?
might just be inventing that one
oh wait, no, the sum should always start at 0 of course
I doubted myself
I should really just google it 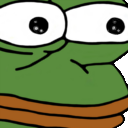
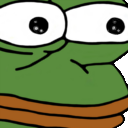
what do you mean it should always start at 0?
the sum of the numbers should always be calculated starting from 0, otherwise it's not much of a sum
as an optimisation, if the sum is ever lower than 0, we can safely assume that we need to start calculating the next subarray
ooh I see
and by doing that, it resets currentMax to be equal to the current Array[i] value in the next iteration of the for loop
kind of starting the sum over from there?
yes, essentially
imagine an array like this
-4, -5, -3, -1, -10, -3, -1
, the max value would always be the smallest single number (-1
in this case), easier to understand the code when you step through it with an array in mind
it's a simple enough bit of code that you can essentially run the algorithm in your head, or on a piece of paper at the least
https://www.geeksforgeeks.org/csharp-program-for-largest-sum-contiguous-subarray/ a better explanation than I can manage, even walks through an examplethank you!
I def understand it a lot better now
so
Here's what I have now:
But Im getting an issue when the array is [-2, -1]
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.