25 Replies
First i tried doing this without Identity but it seems like the Context.UserIdentifier will always be null
Im just reading and trial and error as im going
enter user Id first then you want to send to a specific user, so you use their UserId and enter a message
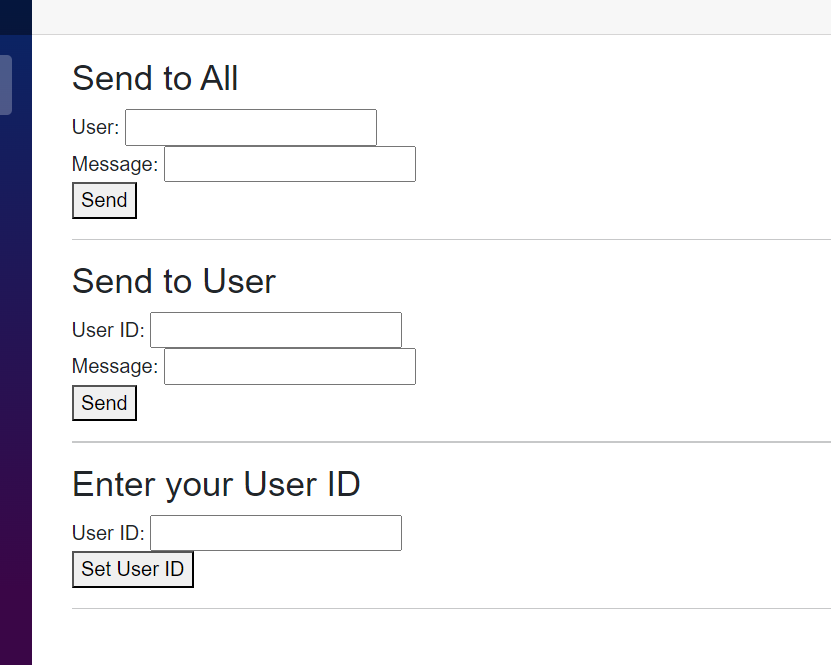
The Context.User.Identifier is meant to automatically set based on the authentication
But it seems like it isnt being set here
Can anyone help out/
im using the user.id in the code above instead of the Context.User.Identifier because it returns me null
what is
Context.User.Identifier
?returns the unique identifier for the current user associated with the connection
Authentication and authorization in ASP.NET Core SignalR
Learn how to use authentication and authorization in ASP.NET Core SignalR.
let's see your auth config, then
and i do call the authentication on use
okay
do u see the problem>?
no
oh, wait
you did post your config
let's see
CustomUserIdProvider
well i just checked the UserIdclaim seems to be null
howcomes its null tho
what other claims are there?
i believe thats the only one
well, don't believe
find out
it is the only one
would i have to put all the other claims on false if im not using them? could that be why?
so, wait
is it there or not?
if it's there, what's the problem?
that claim is the only one there
the problem im facing is that idprovider should assign the Context.UserIdentifier a value if its not null
but it is null
okay, so
again
if the claim is there
then what's wrong?
if
Context.UserIdentifier
is null
, I have to assume you're not correctly configuring SignalR to use your CustomerUserIdProvider
except, then how are you able to confirm what claims are present?
show me the ClaimsPrincipal
this too confusing
what is?
are u using HttpContext directly in the component?
the identity stuff and how SIgnalR is meant to know from authentication and give it a value
could this be because everything is on the same page
like setting a id/username, and it first checks if the user is logged in?
indirectly through hubconnectioncontext
hmmm
no
this is because your auth system is not working
thus, I asked to see your ClaimsPrincipal
the one that led you to give a bunch of conflicting answers
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.