Fetch all models with field value of X
Sorry to ask such a basic question but I want to do the following....
I have a model called Work (and a WorkController and WorkRepository). On my Work module there is a field called "available" of type checkbox. Now i want to fetch all models with "available" selected.
1. What is best practice to implement a e.g. getAllAvailable() method? Is that on the WorkRepository, WorkController of Work model class?
2. How do I select all the models with that checkbox selected. In the laravel docs I saw this
$flights = Flight::where('destination', 'Paris')->get();
but I can't seem to use the where function on my model. E.g. Work::where is not working.
Thanks for any guidance11 Replies
Hi @undersound, a good practice is to create a scope on the model (Twill already use this feature to get published models) : https://laravel.com/docs/10.x/eloquent#local-scopes
For example, on your Work class, if you available attribute is a bool:
Then, you can get the available models like that:
For this 'available' need, you could also use the native 'published' feature of Twill
Laravel - The PHP Framework For Web Artisans
Laravel is a PHP web application framework with expressive, elegant syntax. We’ve already laid the foundation — freeing you to create without sweating the small things.
Thanks @agnonym , is this also applicable to laravel 9.x?
Since at least Laravel 4 🙂
Is the published feature of Twill you mention to indicate if a model instance is published? Because the available field on my model does not represent this state of the model instance. It is more a value to indicate if a piece of work is availble for sale
Sorry real beginner here with Laravel 🙂
Yep, just wanted to tell you it could be the same feature as you want to achieve with available.
y eah I thought that, appreciated
@agnonym that works like a charm, thank you very much! Out of curiousity... how would I implement the same functionality but than with the get function https://github.com/area17/twill/blob/3.x/src/Repositories/ModuleRepository.php#L55. Would I want to use the filter parameter there?
Not sure to understand what you want, is that for admin filtering like that:
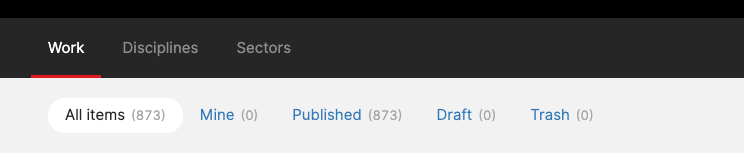
or is that for our frontend to be used in a controller ?
As far as I understand the WorkRepository class gets created when creating a module through
php artisan twill:make:module
. So in my case WorkRepository got created and extends ModuleRepository. I am using this class WorkRepository in my WorkController to fetch Work models for my frontend Route::get('/work', [WorkController::class , 'index']);
I think this WorkRepository class is also used by the admin views but I am not sure. Anyways, in this case I want to use it for filtering in the frontend.ok. Twill is headless CMS, all the files created when you create a module if for admin functionalities (Admin Controller, Repository, Request, ...), but it doesn't mean you can't use it for your frontend
Personally, I don't use the Repository for my frontend as if there are breaking changes in Twill package, I don't want to fix all my frontend
So, on my frontend controller I usually fetch models like that
$workList = Work::with(potential relations like medias, blocks, slugs, ...)->publishedInListings()->get() or ->paginate(x)
the publishedInListings is a scope that filter published models and checks if you have publication dates (start / end) that the model is visible
But I think you can use the get method of the ModuleRepository, something like that: app(WorkRepository::class)->get([relations], [scopes like 'publishedInListings', 'available'], [orders], 100)
If you want all your available and published models order by name attribute, and you need slugs to redirect to a Work page and your model has translations, medias and blocks, it should be (not tested)
app(WorkRepository::class)->get(['slugs', 'translations', 'medias', 'blocks'], ['publishedInListings', 'available'], [['name' => 'asc']], -1);
Thanks @agnonym , that's helpful and nice to see other approaches for getting content.