❔ Breaking an infinite while loop
I need help with stoping an infinite loop when the search word is found
36 Replies
put a boolean or something
or use LINQ Methods if you can
Ok! This is my project
It's an assignment consisted of Binary search
I wanna learn the bug
Couple of notes, if digitList doesn't have any items, no message will be printed out informing the user of whether something was found or not
Also assuming by
otherwise
you mean else
This code path will get executed even though it shouldn't
You also keep executing even after finding the number
You can just break;
you're doing your loop like this
on the ide you're using, they usually would tell you what's wrong with your loop and whatnot
while (first <= last)
this part --> if (first > last)
in your loop is useless
also, proly fix the formatting of your snippet above, kinda infuriating to stare at it 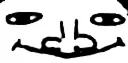
I forgot the paste the whole block
You're right. That message should be prompted too.
Well! There's this message that you mentioned, but I didn't show it from the beginning. This is the whole block btw.
break what? I tried it in multiple places but didn't see the right output.
break;
stops the loop. thats all it does.
so you just need to figure out when to call it.You're right. I need to make a condition that ends the loop when the searched element is found not just stoping the loop because we would like to, makes sense?
I mean when I use the break inside the else statement, it works well, it breaks the loop when found, but in the second screenshot when I input a wrong number, it needs to show a message that the element wasn't found instead of those copied numbers.
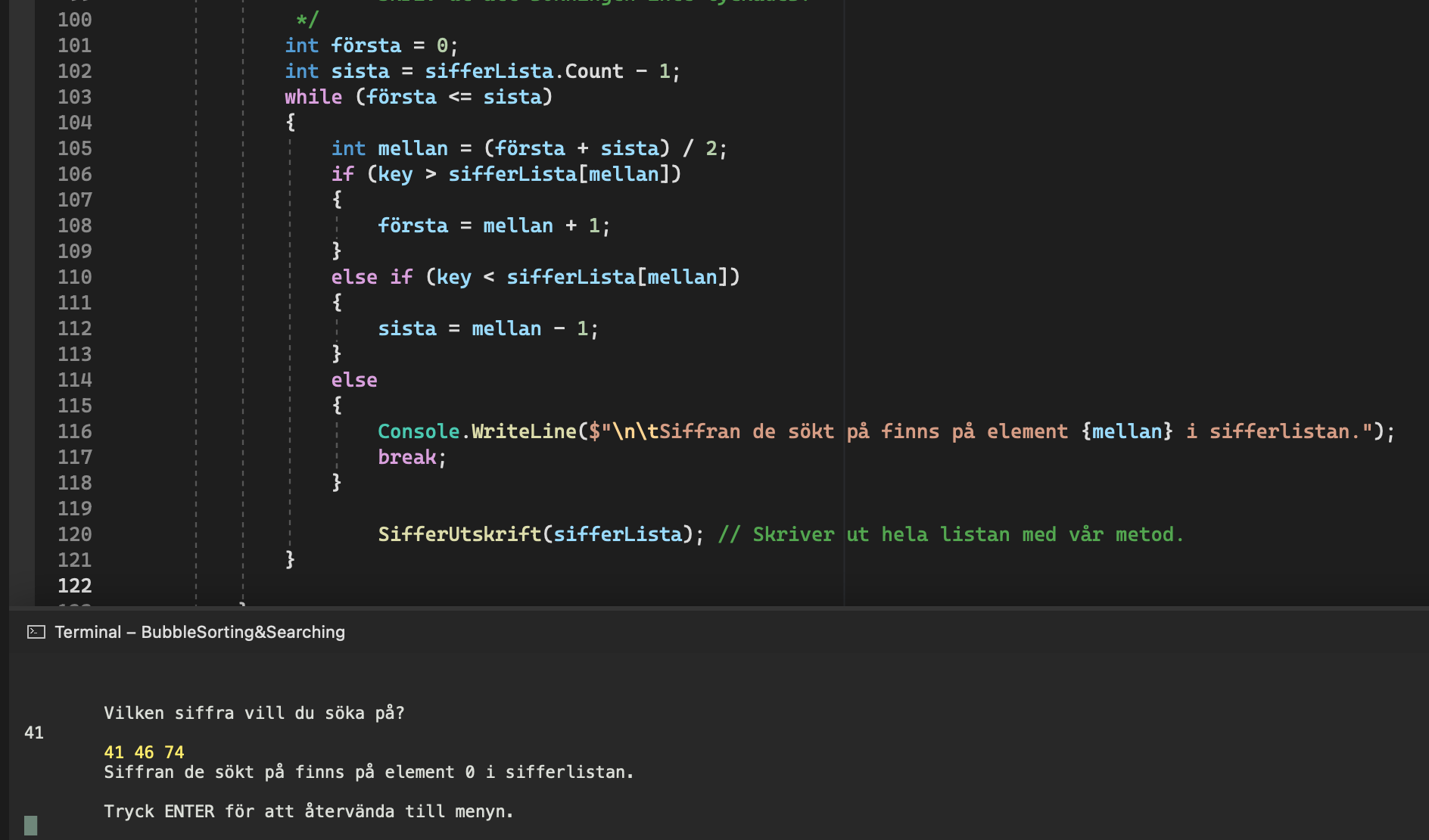
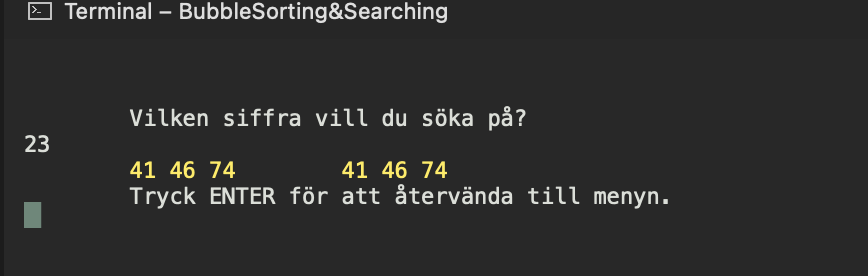
@Pobiegacould you please review the small change that I made?
@Faraj You need to keep track of whether you found the item or not
Ok! Yes, you're right. But does the break position is correct or not?
It's correct, but in the case you're tracking whether the item was found or not, you can just remove
break;
and instead have
while(first <= last && !found)
And set found
to true
instead of breakOk! Just like they did it here.
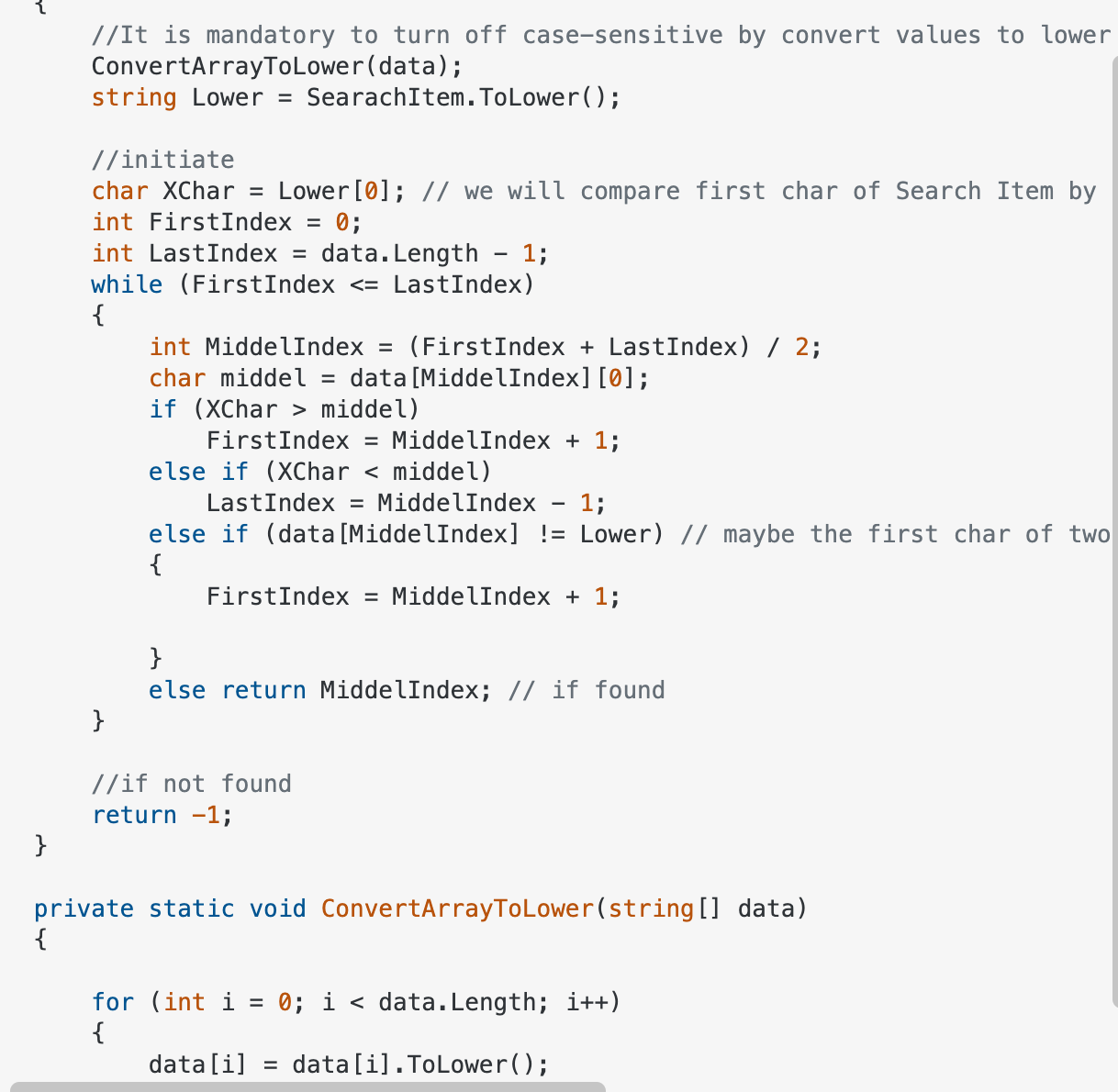
Here it's different
Because they return the index
You're awesome girl, you always show up to offer some help, I really appreciated.
Yeah! It's alphabetically algo.
I'm not entirely sure what do you mean by alphabetically algo, but my point was that they control code flow with the return statement, in your case you just print out a message to the user
So while you could do
return;
I think having found is more clear imosorry I meant in the first code in this link, they're doing almost the same https://stackoverflow.com/questions/26113161/binary-search-of-strings-in-an-array-in-c-sharp
Stack Overflow
Binary search of strings in an array in C#
Ok so I have been at this same error for about 18 hours and am totally lost. What I am trying to do is conduct a binary search where the search begins in the middle of the array and then eliminates...
The code in the link could just use a return statement instead
Ok! I meant a Binary search algorithm searching for string type elements through alphabetically.
You're right. Since I don't have any method defined, therefore I can't return a value if found or not found, so I would go with your approach instead.
Ok! Based on your knowledge.
so where should I set the found to true?
In the code path that checks if the item is matching
Though tbh, I would just refactor that method and make it return an index instead
When you think about a
Search
method you think about something that will return and index or something, you don't expect it to print the found index.
So instead you make the method return an index
And make the caller check whether the index was valid or not
If the returned index is -1, it's obviously invalid and nothing was foundOk! Your assumption is very correct and I noticed all the examples on Google they're using methods and returning either found or -1 I totally agree. But in this assignment I was given pseudocode and they want me to implement it in this approach.
as you can see they're mentioning any method or returning
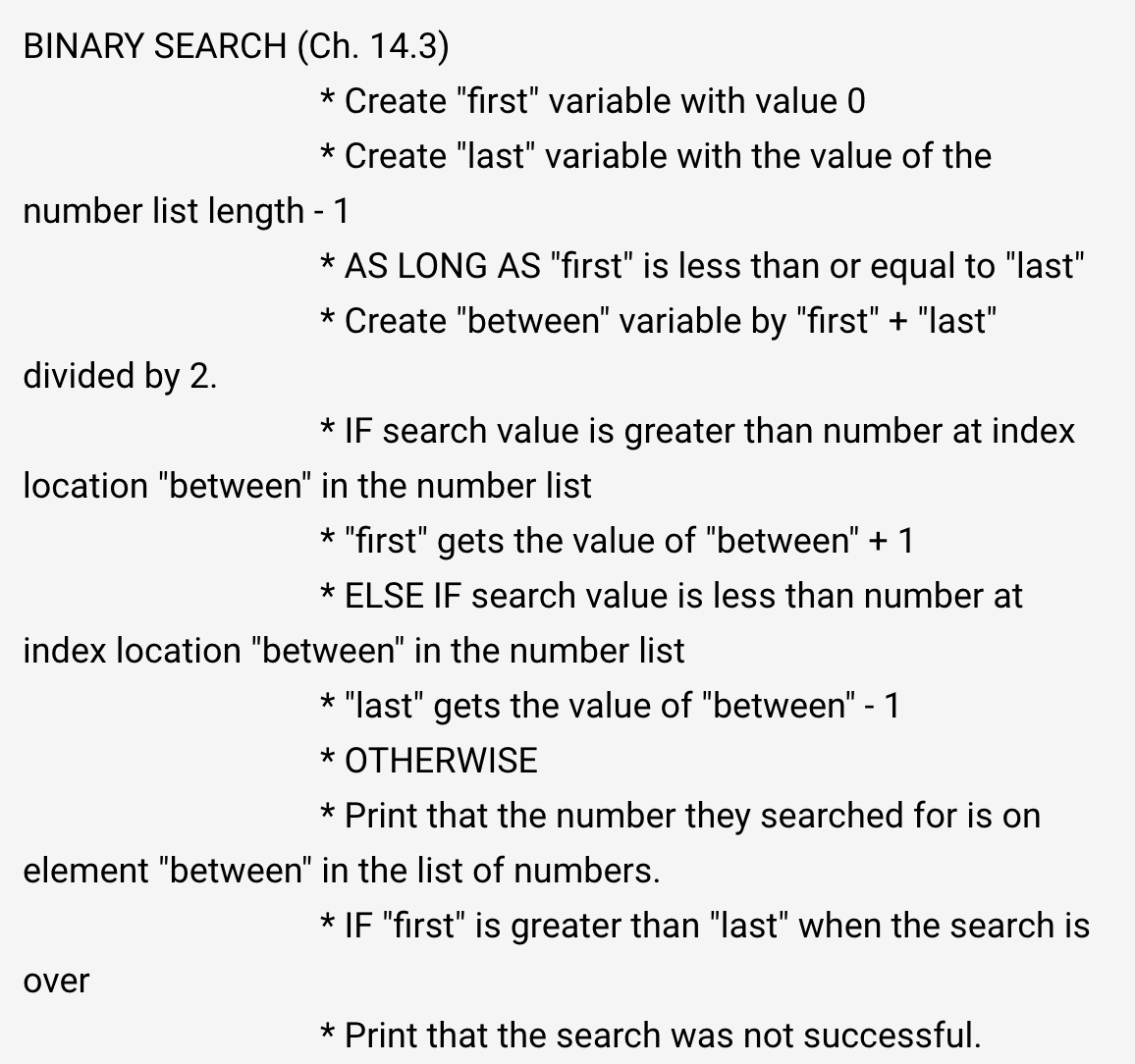
so what do you think?
should I ignore their approach and go with your approach by creating a method
I'm not entirely sure how strict the tutor would be 😅
So I guess keep your implementation
Ok! Yeah! I guess this is what I can do for now at least.
so where did you think the found variable should be located?
my friend
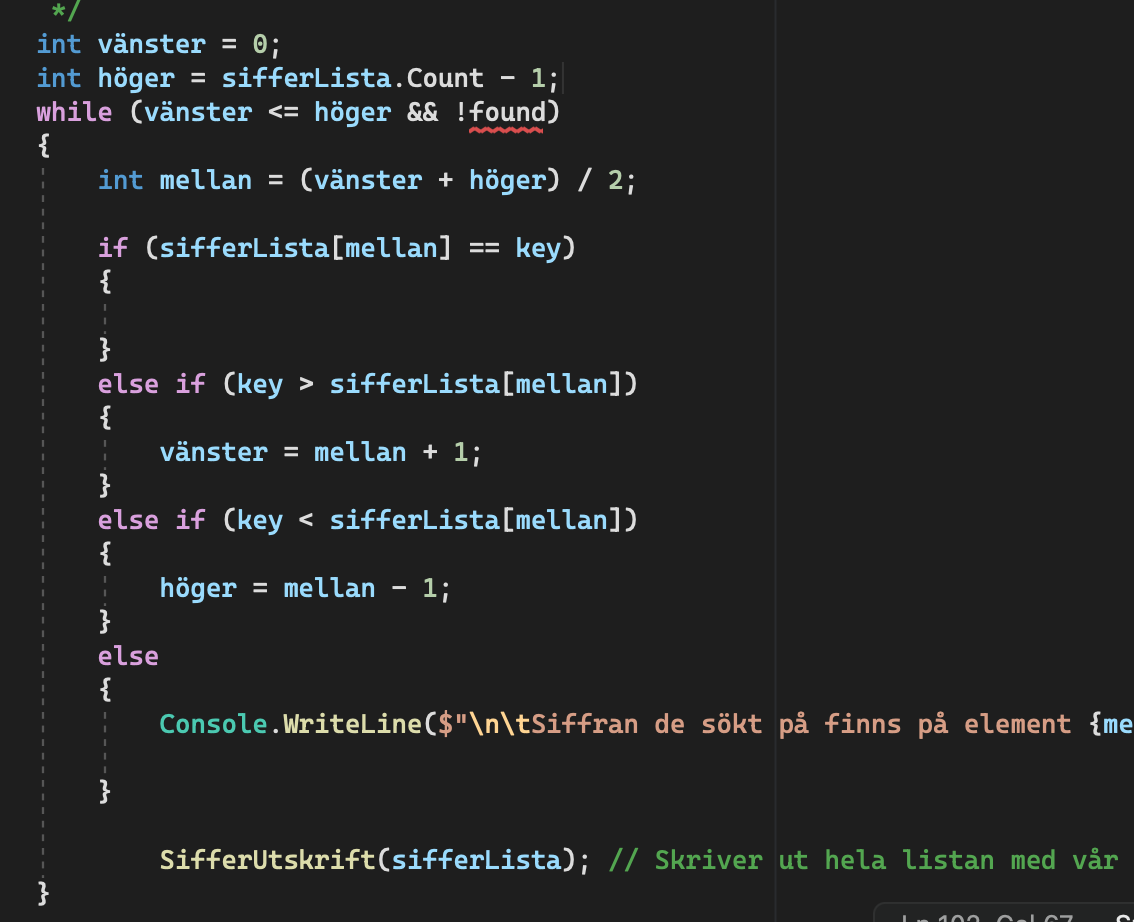
I mean in this approach
must be created outside the while loop so the condition can be checked.
Then updated inside
Ok! Just like when we do incrementing, we put it underneath the second brackets of the while loop. Thanks a lot
Awesome no error
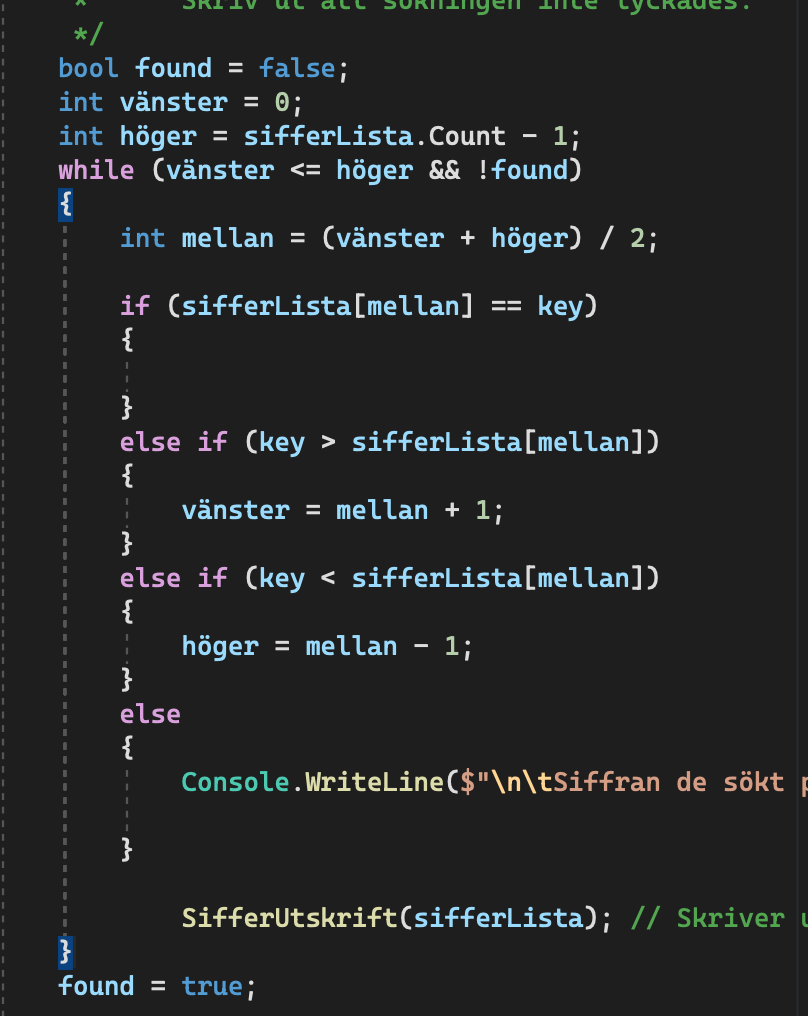
Let me test the code
It's not working actually
Yes, it'll not work, take a look at where you set 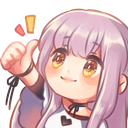
found
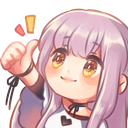
CancellationToken ftw!
Ok
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.