✅ TextBox problem.
Hello, I'm new to programming, I'm trying WinForms now. So I have a TextBox with 10 rows and 3 columns for a total of 30 TextBoxes. I don't know how to solve the problem that I don't always fill in all the boxes, and when I don't fill in any one box, it immediately crashes
19 Replies
so your code...
Very unclear exactly what you are asking for help with here.
You have 30 textboxes, and I'm guessing your code doesnt handle empty strings from them at all, causing the crash.
yes, does not handle empty strings
why 30 textboxes?
So you'll need to make your code handle empty strings then.
When I'm at home I'll try to post a code of how I'm doing
As an example, I want to make such a program, there is no problem with empty cells in excel, but in winform, empty fields are not allowed
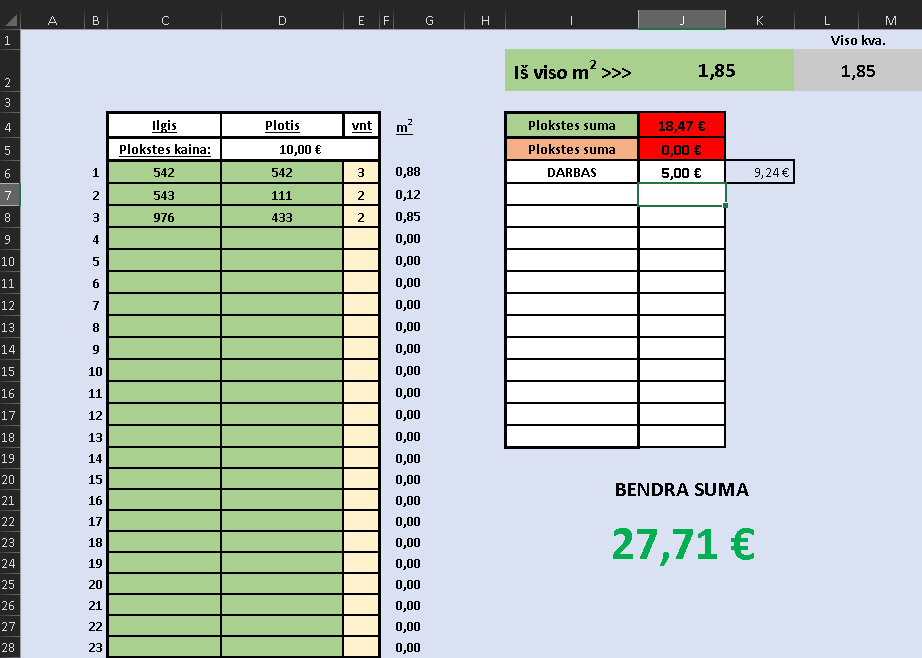
seems to me you are probably trying to parse numbers from empty strings
which will indeed crash
Also, as snowgirl probably wanted to point out, this type of program is probably not best built with 30 textboxes, rather a DataGridView?
I have a code 'int x1 = Convert.ToInt32(this.TextBox1.Text);
int y1 = Convert.ToInt32(this.TextBox2.Text);
int k1 = Convert.ToInt32(this.TextBox3.Text);
int x2 = Convert.ToInt32(this.TextBox4.Text);
int y2 = Convert.ToInt32(this.TextBox5.Text);
int k2 = Convert.ToInt32(this.TextBox6.Text);
int sum = (x1y1)k1;
suma.Text = sum.ToString();' I want empty fields x2, y2, k2, but the program does not allow me to ignore them
yeah
Convert.ToInt32(this.TextBox4.Text)
will crash if TextBox4.Text
is empty
I would recommend using int.TryParse
instead, and checking the return valueOk, I will try this option in the evening
How to upload the code here neatly?
$code or $paste
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/I created the code:
Is this a logical code? Because everything works, now i can empty fields
no no no
tryparse both parses AND tells you if it succeeded or not.
if you want a default value if it fails, I'd suggest making a helper method
now each of your assignments is much simpler:
Thank you very much, it is possible to know exactly why my code was not liked, although it works the same.
"works the same" isnt really true
you used tryparse but then ignored the parsed value
you also had to specify your default everywhere, which is just more noise
parsing a value twice is wasteful
Thanks for the help
if you feel that the thread is done, please
/close
it