How can i remove the parameter
I want to remove the parameter createdAt cause its of type date.
58 Replies
but to be honest what im really tryna do is solve this error
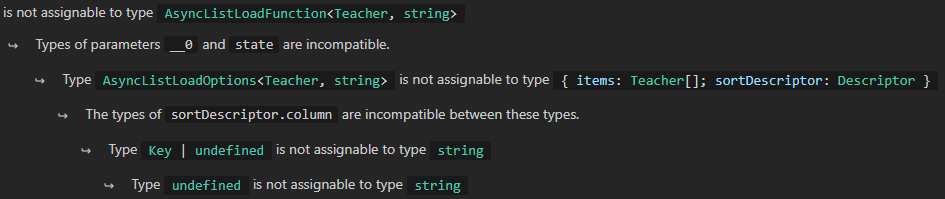
ok so i did
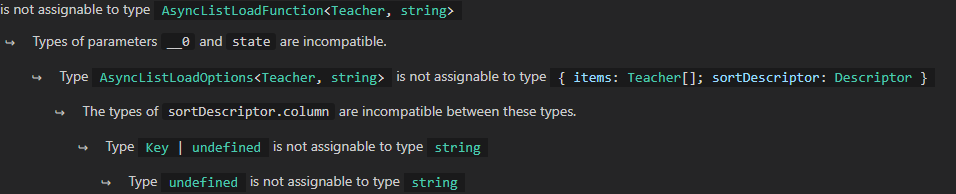
still getting this error
can i see how u queryed the data?
what's your prisma schema for teacher?
lemme see your getAll code
should i change it up to where it only returns name email role phone
okay usually i just do const teacher = api.teachers.getAll.useQuery().data
then just pass it into the function, the type should be Teacher[] when you do this
idk why u need to json parse the data
idk either kept throwing me errors
what kind
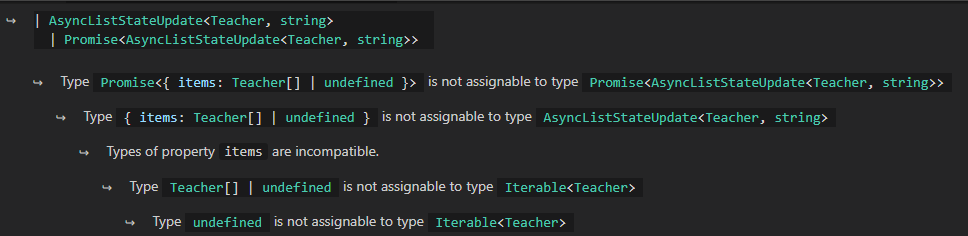
erm im abit confused tbh hahah do you mind we hop on a call and you can share screen me
i would but my upload is so ass that ill just lag like crazy
here ill send u a reference to my code
of how i derived to it
Table | NextUI - Beautiful, fast and modern React UI Library
Tables are used to display tabular data using rows and columns. hey allow users to quickly scan, sort, compare, and take action on large amounts of data.
so for now we just wanna ignore the return
oh damn im so stupid
ok i coulda just done
ouh lol does that work?
ya
no more error on load
not sure how to use await promise in this case to solve the eslint
i thought that was another problem hahah
š
i dont use nextUI that's where i got confused about your code
š
ah
i just used it to get something up and running quickly
alright so i think im done with the load function
ahh interesting, glad you solved your problem š :D
still didnt solve the problem š
LOL
now the sort function
what's the error
its what i sent earlier
.

your column type is wrong
is it
apparently you need to type it as Key | undefined
should be Key then?
wtf is type Key
that's what im asking myself LOL
probably sth from nextui
it isnt
or is it
maybe not?
got it
it was part of nextui
but i didnt find it in there docs
š
useAsyncList
Documentation for useAsyncList in the React Stately package.
it's in beta so docs might not be complete cos of all the changes
put await in?
like this?
im getting a error on this
tf
what's the error
š

wait it's for refreshing data?
basically, you refresh the page to load data?
well
not really
its a way to rerender
so the page doesnt actually refresh
but it re renders
when does it rerender?
when the list changes
adding new teacher or deleting
cant you just do a invalidate?
const CartDelete = api.user.postDeleteCartItem.useMutation(
{
onSuccess() {
void utils.user.getCart.invalidate()
}
}
)
const utils = api.useContext();
i did sth like this
<button
type="button"
onClick={() => { CartDelete.mutate(product.product.id) }}
className="-m-2 inline-flex p-2 text-gray-400 hover:text-gray-500"
>
<span className="sr-only">Remove</span>
<XMarkIcon className="h-5 w-5" aria-hidden="true" />
</button>
ok
wait
teachers.tsx (prisma shit)
ya just do a invalidate instead of refreshData
useContext | tRPC
useContext is a hook that gives you access to helpers that let you manage the cached data of the queries you execute via @trpc/react-query. These helpers are actually thin wrappers around @tanstack/react-query's queryClient methods. If you want more in-depth information about options and usage patterns for useContext helpers than what we provide...
perfect
worked
didnt know about that thanks
last error now
š
now this one makes no sense at all
alright
i fixed everything
im so happy
Glad to help :))