❔ Moq test exception thrown after adding and saved changes to the context object
Exception has occurred: CLR/Moq.MockException
Exception thrown: 'Moq.MockException' in Moq.dll: '
Expected invocation on the mock once, but was 0 times: m => m.Add(It.IsAny<User>())
Performed invocations:
Mock<DbSet<User>:1> (m):
DbSet<User>.ToString()
DbSet<User>.ToString()
DbSet<User>.ToString()'
at Moq.Mock.Verify(Mock mock, LambdaExpression expression, Times times, String failMessage)
at studyPalsTests.UserTests.CreateAccount() in c:\dev\c#\projects\410project\studyPalsTests\UserTests.cs:line 110
Exception has occurred: CLR/Moq.MockException
Exception thrown: 'Moq.MockException' in Moq.dll: '
Expected invocation on the mock once, but was 0 times: m => m.Add(It.IsAny<User>())
Performed invocations:
Mock<DbSet<User>:1> (m):
DbSet<User>.ToString()
DbSet<User>.ToString()
DbSet<User>.ToString()'
at Moq.Mock.Verify(Mock mock, LambdaExpression expression, Times times, String failMessage)
at studyPalsTests.UserTests.CreateAccount() in c:\dev\c#\projects\410project\studyPalsTests\UserTests.cs:line 110
c#
public void CreateAccount()
{
// arramge using mock for users
var mockSet = new Mock<DbSet<User>>();
var mockContext = new Mock<StudyAppContext>();
mockContext.Setup(m => m.Users).Returns(mockSet.Object);
var service = new UserManager(mockContext.Object);
// act
service.addUser(user);
// assert to check if the user was added
try
{
mockSet.Verify(m => m.Add(It.IsAny<User>()), Times.Once()); // exception is thrown here
mockContext.Verify(m => m.SaveChanges(), Times.Once());
}
catch (System.Exception)
{
throw;
}
}
c#
public void CreateAccount()
{
// arramge using mock for users
var mockSet = new Mock<DbSet<User>>();
var mockContext = new Mock<StudyAppContext>();
mockContext.Setup(m => m.Users).Returns(mockSet.Object);
var service = new UserManager(mockContext.Object);
// act
service.addUser(user);
// assert to check if the user was added
try
{
mockSet.Verify(m => m.Add(It.IsAny<User>()), Times.Once()); // exception is thrown here
mockContext.Verify(m => m.SaveChanges(), Times.Once());
}
catch (System.Exception)
{
throw;
}
}
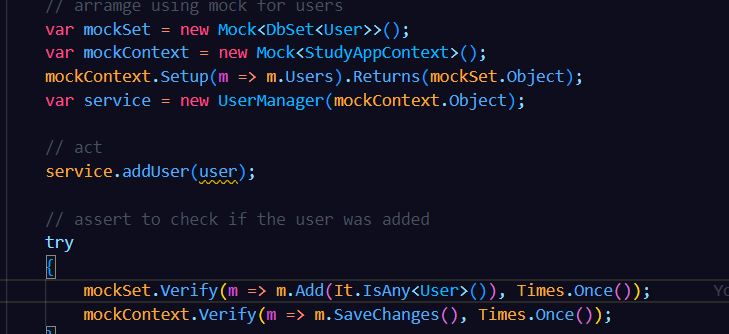
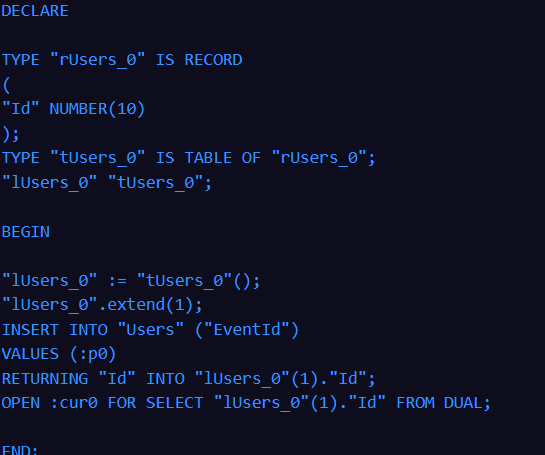
2 Replies
This is the adding method to the context object from the unit test
For context it is stating that there is no previous command for add called to save the changes, however I added the user. Wondering why it isn't working here...
c#
// no issues with this code?
public bool addUser(User user){
if(user == null){
throw new ArgumentNullException("Cannot have null values");
}
try
{
// check to see if there is a user with the same username in db
User user_check = _context.Users.Where(u => u.Id == user.Id).FirstOrDefault();
if (user_check != null)
{
throw new Exception("User already exists");
}
// will add the user to the database + save changes to commit them.
_context.Users.Add(user);
_context.SaveChanges(); // gets added succesfully
return true;
}
catch (Exception e)
{
throw new Exception($"Failed to add user to database {e}");
}
}
c#
// no issues with this code?
public bool addUser(User user){
if(user == null){
throw new ArgumentNullException("Cannot have null values");
}
try
{
// check to see if there is a user with the same username in db
User user_check = _context.Users.Where(u => u.Id == user.Id).FirstOrDefault();
if (user_check != null)
{
throw new Exception("User already exists");
}
// will add the user to the database + save changes to commit them.
_context.Users.Add(user);
_context.SaveChanges(); // gets added succesfully
return true;
}
catch (Exception e)
{
throw new Exception($"Failed to add user to database {e}");
}
}
Looks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.