[SOLVED] Storing pointers in an array instead of the objects themselves?
Id like to store some objects in an array like this. I know that the array will contain a lot of empty spots so I would like to store pointers instead of reserving space for the entire object. Sadly this does not seem to be possible because the object is a managed object.
I don't think that storing the data in an array like this is the most optimal way, but seeing that I need the data in a 2D array like structure this is the most performant thing I could come up with.
I was also thinking about storing an array of dictionaries to get rid of the empty spots, only that would increase lookup time.
If anyone has a better alternative to this please let me know ^_^
(The reason why the array contains a lot of empty spots is because the lookup index matters, things in the x direction are of the same type, and things in the y direction are related to eachother)

21 Replies
(Please ping me on reply, that way I can get back to you the quickest)
Nice red squiggly lines
That tells so 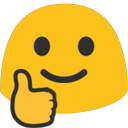
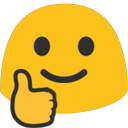
I mentioned this in the post already but the error is due to the object being a managed type

classes in c# are reference types
which means theyre always allocated on the heap
so when you do
new SomeClass()
a reference, aka a pointer, is returnedOh that makes sense
structs on the other hand are allocated inline
so
RuntimeObject[]
is an array of pointers/referencesGood to know ^_^
Thanks
Would you know a better alternative to solve this problem? Since i feel like allocating an array with a lot of nullpointers would be a waste of memory
how big would the array be?
Im trying to store a game scene in a certain way
Object | Object | Object | Object |
__
XYZ | XYZ | XYZ | XYZ |
_
CompA | null | null | CompA|
and are you only allocating it once?
It doesn't have to be an array
isnt a game scene just a collection of entities and their components?
It is, only im trying to store in a way where i can lookup components in an array while also beeing able to loop through all components of the same type in a very effective way (by having all components of the same type stored next to eachtoher in memory)
you could create an array for each component type, and then an array or dictionary that contains those array
Yea, I think that nesting dictionaries in the array would be the best option
But thanks for thinking with me ^_^
nesting dictionaries?
why?
sorry I worded that wrong
I ment putting a dictionary in a list
oh
well you only need one
unless i misunderstood
Jup, the way im currently looking at it i might need none
im currently mainly looking on having a 2D list stored in ram like this
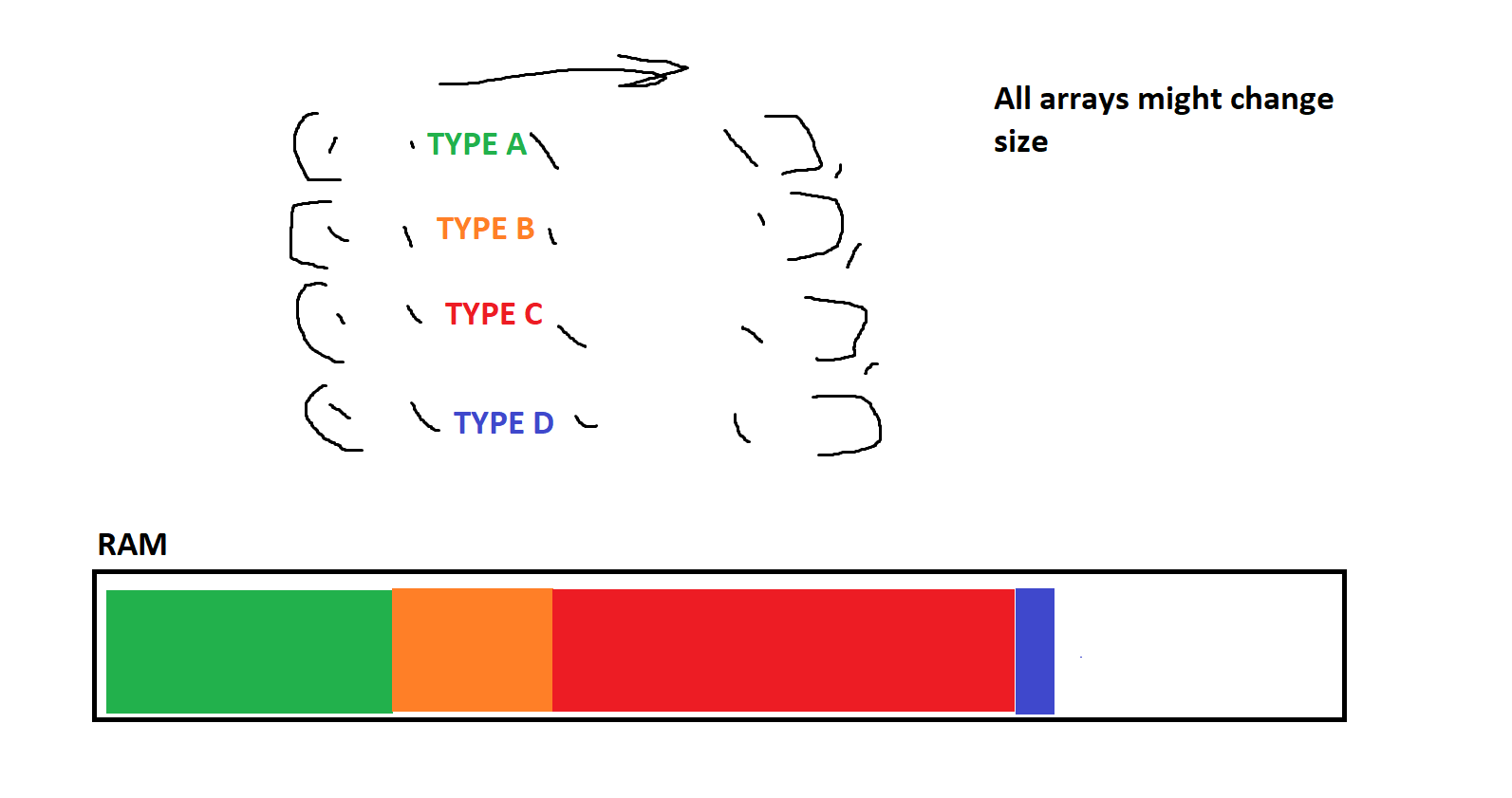
im just not sure how to achieve it
Since the lists might resize
im not sure how it will work with that
Since i dont want to reallocate everything when one of them changes
(So for example, in this case the green can be the objects that keep track of where the components are, while the orange part are all the transforms and the red part is some other component that needs more memory and the blue one the same as red but with less mem usage)
This way when i loop over either all the game objects or all the transforms, or the transforms i can have the best cache consistency
Now that im looking at it, i dont have to store them all in one variable
That would simplify it by a lot
Ill figure something out