How to SSG a page from my T3 app??
I have been following this documentation:
https://trpc.io/docs/nextjs/ssg
So I have a
[chat].tsx
route and I put this in the page component:
But, ctx is giving me ts errors.
And when I do ctx: {}
I get this
Not sure why this error is not addressed in the docs.Static Site Generation | tRPC
Reference project//github.com/trpc/examples-next-prisma-todomvc
30 Replies
your trpc handler needs context that might contain prisma, session data, etc
if you used create t3 app, we have a
createInnerTRPCContext
function that you can export and call
this will generate context without needing req and res, which you don't have in SSGwhere do I call it?
ctx: createInnerTRPCContext({ session: null })
exact syntax will differ depending on your packages etcI can't find it in the t3 doc
where is it imported from
trpc.ts
Server-Side Helpers | tRPC
createServerSideHelpers provides you with a set of helper functions that you can use to prefetch queries on the server. This is useful for SSG, but also for SSR if you opt not to use ssr: true.
read through this article
our docs aren't meant to teach how trpc works, thats what the trpc docs are for
oww
there
createContext
is awaitedif the function that generates your context returns a promise, you need to await it
if not then not
yeh i know but why createContext
instead of
createTRPCContext
createContext
does not exist in my code.
What a hassle to implement SSG in trpc
so, I created this file context.ts
in server folder:
Then I import :
then use it like this????
That's what the docs say
But it expects argumentsthe name of the functions is arbitrary. there's no magic going on here.
it requires arguments because it's a function that has typed arguments:
export async function createContextInner(opts: CreateInnerContextOptions)
the type of the args is a few lines up, or you can have intellisense tell you what it needs
in your case it's a nullable session. there's no session in ssg, so you make it nullI'll try that
i use the serverside helper but after generating it a few page it show and error, something like this any ideas? sorry for out of topic
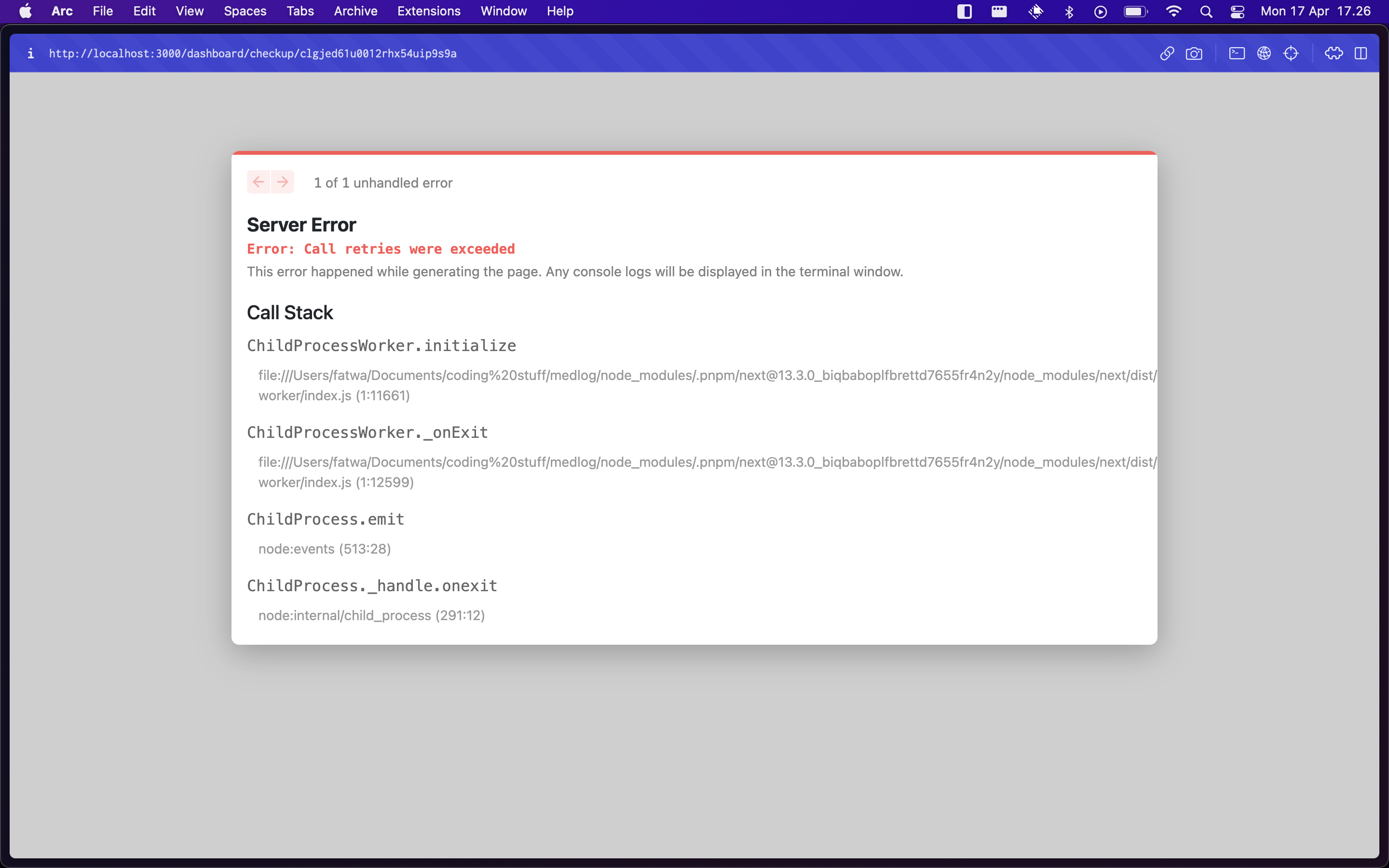
ghmm, I dunno this is the first time I see an error like that.
Try creating your own post in questions.
This is still hella confusin
its just typescript
has nothing to do with trpc
you cant call a function with incorrect arguments
if you define
function someFunc({ foo: number }) {}
and then try to call someFunc()
without args you'll get an error
thats all it isThat's what it is yes
but it's still complaining
GitHub
examples-next-prisma-todomvc/[filter].tsx at main Β· trpc/examples-n...
mirror of https://github.com/trpc/trpc/tree/main/examples/next-prisma-todomvc - examples-next-prisma-todomvc/[filter].tsx at main Β· trpc/examples-next-prisma-todomvc
here it's being done differntly
its being done in the exact same way in that example
look at what
ssgInit
doesyes
just
divided
into different files
my
createTRPCContext
was missing session
Honestly a pain to setup
thx
The whole reason for trying to implement ssg was to check if the chat is not found
and return {notFound: true}
Which I am struggling with atm
I had this in the trpc query
I then try to log the respone, it returns undefined eventhough I can still see the page with the chatData
ahh,May I Ask what data do you need statically generated? Seems odd for a chat
I want to return a 404 page if the chat was not found
Static Site Generation | tRPC
Reference project//github.com/trpc/examples-next-prisma-todomvc
following this guide
why can't I use the data that gets returned from trpc state??
the return statement of the
getStaticProps
I'd have to destructure like crazy tho . . .
read that page again
you get the data by using the query in the component
ok
but how would you generate 404 page?
why would you generate a 404 page in ssg
If the page is not found
if the chatId is invalid
Error pages don't need ssg, just render it normally without serversideprops or staticprops
yeh