Sending messages from a forum channel to database, but unable to grab the tags
Everything is going through to my database from Discord, but I cant figure out how to grab the tags and the tag IDs. I've tried a few things...with the help of our AI overlords...but still no luck. Below is the section of the code where I try to grab the tag id
client.on('messageCreate', async (message) => {
if (message.author.bot) return;
let appliedTags = [];
if (message.channel.type === "GUILD_FORUM") {
if (message.thread) {
appliedTags = message.channel.availableTags.filter(tag => message.thread.metadata.appliedTags.includes(tag.id));
} else {
console.log("No "thread" property.");
}
}
23 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.<ThreadChannel>.metadata
is not a thing.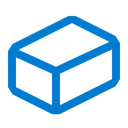
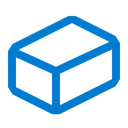
The forum channel is at
message.channel.parent
Thanks. I'm pretty new at this, but took a crack at updating the code. Still not working though. Does anything stand out?
if (message.channel.type === "GUILD_FORUM") {
if (message.channel.parent) {
const thread = message.channel.parent;
const availableTags = message.channel.parent.availableTags;
const appliedTagIDs = message.channel.appliedTags;
appliedTags = appliedTagIDs.map(tagID => {
const foundTag = availableTags.find(tag => tag.id === tagID);
return foundTag ? foundTag.name : null;
}).filter(tagName => tagName !== null);
console.log('Applied tags:', appliedTags);
} else {
console.log(
[WARNING] The message object does not have a "thread" property.);
}
}
If you're on v14 I think you need to use the ChannelType enum
Or the correct enum member
GuildForum
Is this instead of
channel
?Where you're checking the channel.type
I actually just deleted since I dont really need it. Is anything else sticking out as a problem?
// Getting new questions from Discord
client.on('messageCreate', async (message) => {
if (message.author.bot) return;
let appliedTags = [];
// Check if the message's channel is a GuildForum channel
if (message.channel.parent) {
const thread = message.channel.parent;
const availableTags = message.channel.parent.availableTags;
const appliedTagIDs = thread.appliedTags;
appliedTags = appliedTagIDs
.map((tagID) => {
const foundTag = availableTags.find((tag) => tag.id === tagID);
return foundTag ? foundTag.name : null;
})
.filter((tagName) => tagName !== null);
console.log('Applied tags:', appliedTags);
} else {
console.log(
[WARNING] The message object does not have a "thread" property.);
}
Tag suggestion for @manuelmaccou:
Instead of using method-based type guard functions, you can narrow channel types with the
.type
property
For future reference
ah ok thanks
No worries. Just looking
You have
thread
defined as the parent of the channel (the forum channel), so thread.appliedTags
is incorrect as ForumChannel
doesn't have an appliedTags
property
What you want to do is get the appliedTags
property of the ThreadChannel
Also, if you're running this every time a message is sent in the server, you will have issues. A regular text channel has a parent property, so it will likely throw an error when creating a map
What you had earlier to check if the message was in a ForumChannel would work, but you need to use the enums for v14
should be one of
I have another table that has approved channels. This code is only ran if the channel the message is sent in is one of those approved channels
Ok, well if any of those channels isn't a ThreadChannel with the
appliedTags
property, you will likely get errorsI see. So probably better to add it back in to be safe. Thanks
I would say so, yeah
Not gonna lie, I'm pretty lost
Regarding pulling in the tags
Show us what you got
I assigned
const thread
back to message.thread
hoping that would fix the problem you pointed out
if (message.channel.type === 11) {
if (message.thread) {
const thread = message.thread;
const availableTags = message.channel.parent.availableTags;
const appliedTagIDs = thread.appliedTags;
appliedTags = appliedTagIDs.map(tagID => {
const foundTag = availableTags.find(tag => tag.id === tagID);
return foundTag ? foundTag.name : null;
}).filter(tagName => tagName !== null);
console.log('Applied tags:', appliedTags);
}
Your if statement using
message.thread
won't work here, pretty sure that is for threads created from a message. You were better off using message.channel.parent
to see if it had a parent
For the thread
variable, you want to use message.channel
as this represents the ThreadChannel
which has the appliedTags
property
Let me know if that makes senseI swear....If I could I'd give you the biggest hug
Get me the manager....I need to tell them how amazing you are
Haha glad you got it sorted mate 👌🏽