NextAuth CredentialProvider with T3 default Auth Prisma models limitation question.
Actually I made a sign up page using CredentialProvider using Email ID and Password.
My question is,
1.) create t3 app with NextAuth has built in tables named Accounts, Session and VerificationToken. Now due to me using CredentialProvider the rest of the tables are not being used because NextAuth is limiting that intentionally. More on that here -> https://next-auth.js.org/providers/credentials
Now I want to know what functionalities I am missing, and do I even need those? Because my cookies are being created in the browser and useSession is also working fine.
2.) Also please tell me if I can delete the tables which are not being used in the prisma schema made by create t3 app.
Credentials | NextAuth.js
Overview
17 Replies
If anyone wants any kind of additional info please let me know. I found this solution https://github.com/nextauthjs/next-auth/discussions/4394 but I'd rather not deal with it if I can have the above questions answers. I'd be really happy if anyone could reply
GitHub
Database session + Credentials login · nextauthjs next-auth · Discu...
I am in need of help when it comes to logging in with credentails. i am trying to implement next-auth in my project and managed to make 3rd party auth (Google, Github) work. I implemented the Crede...
I guess ill be the only one lonely enough to answer my own post T_T
For anyone who is having the same issue as me, here is the answer. I dont know how I missed it. (Check the image)
Here is another great resource
https://stackoverflow.com/questions/43452896/authentication-jwt-usage-vs-session
Stack Overflow
Authentication: JWT usage vs session
What is the advantage of using JWTs over sessions in situations like authentication?
Is it used as a standalone approach or is it used in the session?
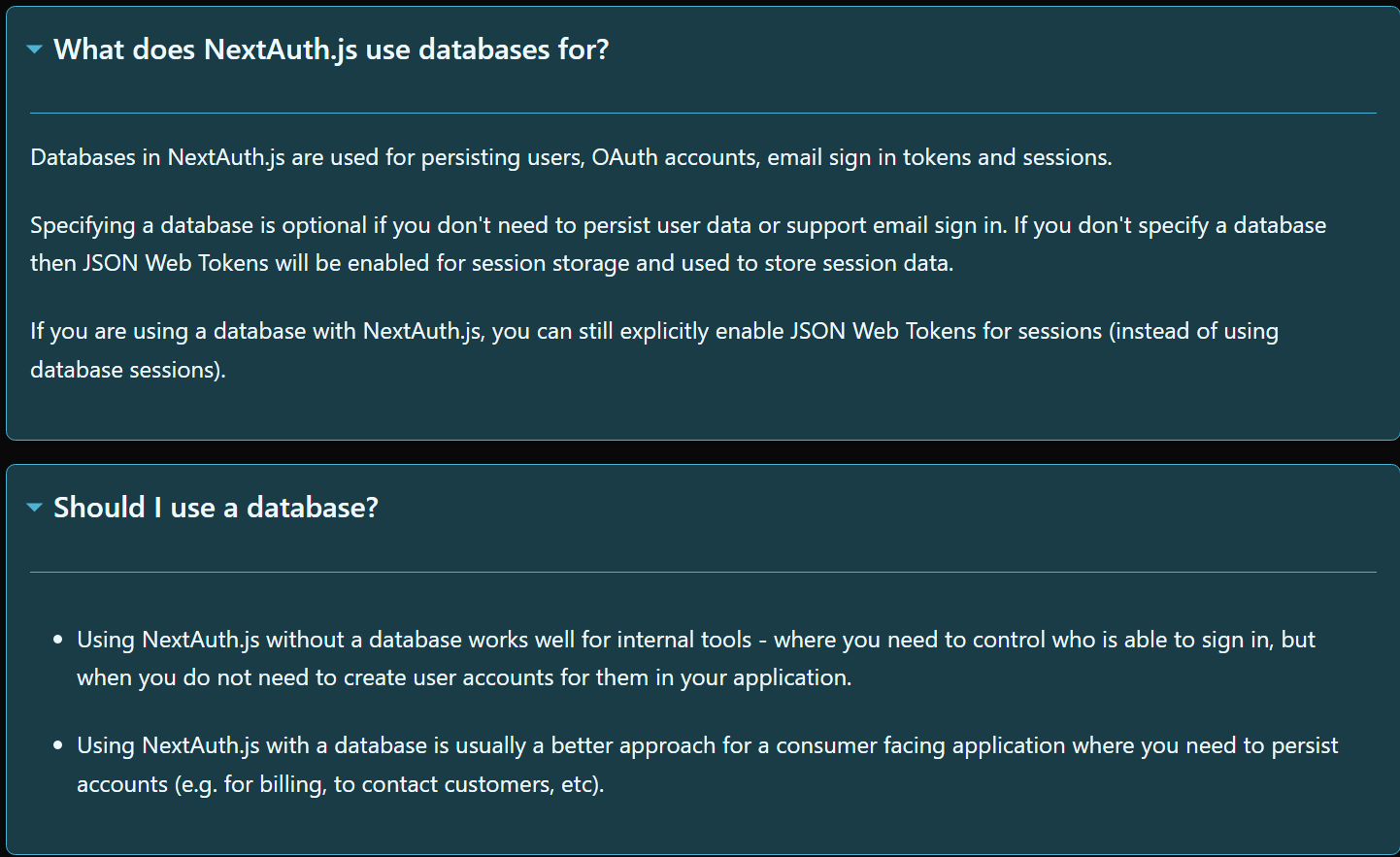
Did you get anywhere with this? I get that using the Credentials provider is a no support option but I really just want a basic username and password login, I don't get why it's so hard to do..
Yes I did it. Well basically I lost some functionality. Those functionality was saving user session data in the database. I don't mind losing that functionality so I am fine with it. I also dropped the three extra tables created when initializing the project with NextAuth as they were not being used due to me using credentials provider.
Here, to make your life easier I'll just give you the code
I had to split it up because of discord text limit. Just paste those two one by one and use prettier to auto indent.
If you find any line hard to understand let me know. I think the only thing that might not be obvious is the type checking. I am using zod for loginSchema to parseAsync to check for input credentials.
Oh also all of this is in auth.ts folder
Yeah this code looked familiar, I've tried this and useSession always returns null so I can't do anything with it
After some debugging the JWT and Session handler doesn't always get the user passed to it, and when it doesn't the Session handler encounters an error trying to read
id
from user
, since user
doesn't always get passed, it's sometimes undefined
causing the error, the bigger problem is that this is a 100% fail rate in my setup. Those handlers DO get the user, but only when it doesn't matter. They are both called twice during sign in, the first time they get the user
, everything is hunky dory, the second time they don't, then error, then no auth/user on the frontend
Flow I've noticed, Authorize -> JWT -> JWT (undefined user) -> Session (undefined user) -> Error -> JWT (undefined user) -> Session (undefined user) -> Error
Its weird, maybe you are doing something wrong in error handling because if you return null in the authOptions cred provider nothing should happen.
Did you try out my code? Because there im going into authorize, returning the whole user data and sending it to the JWT, there I am just using JWT token and setting token.id to the received user.id. That should work with no issues. You can try debugging from authOptions and see if you are returning user data containing the id in it. And then go to JWT and see if it is receiving the id so it can set session token id for the browser.
Yes I did try that code. I'm just going to switch to Lucia Auth instead, more boilerplate but at least I can have basic username&password without jumping through several loops and being told its not a good idea to use creds even though I don't see the harm in them..
If it is working as you want then more power to you :D
True, just didn't think I'd need to switch to a different auth library just to get what I want without the headache
Does it create session tokens and all too like in NextAuth?
Yeah they really want you to follow the passwordles sign in no matter what. Maybe I should have went with that instead. I wouldnt had to think about creating another functionality to reset password on forgetting
Yeah that's what I hate but oh well..
There's a password somewhere along the line, would've been easier if it was done locally
If by token you mean a cookie then yeah
It does support oauth too so if you still need discord or GitHub or whatever other service as a login
I see, thats better then x) I dont know the whole picture so I cant say for sure. I hope Theo doesnt get mad at me for that 😂
From my limited use, I'd argue against swapping for now, there's stuff I'd like Lucia to change before I'd say it can be a good alternative
Has Theo even mentioned credential logins at all ever?
Hmmm the videos that ive seen from him, I have mostly seen him use other methods. I can't be sure though as I have not seen all of his videos.
I'd say it would be nice to hear his thoughts on it but this thread isn't the place
https://github.com/ruhap/t3-credentials this works, can use as example
GitHub
GitHub - ruhap/t3-credentials: t3 stack with next-auth credential p...
t3 stack with next-auth credential provider. Contribute to ruhap/t3-credentials development by creating an account on GitHub.
I might try that later though it looks like the same setup as the code earlier in the thread, which didn't work for me 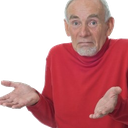
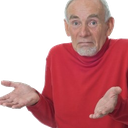