❔ Im learning refs and outs
i keep getting cs0177 errors if someone could take a look
public static void RemoveAllHeroes(string name, out List<Hero> sub)
{
List<Hero> _heroRem = new List<Hero>();
for (int i = 0; i < _heroes.Count; i++) { if (_heroes[i].Name.StartsWith(name, StringComparison.OrdinalIgnoreCase)) { _heroRem.Add(_heroes[i]); _heroes.RemoveAt(i); } } }
for (int i = 0; i < _heroes.Count; i++) { if (_heroes[i].Name.StartsWith(name, StringComparison.OrdinalIgnoreCase)) { _heroRem.Add(_heroes[i]); _heroes.RemoveAt(i); } } }
35 Replies
what does the error tell you?
also, $code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/You need to assign the out parameter
i was trying to get him to actually read the error message
because that's exactly what it says 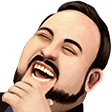
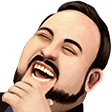

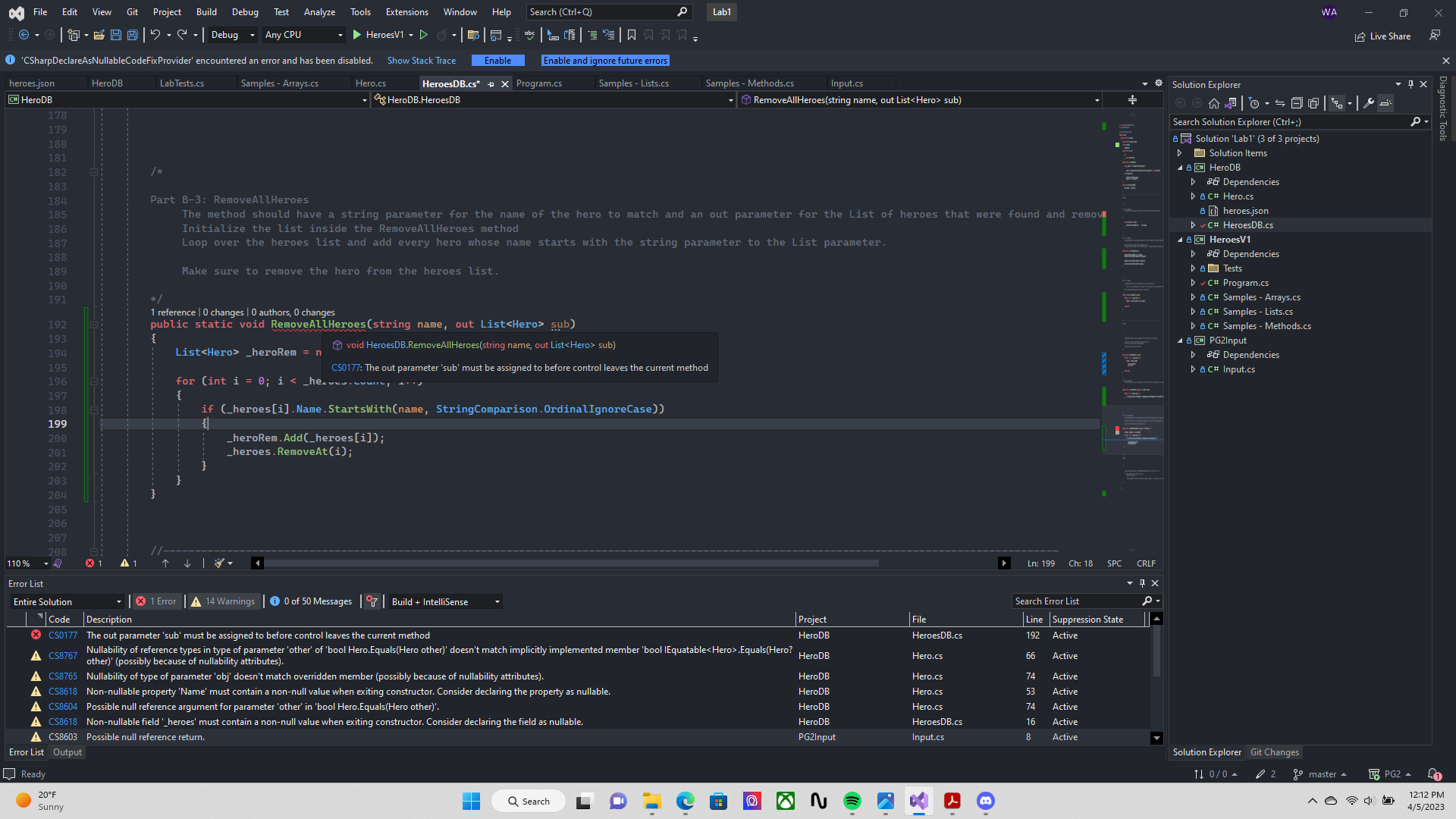
sorry new to all this
so what is the message telling you to do?
the out parameter 'sub' must be assigned to
did you try doing that?
i thought that involves the new list i created
added to that list
that list has nothing to do with the parameter in your method
sub
must have a value assigned before the method returnsYou can think of an
out
parameter as an empty box that has to be filled before you return
ok
sub
is already declared, you can't redeclare it like that
just sub = new List<Hero>();
i thought you would need that first part :/
I'm curious. Is there a performance hit with the
out
keyword as an alternative to a Tuple?out
is basically ref
with a guarantee that the method will assign a value
you'd have to bench both ways, but here you wouldn't even need a tuple since it currently returns void
yea, though in many cases i've seen
out
used its usually for things that start with the method name "Try" xDthat convention has existed before we got better tuples
Also, the way you're removing elements has a bug, you need to iterate from the end not that start, otherwise you'll miss some items
from the end of it? so like working backwords? or is it that count--
Yeah work backwards
for (int i = _heroes.Count - 1; i > 0; i--)
if you go from start to end, when you remove an item the items after it get shifted back so you'll end up skipping the item after it
oh thats really good to know never thought about it like that
also i'm pretty sure you'll hit an indexoutofrangeexception if you ever remove an item, since the count you set up your loop with is no longer accurate
Kouhai#8274
REPL Result: Success
Result: List<int>
Compile: 622.418ms | Execution: 49.956ms | React with ❌ to remove this embed.
Kouhai how are you able to show it like that
$eval
To compile C# code in Discord, use
!eval
Example:
Please don't try breaking the REPL service. Also, remember to do so in #bot-spam!ok sweet thanks
$refvsvalue
ref ⬆️
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.