26 Replies
so i have a framework/library for a 2D game and i need to add somethings to it, one of these things is tracing/logging
For example i have a method in this class, is the logging done correctly?
It's hard to gauge the specifics of your assignment, but usually logging is done through Loggers, and not with Console.Writeline
below the writeline is a logger
the writeline is to write to the screen but im not sure i should have that since this is a framework for a 2D game and there prob wont be a console for it, but this is just to test that things work in my code in a different program
Ah I see, sorry for that.
I would double check if you can't DI a logger from that framework or something like that, or hook into something they use.
Otherwise the logging itself seems fine to me
Would i have to be doing this for all methods, as in adding a logger to log whats happening, so for hit loot combat and so on right, it makes sense
I can show the log to the console right? im not sure if this is correct or not but instead of the writelines i can just log it to the console instead of something like a text file right
what do u think
Yeah sounds about right
i added this to my logger class but it doesnt write to the console and i cannot find my txt file anywhere once i build in my files
this is my logger class and app.config which is in the library and its not working in my console project that im calling it from
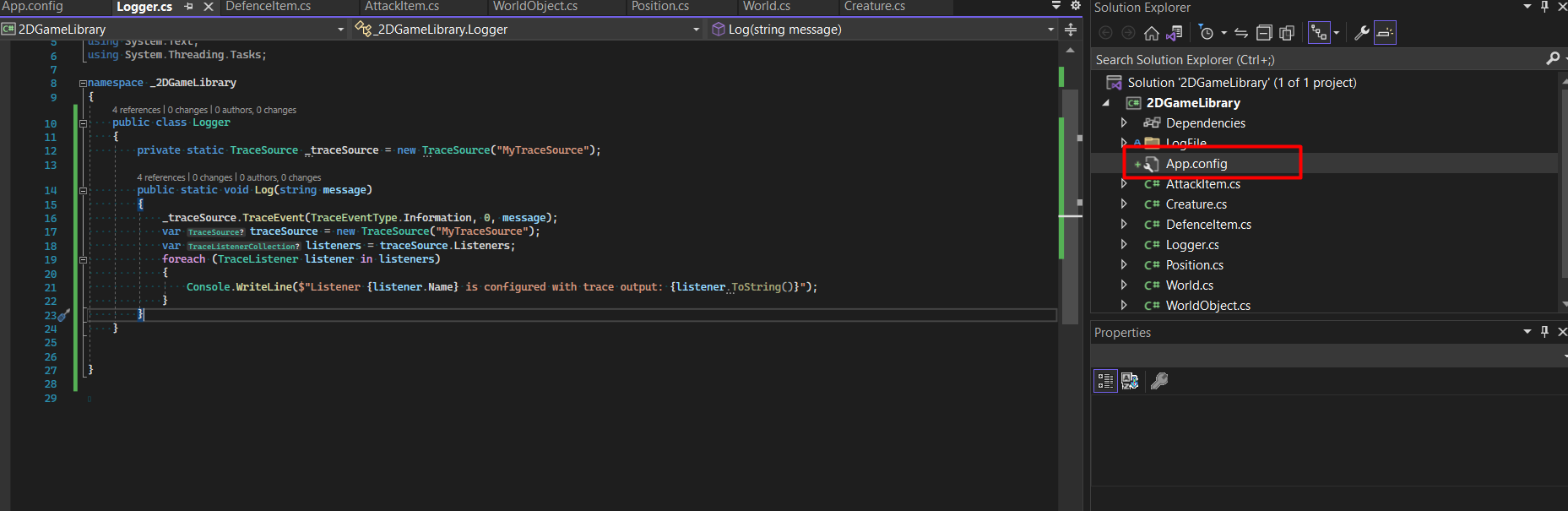
is this the correct place for the config file to be?
at runtime it should be adjacent to the exe
iirc
whats the exe here if this is a library and not a console app
Libraries shouldn't really have config files
The normal thing is for the executable to have a config file
"The framework must be configurable from a configuration-file" is what i need to do then following this,
"The framework must support tracing/logging messages"
I'm not sure about the ins and outs of your assignment but generally in .NET things use the Microsoft logging packages
A library usually depends on
Microsoft.Extensions.Logging.Abstractions
and accepts an ILogger<Whatever>
And it's up to the executable to provide it
Have you not been given any direction for your assignment?the assingment is just making a 2D turn based game framework
and it has requirments
i dont really understand what it means by "The framework must be configurable from a configuration-file" but i know for tracing it needs to be done right
Have you been working on similar things as part of your course?
i missed the lesson on tracing/configuration but this is all there is to the content

the slides dont mention anything about configuration
mostly talks about logging and tracing
i think its referring to that then right
I would guess so yeah
https://discord.com/channels/143867839282020352/1093214455669723288/1093243884764667985 do u see something wrong with this then
Protagonist#8031
this is my logger class and app.config which is in the library and its not working in my console project that im calling it from
Quoted by
<@!1019262695997968415> from #Assignment Overview help (click here)
React with ❌ to remove this embed.
it doesnt seem to me displaying on the console
So your framework needs to display trace messages and then the executable can configure them
im sure the framework would log the trace messages and when framework is being used by consoleapp for example it can then display it to the console
but i want to do it from both the console and a txt file
neither works tho
Listener Default is configured with trace output: System.Diagnostics.DefaultTraceListener
it still seems to be using defaultTraceListener
so guys I've made the logging work and printing to the console, but whats the configuration file for?
also the txt file doesnt seem to log the tracing
How do i make my library configurable
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.
Was this issue resolved? If so, run /close
- otherwise I will mark this as stale and this post will be archived until there is new activity.