❔ ✅ abstract-Parent and Child static overrides?
How do I redeclare an objects element which needs be from a static context in a child class?
I have an abstract parent class:
and some child classes (here's an example):
where in I'm trying to override
prefabBlock
and want to give it a color RawColor
; RawColor
requires it's Color24 be static. I have been struggling with this massively.28 Replies
Note: the childs
prefabBlock
is not overriding the parents, so the parents is the one getting through to the base methods of the child.Is what you're trying to do is
ResizableGatePrefab.color_t = Color24(0,0,0)
ResizableAndGatePrefab.color_ = new Color24(127,127,127)
?
You can't mark static methods and properties as abstract or virtual, your best option would be to hide the parent.s color_t with new
new public static Color24 color_t = new Color24(127,127,127);
I've tried
Child:
and the color is still (0,0,0) for the child objects
Can you show how you're using it?
What do you mean?
Like how are you testing that the color is still (0.0.0)
In unity, these two objects have a prefab which starts in their child classes.
These are two child classes of ResizableGatePrefab which have colors other than (0,0,0) (or close) defined; however, both of this still use their parents default color (0,0,0)
When I don't declare the default object I struggle with declaring it in the child because passing static objects in C# is- more complicated than I expected (or impossible?)
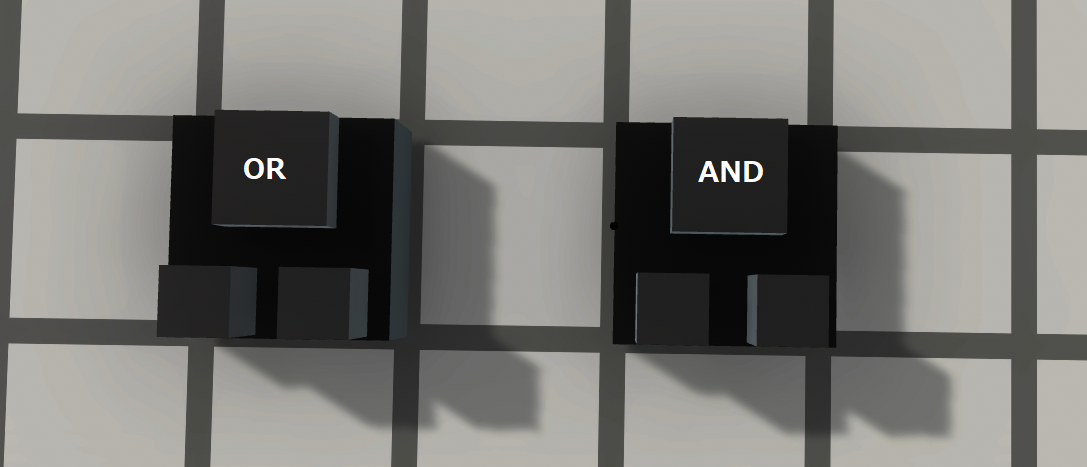
I struggle to see why you have color as a static.
Block
has a RawColor property right?I wish I knew why color needed to be static too.
the
prefabBlock
struct requires that RawColor
be a static Color24.. for whatever reason.
I don't like it either.How does it require it to be static?
I'm not that well versed in unity but how would they enforce that?
I can change it and show you the compile error, one sec
"A field initializer cannot reference the non-static field" :c sad
When I make it static, it works.. but every instance takes on the parent one (0,0,0)
Yes, field initializers can't reference other fields.
Properties on the other hand can reference them
So
Works
They're still (0,0,0); I tried it with and without new's for hiding. I'll keep testing.
no matter which way I change the child to have new hidings and => expressions, it doesn't change the color.
Okay, how are you using RawColor in 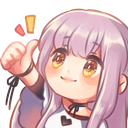
Block
?
If possible, please show the code for Block as well 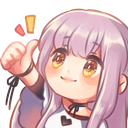
Child:
Block:
This was under some random dll in the build
Lemme find IRenderData too-
That one was easier to find:
Note: the only code shown I wrote are in ResizableGatePrefab and ResizableAndGatePrefab
But, I still can't really tell how the
RawColor
is getting used, I can't imagine it being checked on every Update()
callHere's Color24 too, just in case:
https://paste.mod.gg/odsfsmwgwths/0
BlazeBin - odsfsmwgwths
A tool for sharing your source code with the world!
Color24 seems okay
ClydeBot won't let me send the rest.
😅
$paste
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
BlazeBin - odsfsmwgwths
A tool for sharing your source code with the world!
I should state, I believe much of this code I'm looking at is owned by some company
Yeah
The question is, how is RawColor accessed?
Like if you set RawColor in an update call, does the color change?
Still (0,0,0)
:c
heck (well I already tried that so I'm not really surprised, just sad)
Update() as the unity thing?
Yeah, set it in Update
I found a solution
!close
Closed!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.