❔ Optimizing code
Currently I am refactoring some code in my WPF app as some parts load quite slow. I am wondering what is the correct way to use stuff like
Parallel.ForEach
Task.WhenAll
and
Task.Run
For example I have a for loop that iterates through a collection and makes an API call for each, they are not really dependent on each other. So is that a good case for Parallel.ForEach
? (ignoring the fact that I need to optimize by making a single API call 😅 )
Then I have some initialization methods on my ViewModels that gather data with a few API calls. Like one to get personnel and another to get app data like settings. Is that a good case for Task.WhenAll
?
and if I have a async Task
that I don't want to await
as it's loading the notifications panel rather than a page, can I just run that in Task.Run
? Without awaiting it?
18 Replies
these maybe feel like bandaid solutions? have you analyzed if those spots are really causing issues? have you tried optimizing the code that's the problem?
Yes, I used the profiler earlier and found some methods that are slow. My first example about the loop of API calls. Was looping 330 objects 😩 I filtered the data to only get the relevant stuff and that helped. Just wondering if Parallel.ForEach is a good idea too.
could you show those particular methods? or is it like sensitive data
Initialize
lol
also you should probably await that instead of Task.Run
ning itunfortunately it is Initialise in british english...
oh
looks weird
just like practise...
my
actually makes more sense
but im not used to it
plus the rest of english doesnt make sense, so why should this
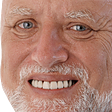
I was thinking that I don't want to await it though, I just want it to run in the background and not block execution
then just call it and dont await
For example I have a for loop that iterates through a collection and makes an API call for each, they are not really dependent on each other. So is that a good case for Parallel.ForEach
?
no thats not what Parallel.ForEach
is for
for this, you could create a list of tasks and call each method and add the task to the list
then Task.WhenAll
Then I have some initialization methods on my ViewModels that gather data with a few API calls. Like one to get personnel and another to get app data like settings. Is that a good case for Task.WhenAll
?
a general rule of thumb that i follow is that if you dont need to sequentially await, then dont and use Task.WhenAll
and if I have a asyncdontTask
that I don't want to await as it's loading the notifications panel rather than a page, can I just run that inTask.Run
? Without awaiting it?
Task.Run
it, do _ = DoSomethingAsync();
if you dont want to awaitSo then this is fine?
no
it should return a task
It's an
override
from a library so I can't really change itThey also said it's WPF
So Task is just out of the question for most things
oh
its not
It is
just for control event handlers
i use wpf
if you dont await, exceptions will be swallowed
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.