✅ Sending an HTTP Exception in Web API
I am having some trouble with sending an HTTP exception in web api as it seems how its dont has changed over the course of different .net versions.
There seems to be an issue of what return type I have, but I found examples online where people were able to do it with an ActionResult.
I found examples which implemented it like this but it will not compile for me because it cannot convert the return type.
I have also tried what is shown on the learn microsoft page where it says essentially to do
However doing so causes my server to encounter an unhandled exception and cease to work.
What is the correct way of doing this in .net 6???
26 Replies
return NotFound(...)
Also, this should probably be an async method, given that you are accessing a database in itWhat dependency is NotFound under?
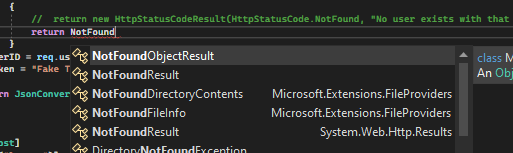
I will take this into consideration and bring this up with my group at the next meeting
can you show the controller declaration?
NotFound
is a method from ControllerBase
also, this worries me: return JsonConvert.SerializeObject(r);
its newtonsoft, and you are doing response serialization manuallyAha! Okay, yes my issue was with the controller base being an ambigious reference because of another solution I attempted earlier
Thank you so much!!! NotFound exists now and I can get it
with .net 5 and above, its recommended to use the built in json serializer (STJ) instead of newtonsoft, unless you actively need a feature only newtonsoft has
and you shouldnt be manually doing the serialization in 99% of cases
its better to just return the object
As far as the manual serialization goes its because my front end dev wants to do some weird stuff
Oh hmmm I see
will that manually seriallize it into json?
automatically, but yes 😛
err autmoatically i mean
weiiird we had some issues with that which is why we switched to doing it this way. Tho i wouldn't put it past something having been wrong in the front end when we tried it 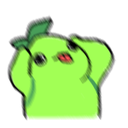
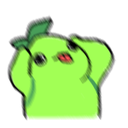
so you'd set your response type to be
ActionResult<LoginReturn>
well the way you have it right now
you are returning a json string, containing json
ie, your return will be...
instead of returning actual json@.@
Wow thats a blunder
Its a miracle it works rn...
feel free to doublecheck
but thats what it looks like to me
unless you've disabled the automatic response serializer in the pipeline
hmmm maybe I have cause looking at my response it seems to be received as just json
can you share your
Program.cs
without exposing any secrets?Getting things seriallized properly was a headache a few months ago and I don't remember everything we did to sort it out
Sure lemme grab it, this is just a long term project for college so
ah
hm
I see nothing touching the serializer at all
Hmmm... I'll look into it a bit more and bring that up at my next meeting as well. Thanks so much for all the help, I really appreciate it. It's so hard to find good advice for this on the internet because .net stuff has changed so much over time. You're a life saver
okay it wont be stringwrapped
but its still wrong
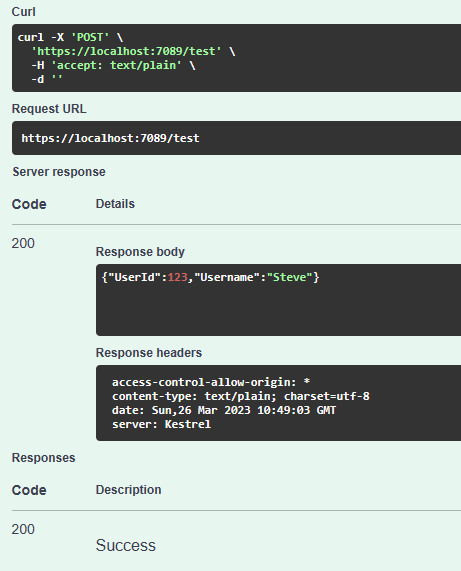
the content-type is
text/plain
and this is what it looks like with my suggested changes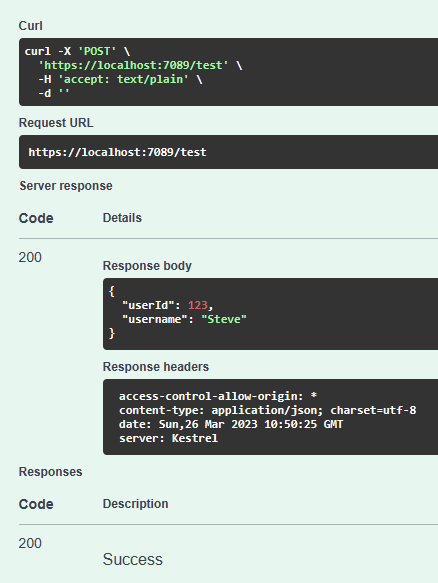
properties are using
camelCase
names, as is standard in web.
content-type is application/json
, as it should be
and my code doesnt need to bother with serializing, which leads to less code 🙂Amazing!!! Thanks so much!!!
You can
/close
the thread if you don't have any further questions.