Rendering function vs variable assignment
Hey everyone,
I'm wondering if anyone knows whether there's a difference between having a rendering function and variable assignment. To have a better understanding of problem imagine a scenario where depending on some value You need to render an icon. You create a map where keys are the values and keys' values are icons. This can be done in two ways. First with variable assignment:
And second with rendering functions:
Does anyone know whether there's a benefit or some loss to any of these approaches (maybe bigger build size but quicker runtime or other way round)? Or maybe there's another, more proper way of doing this? Thanks!
9 Replies
I don't even think the first one works
It does somehow
This is the only difference I can find
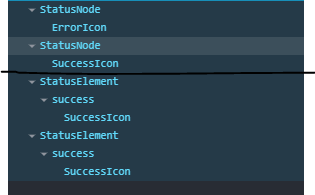
TLDR it's irrelevant
Yeah, thought so. Thanks!!
It is very relevant. You are redefining actual React components if you do this:
It is perfectly fine though to treat it as just a normal function can call it in your JSX though:
In your example its not so egregious. But if you did have React state and other hooks then you'll encounter weird behaviours as state will no longer persist between renders
Read more: https://github.com/jsx-eslint/eslint-plugin-react/blob/master/docs/rules/no-unstable-nested-components.md
GitHub
eslint-plugin-react/no-unstable-nested-components.md at master · js...
React-specific linting rules for ESLint. Contribute to jsx-eslint/eslint-plugin-react development by creating an account on GitHub.
And if its not a function in the map then just doing
{icon}
also works in your JSX tooI don’t really agree, we are dealing with SVG’s here, but yeah if the components had state you’d end up with “super components” which can lead to unexpected behavior