Stuck on some things involving listbox and textbox.
I have a simple application where the user inputs information into textboxes, and when they press a “save” button that information displays in a listbox. The idea is to create a record of customers for a store. The attached photo is my example. Right now Im trying to create the functionality for the “first” and “last” buttons, which are supposed to display the information from the list box in the text boxes. I don’t know how to go about doing that, though, and after about 3 hours of looking on youtube and google without getting any less stuck im coming here.
Another thing i am stuck on is the counter next to customer # at the top and record # on the left in the bottom list box. What ive done to try and get it to count like it should is create a label where the counter will go and put this code under the save button:
label6.Text += listBox1.Items.Count;
it sort of works but instead of counting like 1, then turning into 2, and so on it just keeps adding numbers like 1, then 12, then 123.
The functionality behind the first and last buttons are more important right now but i immensely appreciate any help or advice on any of this.
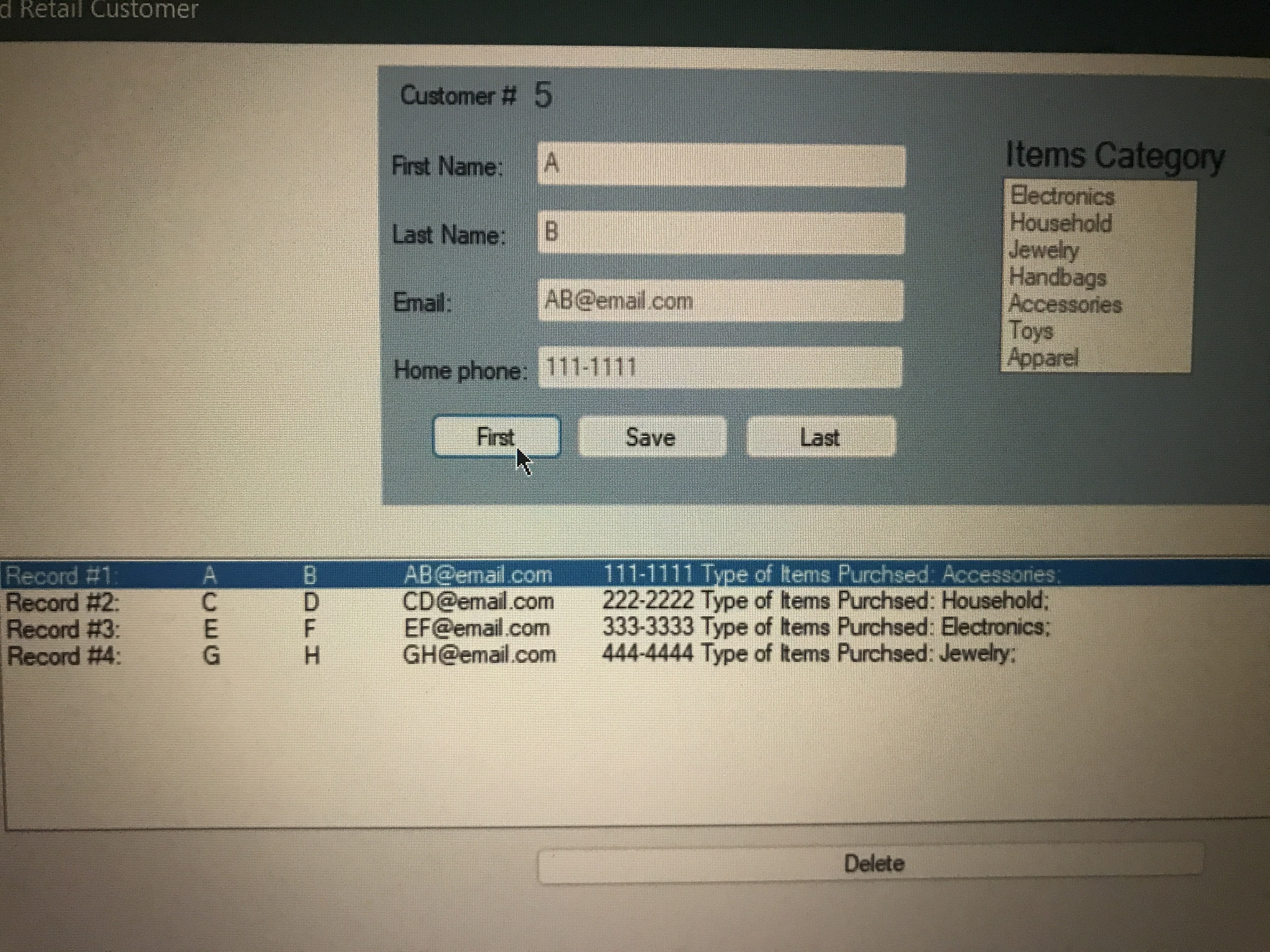
79 Replies
the
label6.Text += listBox1.Items.Count;
issue is a simple one:
label6.Text
is a string
, string + anything
will also result in a string, basically it calls string + anything.ToString()
, eg "1" + 2
-> "1" + 2.ToString()
-> "1" + "2"
-> "12"
u basically have 2 ways of doing that: either store a counter variable somewhere public int nextId = 1;
and then do label6.Text = (nextId++).ToString();
(would prefer this), or convert the label's text to an int
first: label6.Text = int.Parse(label6.Text) + 1;
to the other part i come in ~30min got an important phone call rnNo, the counter issue you fix by using string Interpolation
`Text = $"Customer #{listbox.Itema.Count}";
regarding the last/first functionality.
usually u store the data in its own type and throw it in a list or something like
public List<Customer> customers = new List<Customer>();
then i would write a method that takes a Customer
object and updates the gui respectively
public void UpdateGUi(Customer customer)
then on first/last u simply get the respective customer object from the list (dont forget to check if the list is empty) and call UpdateGui()
from there
that wouldnt work, lets take the data from the screen shot as example, if they delete one entry thats not the last and they create a new entry, they end up with #4 twice
with the counter and list approach u can also load data stored in a file so u can "continue" after the program was restartedfirst of all thank you both for replying, and i tried all the suggestions for the counter and label6.Text = int.Parse(label6.Text) + 1;
seems to work the best except i got one error message “cannot explicitly convert type int to string” so i think im missing a step
oh, right, u would have to wrap it in parenthesis and call
ToString()
on it
(int.Parse(label6.Text) + 1).ToString()
also i would recommend giving ur components more self-explanatory names, eg. customerNumberLabel
or alike. that way u dont have to memorize which numbered label is for what
also usually u should also mention which GUI frame work u r using (winforms, wpf, mau, avalonia, etc) when asking GUI questions - tho here it didnt matterthat got rid of the error and i was able to run it but i ran into an exception i think its called, “imput string was not in a correct format”
oh thank you, i’ll do that next time
can u show me the code line where the exception was thrown?
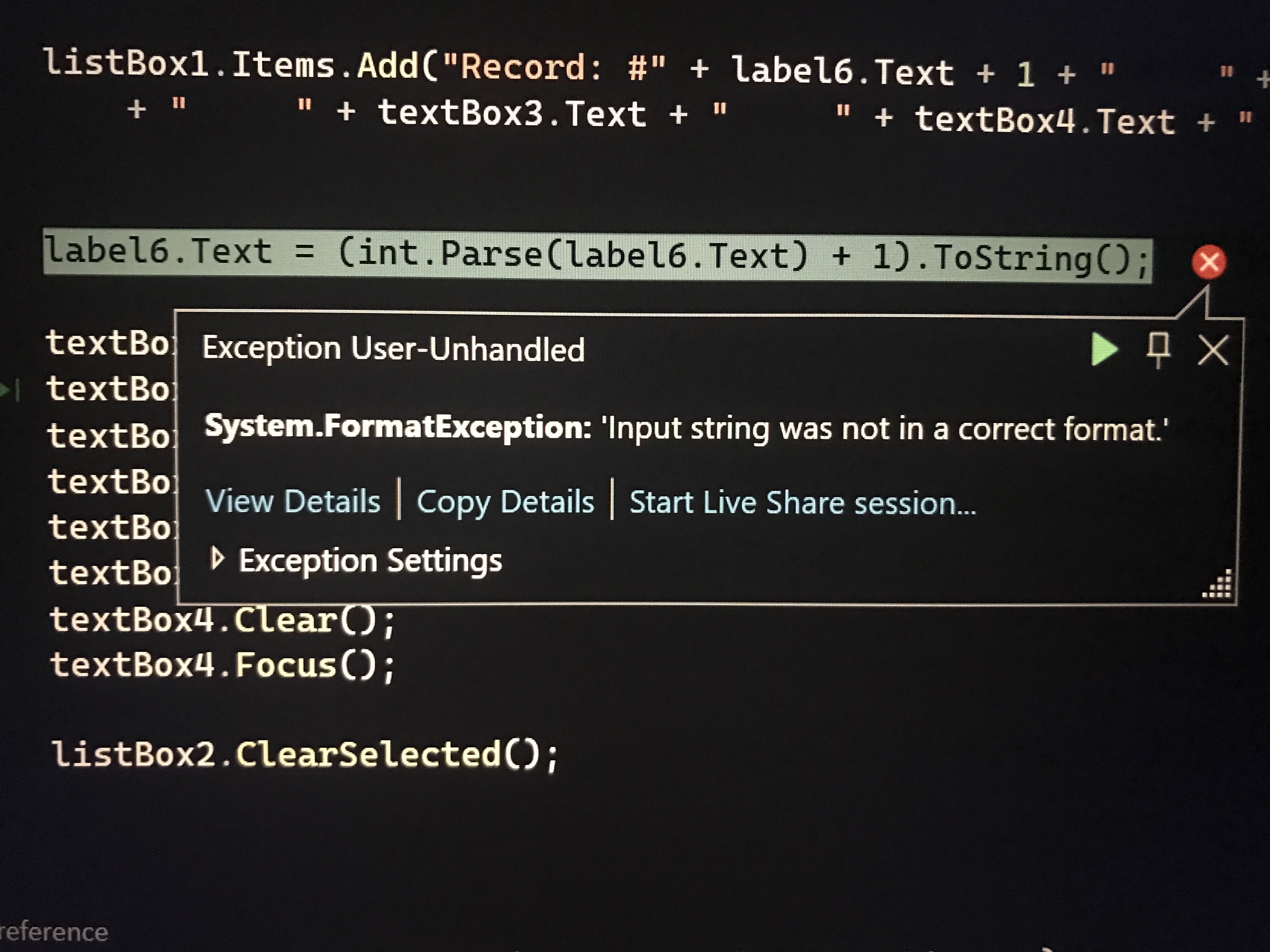
It means you tries to parse something that wasn't a number into a number
So label6.Text contains something that isn't a number
aaah, so
Customer # 5
from the screenshot is the whole label6
? then that variant doesnt work (thats why i recommended the counter approach)
in that case i would recommend the counter + Pobiega's approach: label6.Text = $"Customer # {nextId++}";
It's usually a good idea to separate your program state from your presentation when possible, so the counter idea is good
Means the label only shows values, it is never used as a "source of truth"
“nextID does not exist in the current context”
u will have to create the member somewhere
public int nextId = 1;
should i put it under the “save” event too?
can u elaborate?
No, it will need to go at a higher scope so it remembers the value
"save" is temporary
$scope
you probably want
$scopes
$scopes
thing a
is available in scope A
and scope B
thing b
is available only in scope B
The thing inside B will go away when B ends
Scope A here is most likely your Form class
i dont think this is the correct place either
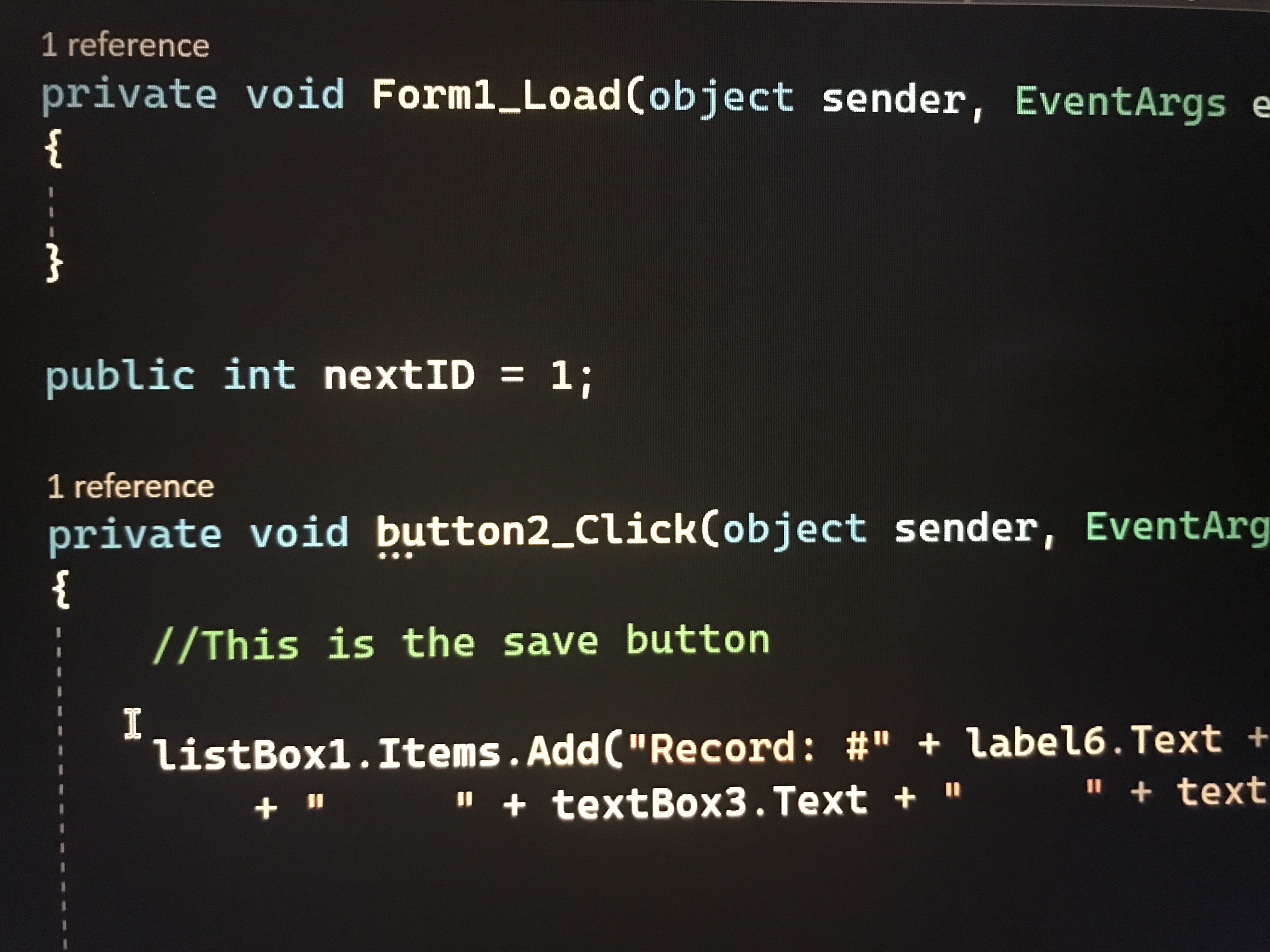
it more or less is, u can use it from any method inside that class by
nextID
You'd normally place that at the top of the class, and it should be
private
But what you have is legal 🙂it still says that nextID doesnt exist in the current context :/
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/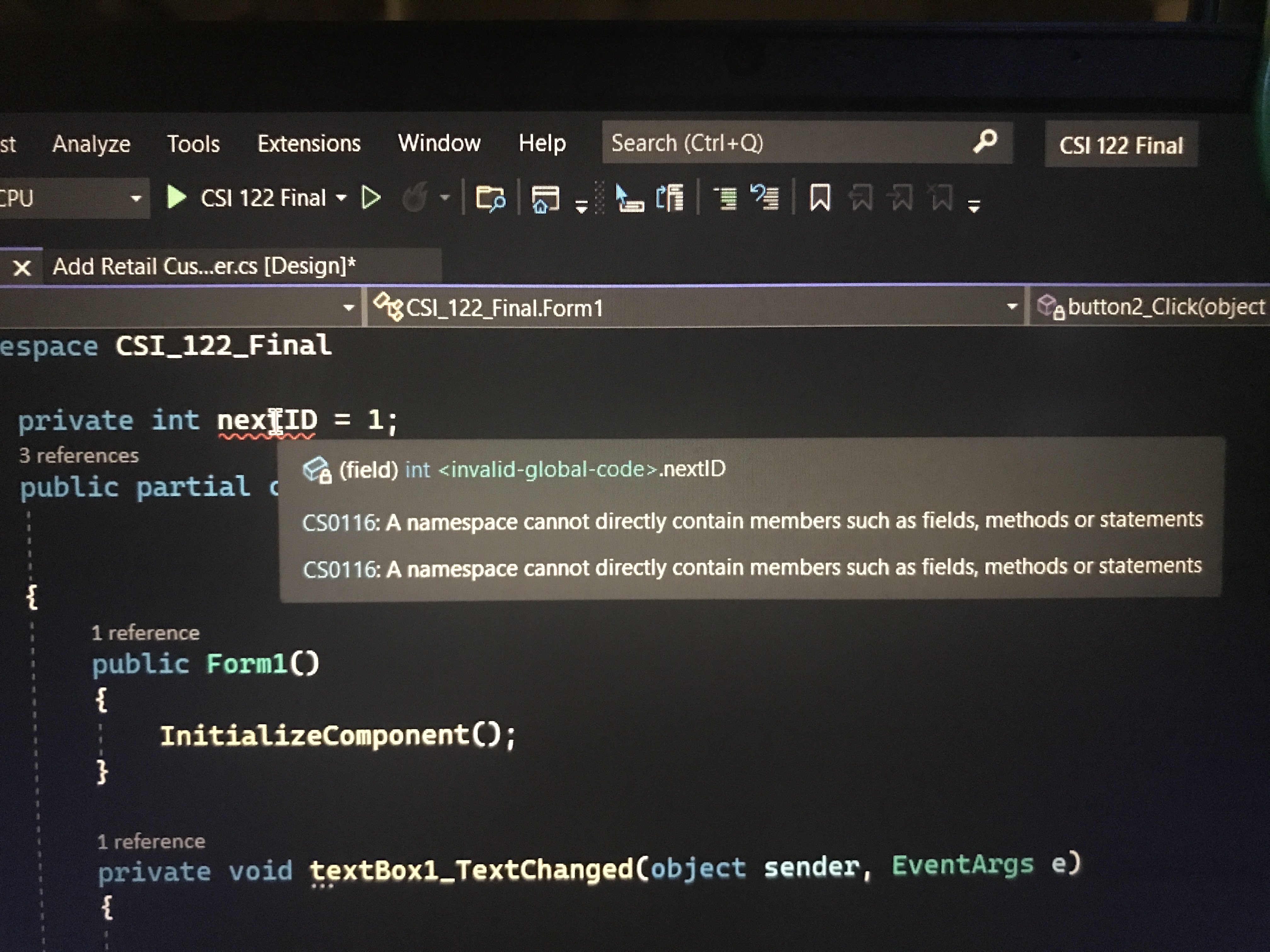
I said top of class, not on top of your class 🙂
It goes inside the class
ohhhh i see
do i put it in brackets? putting it in class seems to throw the whole thing off because of these {}
The brackets are very important
They define the class scope
So yes, it must go inside the brackets
There should never be anything I'm between
You should take some time to practice the fundamentals of C# when possible
i am taking more classes in the following months, thank you so much for all of your patience
Don't assume that class-time will be enough
You will need to put in some extra time and effort
You are currently learning both C# and how to think like a programmer
That's quite a bit of cognitive load
well it still says that the name doesn’t exist, but there are no bracket errors now
Show the code.
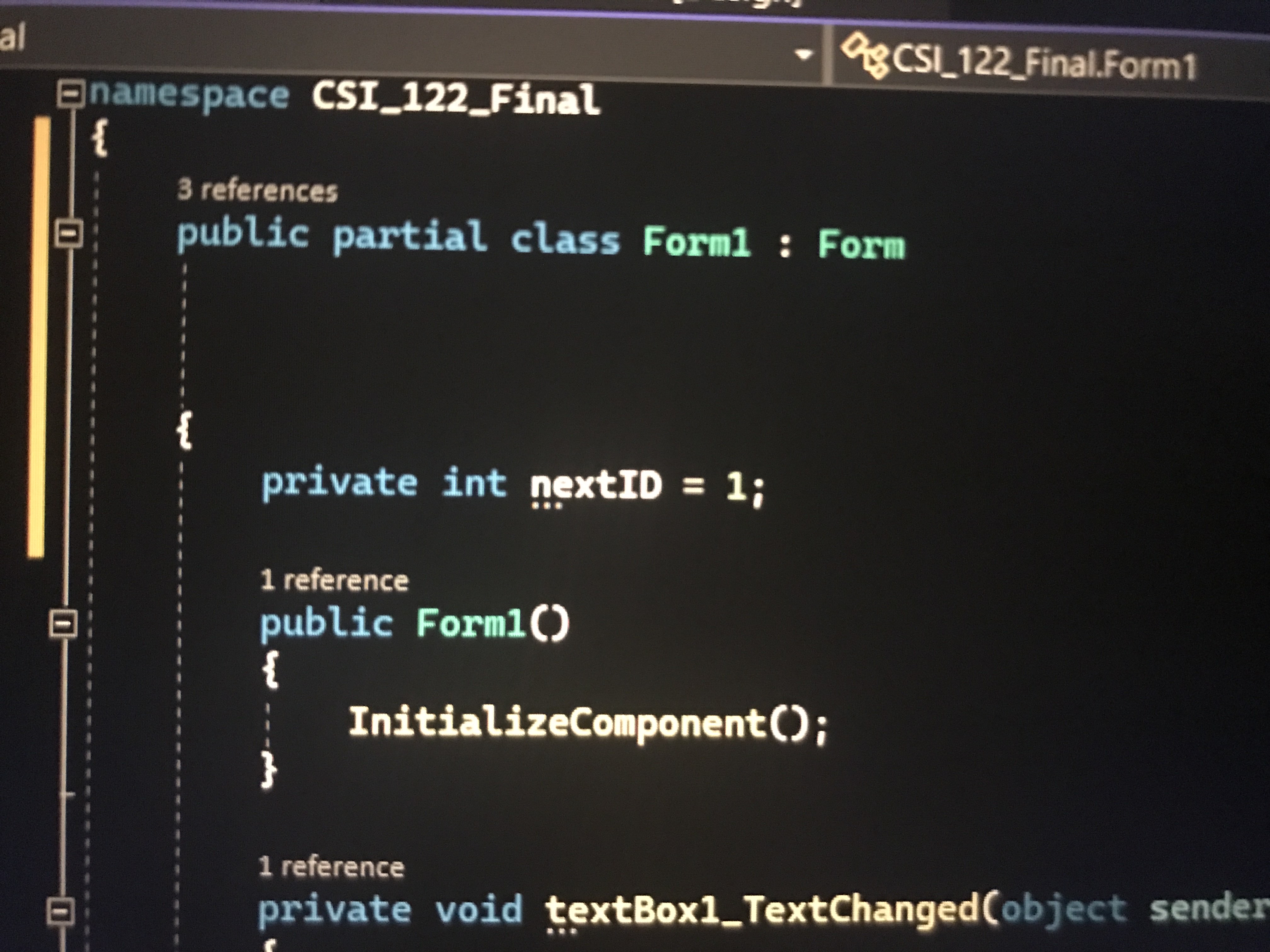
Yep, that looks fine. Show where the error is?
Oh and remove those blank lines haha
They are just confusing
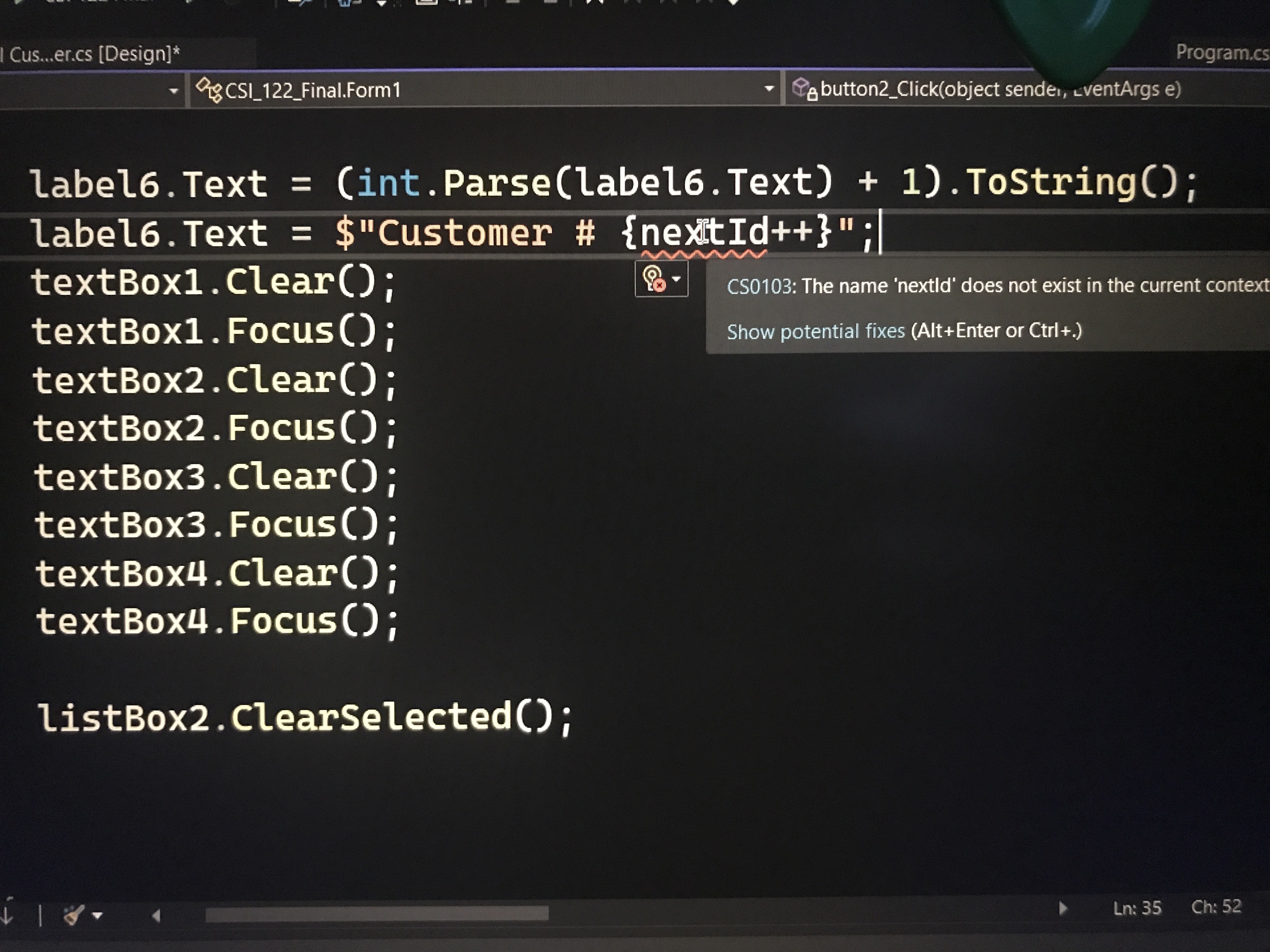
That doesn't make sense. Can you copy paste the entire file to $paste please?
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
Need to see the full thing, these snippets obscure a lot of details
u called it nextID and use it as nextId
Ah, yup
nextID is a completely different name than nextId
Code is case sensitive
okay i was able to run the app, but i ran into another exception
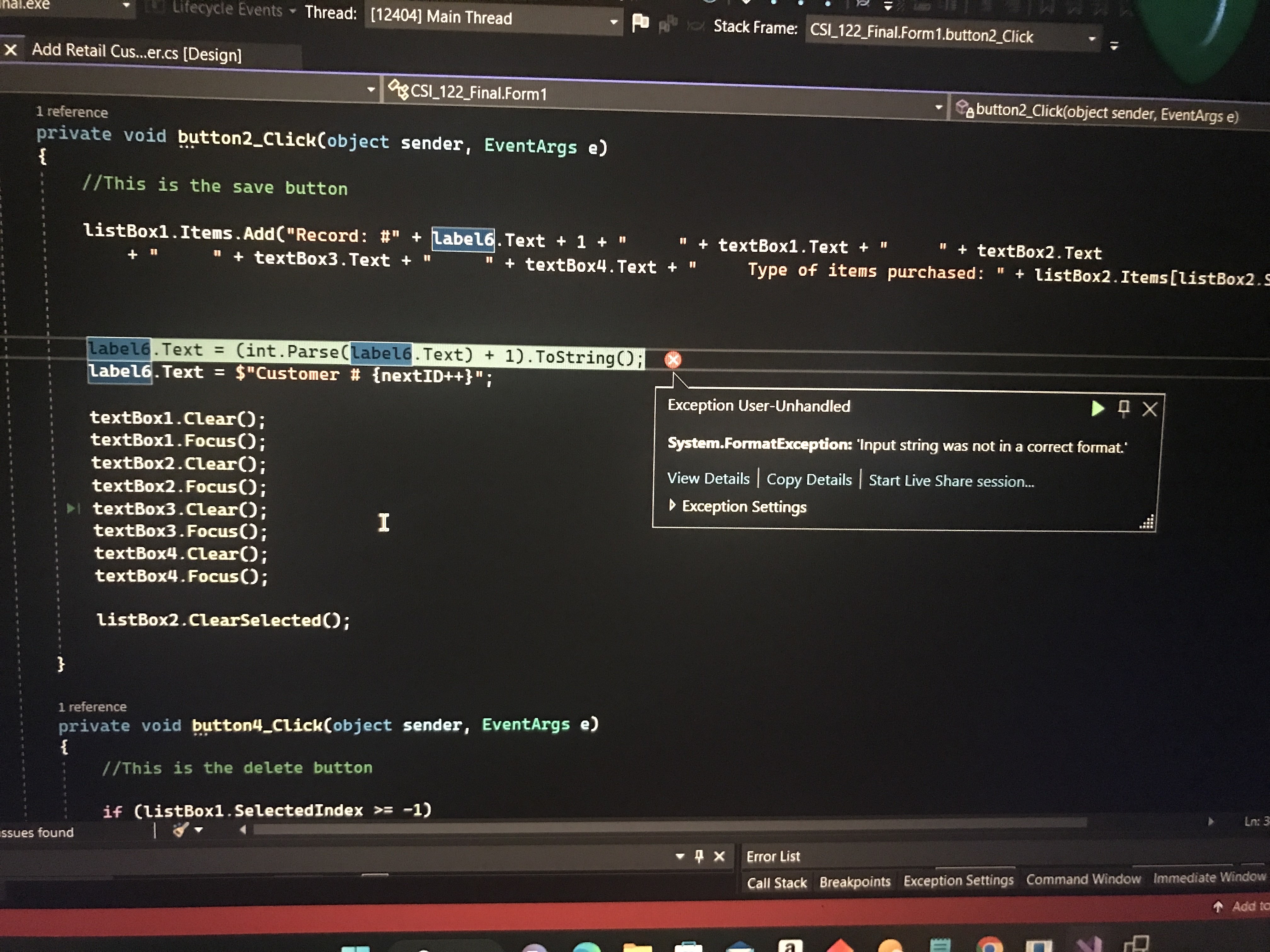
that line is obsolete now, just remove it
its complaining because "Customer # <some number>" isnt a number ;p
thats why we use the counter and
label6.Text = $"...";
insteadoh right, sorry im running into mistakes like that bc i was really stressed but im calming down now
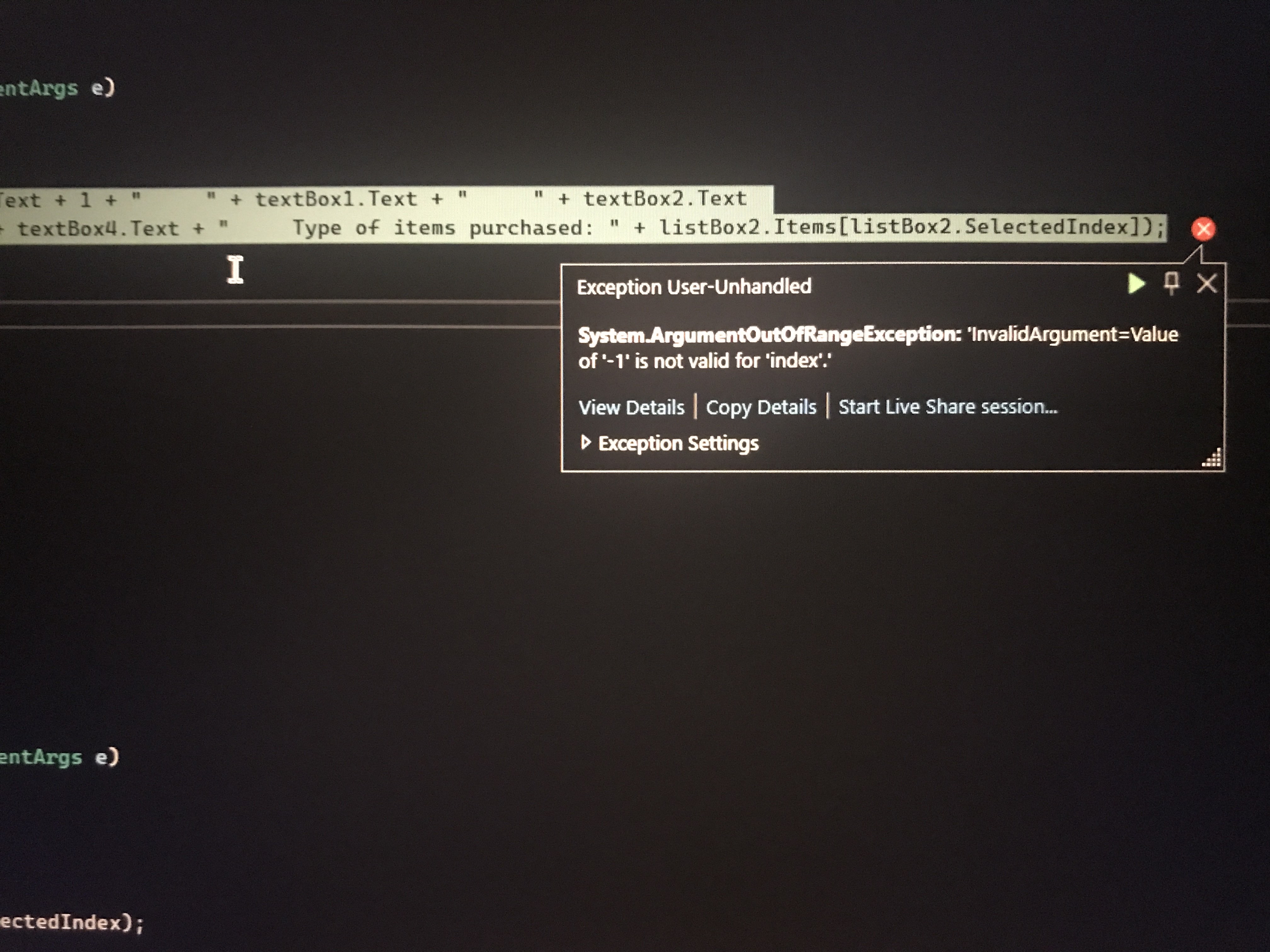
SelectedIndex
of -1 means there is no item selected, thus u need to handle this edge case:
I think it might be time to start talking about the elephant in the code here, the fact that your listbox entries are giant strings 🙂
when you add a new entry, you build a big string from a bunch of separate values. This works, as you can see, but now each individual value no longer exists - its all one big string.
so for the "put data from listbox into textboxes again" thing, this is now... really hard, because of the above fact
there comes that into account again ;p
^
ohhh i think i understand. can i still make it work or would i have to redo the listbox entries?
btw u can get rid of all but the last
.Focus()
callyou can easily fix this
u would have to adjust some stuff once u have something like a
Customer
class, but thats easily doneYou need to make your own type to represent a customer
and if that type were to override the
ToString
method, that would be good 😛
full separation of state and presentation is the dream, but it will be harder for a beginnerim just trying to get it to run without running into exceptions, even if it doesn’t totally work (at least for tonight, i have to be asleep in a couple hours)
You should have all you need for that, if you put the first/last buttons and editing rows on hold for now
i put everything under the “save” button into the “if” part of this, and put a messagebox.show(“input info in all fields”); in the “else” part. where would i put the customer class
I thought you said you wanted to wait with that?
im still trying to get rid of the exception
what exception?
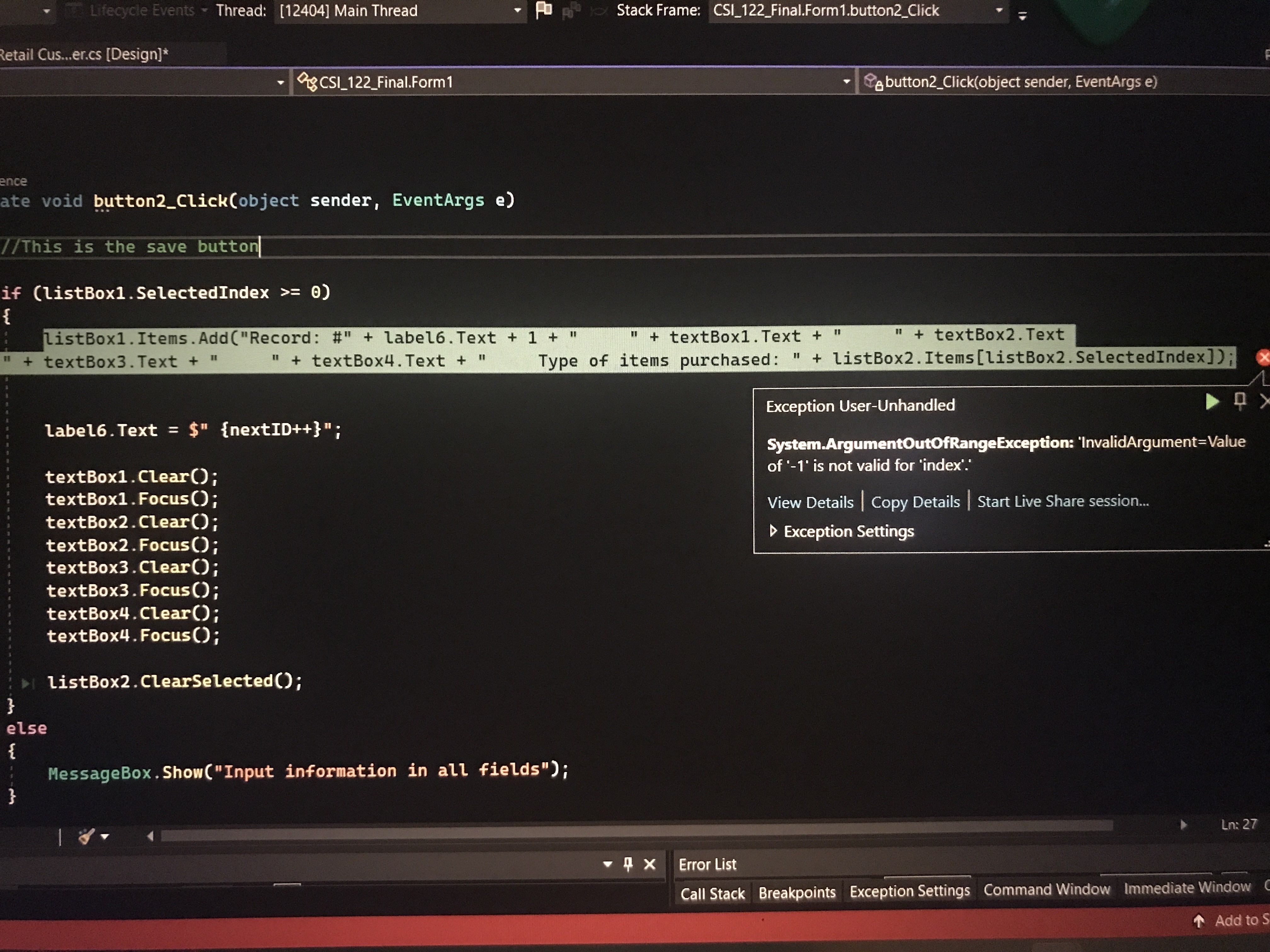
u check
listBox1.SelectedIndex
, instead of listBox2
'sit still says the same thing
show. code.
we have no idea what you changed or how
also, consider just using discord on the computer so you can share code by copypaste instead of camera :d
ill switch rn
okay this is the whole thing
so if
listbox1.SelectedIndex
is valid, use listbox2.SelectedIndex
to access the value... from listbox1
?
does that make sense?
Sorry to say but this looks like just randomly throwing things at the screen to see what sticks :/it was rushed but i dont know what to change, the listbox2.select index is supposed to access whatever was selected from the "electronics, household, jewelry, etc" list
Think about it
What are you trying to access?
You want to get the currently selected item from
listbox2
.
How do we do that? What values must be valid for that to make sense?Well it says the value of -1 isnt valid, so it has something to do with the way it is processing the values within the lists?
Read my last 3 messages again.
think about what
listBox1.Items[listBox2.SelectedIndex]
does, it takes the index of the selected item of listBox2
and then u try to get an item of listBox1
at the given index
is that what should happen?and tbh, if you just want the currently selected item, use the
SelectedItem
property :p
"if something is selected, get the currently selected item"
we already know SelectedIndex
returns -1 if nothing is selectedtotally forgot about that xD
Hey I wanted to come back for a sec and let you guys know I'm done with the app, I got it to where it'd do most of what its supposed to and I also found someone I know irl that happens to know C# too, so I can reach out to him for help as well. More importantly I wanted to thank you both so much for how much time you took helping me out and being patient with me and genuinely explaining things. Your help made a huge difference for me. I immensely appreciate it beyond what I can express right now so again a huge huge thank you!! Have a good rest of your day/night 🙂
glad i could help, also if u r done here please use /close to mark the thread as answered
you're welcome. please
/close
the thread if you dont want to ask more related questions 🙂