How to write a specific script
I need a script that can turn this:
into this:
"translation": {
"x": -0.15,
"y": -0.22,
"z": 0
},
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.8,
"y": 0,
"z": 0.5
}
"translation": {
"x": -0.15,
"y": -0.22,
"z": 0
},
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.8,
"y": 0,
"z": 0.5
}
tf.Translation.X = -0.15f;
tf.Translation.Y = -0.22f;
tf.Translation.Z = 0;
tf.Rotation.X = 0;
tf.Rotation.Y = 45;
tf.Rotation.Z = 0;
tf.Origin.X = 0.8f;
tf.Origin.Y = 0;
tf.Origin.Z = 0.5f;
tf.Translation.X = -0.15f;
tf.Translation.Y = -0.22f;
tf.Translation.Z = 0;
tf.Rotation.X = 0;
tf.Rotation.Y = 45;
tf.Rotation.Z = 0;
tf.Origin.X = 0.8f;
tf.Origin.Y = 0;
tf.Origin.Z = 0.5f;
31 Replies
The input format is JSON
have you looked at what's available in System.Text.Json?
Not yet
What class can do that from object?
Or from string
you should google that namespace i mentioned
$json
How to serialize and deserialize JSON using C# - .NET
Learn how to use the System.Text.Json namespace to serialize to and deserialize from JSON in .NET. Includes sample code.
I tried this
It doesn't work
using System.Text.Json;
namespace CodeFromJson
{
public class ModelTransform
{
public Vec3f Translation;
public Vec3f Rotation;
public Vec3f Origin;
public float Scale;
}
public class Vec3f
{
public float X;
public float Y;
public float Z;
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<ModelTransform>(jsonString);
Console.WriteLine($"tf.Translation.X = {tf?.Translation.X}");
Console.WriteLine($"tf.Translation.Y = {tf?.Translation.Y}");
Console.WriteLine($"tf.Translation.Z = {tf?.Translation.Z}");
Console.WriteLine($"tf.Origin.X = {tf?.Origin.X}");
Console.WriteLine($"tf.Origin.Y = {tf?.Origin.Y}");
Console.WriteLine($"tf.Origin.Z = {tf?.Origin.Z}");
Console.WriteLine($"tf.Rotation.X = {tf?.Rotation.X}");
Console.WriteLine($"tf.Rotation.Y = {tf?.Rotation.Y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.Rotation.Z}");
Console.WriteLine($"tf.Scale = {tf?.Scale}");
}
}
}
using System.Text.Json;
namespace CodeFromJson
{
public class ModelTransform
{
public Vec3f Translation;
public Vec3f Rotation;
public Vec3f Origin;
public float Scale;
}
public class Vec3f
{
public float X;
public float Y;
public float Z;
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<ModelTransform>(jsonString);
Console.WriteLine($"tf.Translation.X = {tf?.Translation.X}");
Console.WriteLine($"tf.Translation.Y = {tf?.Translation.Y}");
Console.WriteLine($"tf.Translation.Z = {tf?.Translation.Z}");
Console.WriteLine($"tf.Origin.X = {tf?.Origin.X}");
Console.WriteLine($"tf.Origin.Y = {tf?.Origin.Y}");
Console.WriteLine($"tf.Origin.Z = {tf?.Origin.Z}");
Console.WriteLine($"tf.Rotation.X = {tf?.Rotation.X}");
Console.WriteLine($"tf.Rotation.Y = {tf?.Rotation.Y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.Rotation.Z}");
Console.WriteLine($"tf.Scale = {tf?.Scale}");
}
}
}
probably because this json
is invalid
"translation": {
"x": -0.15,
"y": -0.22,
"z": 0
},
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.8,
"y": 0,
"z": 0.5
}
"translation": {
"x": -0.15,
"y": -0.22,
"z": 0
},
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.8,
"y": 0,
"z": 0.5
}
I input this line
"groundStorageTransform": { "rotation": { "x": 0, "y": 45, "z": 0 }, "origin": { "x": 0.5, "y": 0, "z": 0.5 }, "scale": 0.85 }
"groundStorageTransform": { "rotation": { "x": 0, "y": 45, "z": 0 }, "origin": { "x": 0.5, "y": 0, "z": 0.5 }, "scale": 0.85 }
invalid
Why?
How I can fix it?
{
"groundStorageTransform": {
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.5,
"y": 0,
"z": 0.5
},
"scale": 0.85
}
}
{
"groundStorageTransform": {
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.5,
"y": 0,
"z": 0.5
},
"scale": 0.85
}
}
It is strange
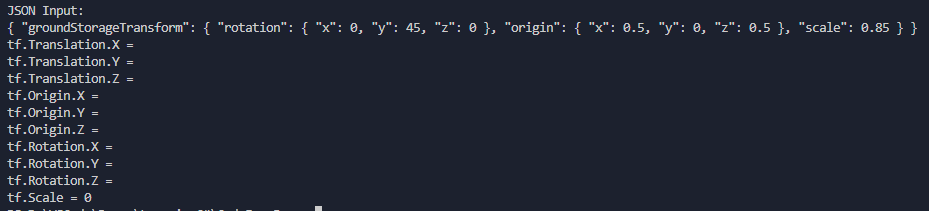
the problem is in your model
this
I need to add new()?
{
"translation": {
"x": -0.15,
"y": -0.22,
"z": 0
},
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.8,
"y": 0,
"z": 0.5
}
}
{
"translation": {
"x": -0.15,
"y": -0.22,
"z": 0
},
"rotation": {
"x": 0,
"y": 45,
"z": 0
},
"origin": {
"x": 0.8,
"y": 0,
"z": 0.5
}
}
public class Origin
{
public double x { get; set; }
public int y { get; set; }
public double z { get; set; }
}
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Rotation
{
public int x { get; set; }
public int y { get; set; }
public int z { get; set; }
}
public class Translation
{
public double x { get; set; }
public double y { get; set; }
public int z { get; set; }
}
public class Origin
{
public double x { get; set; }
public int y { get; set; }
public double z { get; set; }
}
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Rotation
{
public int x { get; set; }
public int y { get; set; }
public int z { get; set; }
}
public class Translation
{
public double x { get; set; }
public double y { get; set; }
public int z { get; set; }
}
I need only float for numbers
then get rid of the properties you don't need
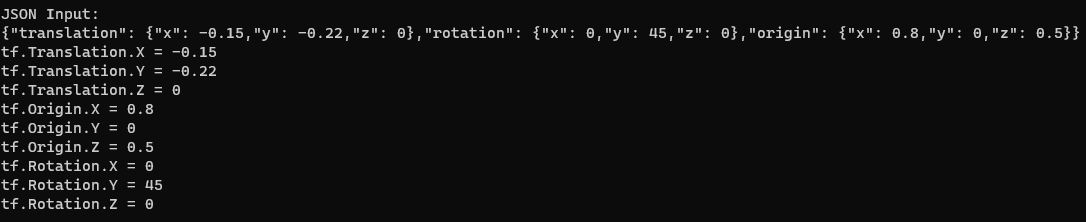
using System.Text.Json;
namespace CodeFromJson
{
public class Origin
{
public double x { get; set; }
public int y { get; set; }
public double z { get; set; }
}
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Rotation
{
public int x { get; set; }
public int y { get; set; }
public int z { get; set; }
}
public class Translation
{
public double x { get; set; }
public double y { get; set; }
public int z { get; set; }
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string? jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<Root>(jsonString);
Console.WriteLine($"tf.Translation.X = {tf.translation.x}");
Console.WriteLine($"tf.Translation.Y = {tf?.translation.y}");
Console.WriteLine($"tf.Translation.Z = {tf?.translation.z}");
Console.WriteLine($"tf.Origin.X = {tf?.origin.x}");
Console.WriteLine($"tf.Origin.Y = {tf?.origin.y}");
Console.WriteLine($"tf.Origin.Z = {tf?.origin.z}");
Console.WriteLine($"tf.Rotation.X = {tf?.rotation.x}");
Console.WriteLine($"tf.Rotation.Y = {tf?.rotation.y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.rotation.z}");
}
}
}
using System.Text.Json;
namespace CodeFromJson
{
public class Origin
{
public double x { get; set; }
public int y { get; set; }
public double z { get; set; }
}
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Rotation
{
public int x { get; set; }
public int y { get; set; }
public int z { get; set; }
}
public class Translation
{
public double x { get; set; }
public double y { get; set; }
public int z { get; set; }
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string? jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<Root>(jsonString);
Console.WriteLine($"tf.Translation.X = {tf.translation.x}");
Console.WriteLine($"tf.Translation.Y = {tf?.translation.y}");
Console.WriteLine($"tf.Translation.Z = {tf?.translation.z}");
Console.WriteLine($"tf.Origin.X = {tf?.origin.x}");
Console.WriteLine($"tf.Origin.Y = {tf?.origin.y}");
Console.WriteLine($"tf.Origin.Z = {tf?.origin.z}");
Console.WriteLine($"tf.Rotation.X = {tf?.rotation.x}");
Console.WriteLine($"tf.Rotation.Y = {tf?.rotation.y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.rotation.z}");
}
}
}
translation, origin and rotation are vectors
no, they are not
they are just kvp in a json
they are numbers
that you want to create a vector from those 3 numbers is another story
I only need to output all numbers as float
then change the type
to float
using System.Text.Json;
namespace CodeFromJson
{
public class Origin
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Rotation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Translation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string? jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<Root>(jsonString);
Console.WriteLine($"tf.Translation.X = {tf.translation.x}");
Console.WriteLine($"tf.Translation.Y = {tf?.translation.y}");
Console.WriteLine($"tf.Translation.Z = {tf?.translation.z}");
Console.WriteLine($"tf.Origin.X = {tf?.origin.x}");
Console.WriteLine($"tf.Origin.Y = {tf?.origin.y}");
Console.WriteLine($"tf.Origin.Z = {tf?.origin.z}");
Console.WriteLine($"tf.Rotation.X = {tf?.rotation.x}");
Console.WriteLine($"tf.Rotation.Y = {tf?.rotation.y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.rotation.z}");
}
}
}
using System.Text.Json;
namespace CodeFromJson
{
public class Origin
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Rotation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Translation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string? jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<Root>(jsonString);
Console.WriteLine($"tf.Translation.X = {tf.translation.x}");
Console.WriteLine($"tf.Translation.Y = {tf?.translation.y}");
Console.WriteLine($"tf.Translation.Z = {tf?.translation.z}");
Console.WriteLine($"tf.Origin.X = {tf?.origin.x}");
Console.WriteLine($"tf.Origin.Y = {tf?.origin.y}");
Console.WriteLine($"tf.Origin.Z = {tf?.origin.z}");
Console.WriteLine($"tf.Rotation.X = {tf?.rotation.x}");
Console.WriteLine($"tf.Rotation.Y = {tf?.rotation.y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.rotation.z}");
}
}
}
I input valid json but it gives null for something
I forgot to put null check at translation
And still no success
It outputs all values as null
show code
using System.Text.Json;
namespace CodeFromJson
{
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Origin
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Rotation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Translation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string? jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<Root>(jsonString!);
Console.WriteLine($"tf.Translation.X = {tf?.translation?.x}");
Console.WriteLine($"tf.Translation.Y = {tf?.translation?.y}");
Console.WriteLine($"tf.Translation.Z = {tf?.translation?.z}");
Console.WriteLine($"tf.Origin.X = {tf?.origin?.z}");
Console.WriteLine($"tf.Origin.Y = {tf?.origin?.y}");
Console.WriteLine($"tf.Origin.Z = {tf?.origin?.z}");
Console.WriteLine($"tf.Rotation.X = {tf?.rotation.x}");
Console.WriteLine($"tf.Rotation.Y = {tf?.rotation?.y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.rotation?.z}");
}
}
}
using System.Text.Json;
namespace CodeFromJson
{
public class Root
{
public Translation translation { get; set; }
public Rotation rotation { get; set; }
public Origin origin { get; set; }
}
public class Origin
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Rotation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public class Translation
{
public float x { get; set; }
public float y { get; set; }
public float z { get; set; }
}
public static class CodeFromJson
{
public static void Main(string[] args)
{
Console.WriteLine("JSON Input:");
string? jsonString = Console.ReadLine();
var tf = JsonSerializer.Deserialize<Root>(jsonString!);
Console.WriteLine($"tf.Translation.X = {tf?.translation?.x}");
Console.WriteLine($"tf.Translation.Y = {tf?.translation?.y}");
Console.WriteLine($"tf.Translation.Z = {tf?.translation?.z}");
Console.WriteLine($"tf.Origin.X = {tf?.origin?.z}");
Console.WriteLine($"tf.Origin.Y = {tf?.origin?.y}");
Console.WriteLine($"tf.Origin.Z = {tf?.origin?.z}");
Console.WriteLine($"tf.Rotation.X = {tf?.rotation.x}");
Console.WriteLine($"tf.Rotation.Y = {tf?.rotation?.y}");
Console.WriteLine($"tf.Rotation.Z = {tf?.rotation?.z}");
}
}
}
works for me
pass this json
{"translation": {"x": -0.15,"y": -0.22,"z": 0},"rotation": {"x": 0,"y": 45,"z": 0},"origin": {"x": 0.8,"y": 0,"z": 0.5}}
{"translation": {"x": -0.15,"y": -0.22,"z": 0},"rotation": {"x": 0,"y": 45,"z": 0},"origin": {"x": 0.8,"y": 0,"z": 0.5}}
Yeah it works
Thank you ❤️

I hope I make it as VSCode extension someday