❔ How Would I extract a single byte from an int
In my project, I have information which is effectively a byte[4] stored in an int32, I want to grab a specific byte from this
I currently have the code
this produces an error as it should, but how would I do this properly
16 Replies
just realised after sending that I could left shift it before right shifting it, so that all other values are overflown and elimenated
is that the best way? or is there a better?
is what I have changed it to, but it seems a bit verbose with too many brackets
also is "bitfucking" a word which people use? I have been using it a bunch with my friend when we are clueless about bit shifting, but I googled it and couldn't find anyone else using it, have we made it up?
it is the verb form of "bitfuckery"
there are a few ways you could do this
or with this class but then you are allocating memory which is maybe not prefered
https://learn.microsoft.com/en-us/dotnet/api/system.bitconverter.getbytes?view=net-7.0#system-bitconverter-getbytes(system-int32)
BitConverter.GetBytes Method (System)
Converts the specified data to an array of bytes.
more complicated bitfuckery
https://graphics.stanford.edu/~seander/bithacks.html
@Sherbert Lemon does this help?
does the first one work? how does it just work with an and?
but yeah defo not the getbytes, the whole point of the bitfuckery was to avoid a few hundred to a few thousand arrays needlessly
oh yeah ok i get it now
i'm not very good at bitfuckery, i partially did this to get better at it
the & byte.MaxValue is so only the 8 bits stay and the other turn 0
yep that is quite a nice concise way of doing it thanks
how does the pointer one work then, I will defo just use the first thing but that intrigues me
with pointers you can change the type of a thing (which is dangerous to do ofc) so if the compiler thinks it points to a byte array instead of an int. an int is 4 bytes so you can use it like its a byte array of 4 bytes instead
ah ok that is nice, not a very c# way of doing it tho lol, and this is in unity so it is a bit of a pain to enable unsafe code
thanks though, I will defo have a read through the bitfuckery page, he calls it bittwiddling, is that the more normal way of calling it?
idk
what do you call it?
id call it bitfuckery
dont do this pointerfuck though
(i just want to show it bcuz i think its a bit crazy)
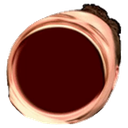
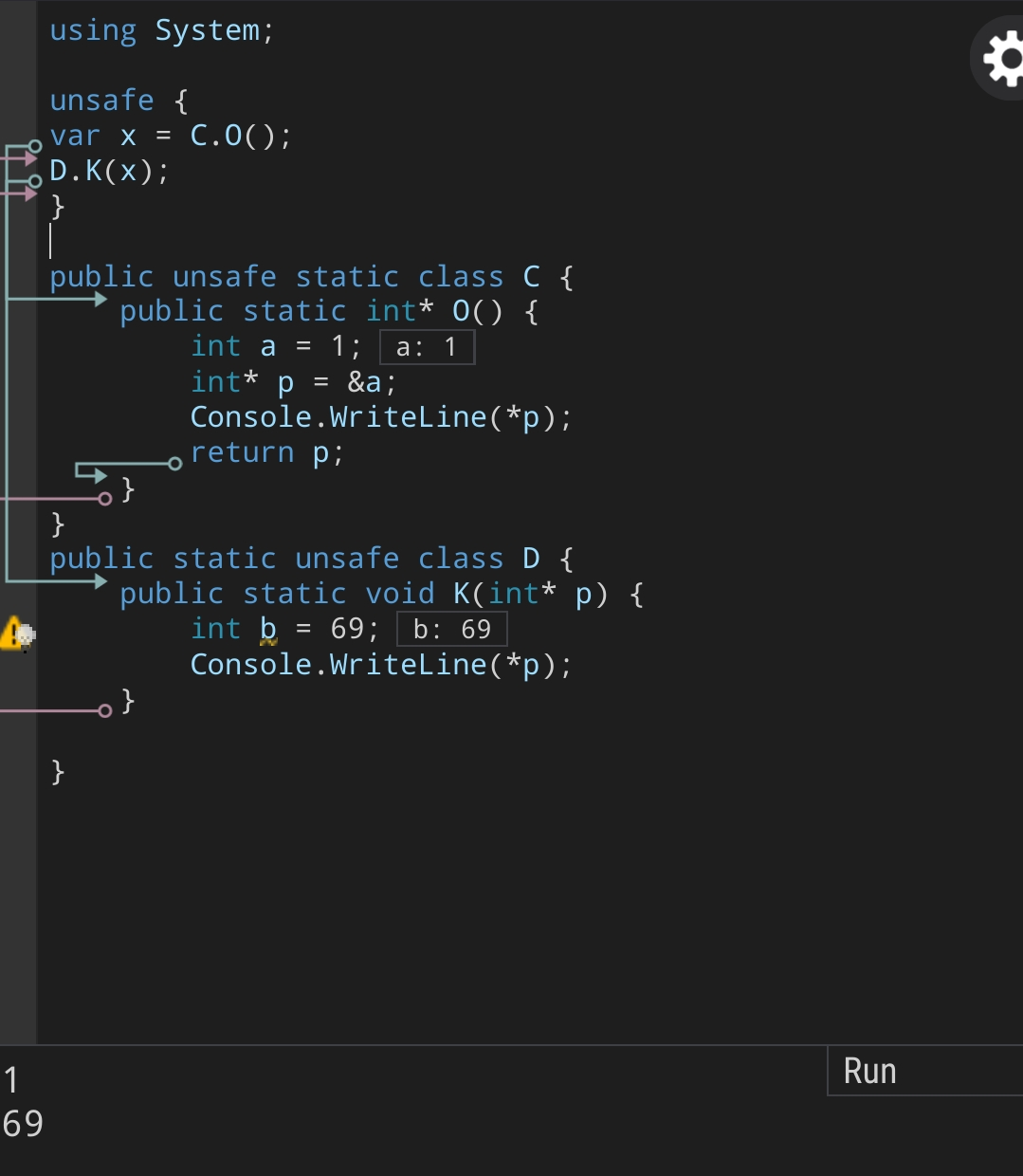
pointers are dangerous
so yeah go with the safe solution
lol what, that took me a good minute to clock what it was doing, weird ig you have to pin it but is a bit of a pain to do, i'll leave pointerfuckery to cpp
that is good though, I can blame you if I ever call it bitfuckery in class and say that is what everyone calls it. At least whenever we finally get to that topic lol, the computer science curriculum moves so slowly in the uk
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.