Update JSON File with new values without overwriting existing data
Hello,
So I'm currently developing a Discord bot that stores values such as 'settings' for each guild. I already got that part figured out very well but I would like to know how I should handle additions / removes.
I have a class as followed:
As an example, currently, the json file doesn't have the
public bool EnableModeratorNotifications
included but it is a new feature I would like to add. If I add that to my class, how would I serialize it into the json file with a default value like true/false without overwriting the existing values there?46 Replies
are you deserializing the JSON into an instance of that before writing it back out?
Yes.
I think system.text.json will just use the default value then
(btw, you don't need
!
on that bool, that's only for nullable ref types)ah okay
also, if you want those props to always be set, you might want instead
What does 'required' specifically do?
the compiler yells at you if you try to create an instance without setting that prop
jcotton42#1663
REPL Result: Failure
Exception: CompilationErrorException
Compile: 489.636ms | Execution: 0.000ms | React with ❌ to remove this embed.
ah okay makes sense
jcotton42#1663
REPL Result: Success
Compile: 553.507ms | Execution: 56.267ms | React with ❌ to remove this embed.
So, I heard about
[JsonProperty(DefaultValueHandling = DefaultValueHandling.Populate)]
and DefaultValue()
would that do something?
or could I basically do public string GuildPrefix { get; set; } = "Value Here";
that looks like Newtonsoft?
are you using newtonsoft, or system.text.json?
I think it is Newtonsoft if I am not mistaken
Yep, it's Newtonsoft.Json;
I'm not familiar with that part of it
I always use STJ
Is there a benefit of using STJ over Newtonsoft?
faster, lower memory usage, etc.
Newtonsoft has more features, but I don't find myself needing them
it's also one less dependency to bundle, if you care about that
oh, i did hear about STJ but I somehow always ended up using Newtonsoft.Json
I will try your advice and check
in any case, here's how STJ works by default
gives
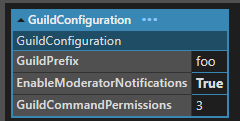
Oh, so it serializes on it's own basically?
err, oops
meant NewtonSoft
pretty sure STJ is the same, lemme try
ohh
oh huh
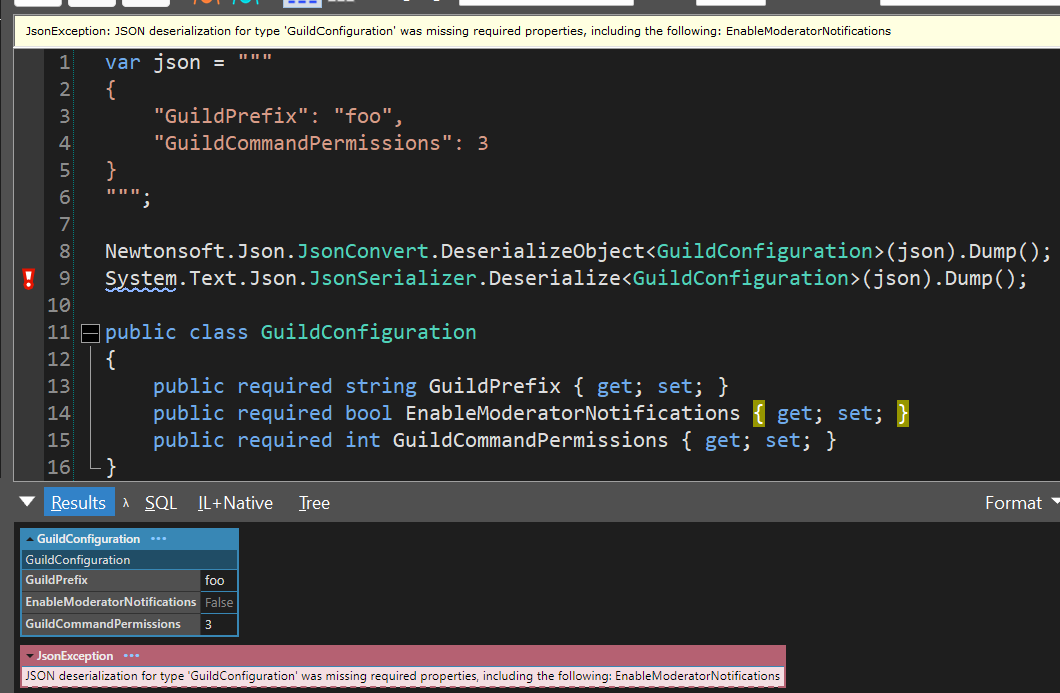
I bet that's configurable...
Hm I see
https://learn.microsoft.com/en-us/dotnet/standard/serialization/system-text-json/required-properties
Require properties for deserialization
Learn how to mark properties as required for deserialization to succeed.
yeah that's intended
For some reason, for me
.Dump();
isn't availableah sorry
that's a LINQPad thing
it's writes to the lower pane
oh okay
Instead of a string, is it possible to deserialize the object using a path to the json file?
Because if so, I would simply add it to my loop when checking if each guild has the appropriate json file
if it exists, simply update it
STJ can take a stream to deserialize
it looks like NewtonSoft can't, which is surprising
so you'd need to read the file into memory as a string
then deserialize
So like this,
yeah
I did but for some reason the new value wasn't added to the json
well, you're only deserializing
you're not serializing back to it
(also, if you're not that far in, you might want to consider using a database; managing tons of json files will get hairy pretty fast)
oop i was told that json can be pretty tough
but i get it, im deserializing it but i have to serialize it back
it's not "json is tough" it's "when you have a ton of files spread about you're poorly approximating a database"
I would use a database since I have worked with MySQL before a lot
for a bot I'd use something like SQLite or PostgreSQL
(I have... opinions about MySQL, tbh)
EF Core makes interfacing with the db pretty easy
The bot would handle a lot of cases at once and im not sure how the performance would be for the bot
like, i once added a leveling system which means everytime a user types a message, it would change his score in the json which worked fine
databases are obscenely fast
even sqlite
Would that mean I have to keep the connection to the database open 24/7 once it's open
hm i see
if you use EF it manages the connection for you
I haven't done it manually in a long while
i haven't worked with a DB in awhile yeah
I still can't seem to figure out how to update the json file
Setting a default value in the designer class works fine but actually adding that value into the json file doesn'twork :/
Nevermind, it worked using this code:
I mean this looks kinda weird but it works?
I mean
how else would you expect it to work?
you deserialize to an object
update the object
serialize back
Well, tbh, this looks like sloppy work.
I usually look at the code in a more clean way but this will do.