Using default values from a JSON file
I have this issue on the live version:
https://cozynova.online/mvptimer/
I would like to use the default values from a JSON file if the user inputs nothing, at the moment I assume values of true/false, but I feel like I'm not doing it correctly.
tl;dr
1. If user inputs nothing -> use CDR values
2. If user inputs something -> use their values (already working)
MVP Timer
Looking to set up a timer for an MVP?
3 Replies
This is the code snippet handling this:
And this is an example from the JSON file:
I commented the conditions, but basically right now it doesn't admit any because it's defaulted to
00:00:00
(or it assumes this time, basically).
I'm still beginner in JS 😔If the user inputs nothing at all you want to use the default values? Or do you want the user's input to overwrite the defaults? Both are pretty easy to do. If you want to check for any user input then you're on the right track, though I would do it a bit differently.
for the latter (which is what your initial post indicates) then you do what you're doing: check for user input and if there is any, use it. Otherwise use the default. To get the default you'll either have to read the JSON and parse it (using
JSON.parse()
) or hardcode it into the JS file as an object (either in the main script or import it from another file).
If you want to set the defaults and have any user input overwrite it, then I'll teach you something called Object.assign()
. It's pretty easy: you give it an object to write to and any number of additional object to copy from. Objects are copied from left to right, so any properties in later objects overwrite earlier object.
Normally the first parameter is an empty object, so all copying happen to the new object and you don't accidentally overwrite an existing object.
So you do something like:
This makes an empty object. Then it copies the defaults. After that, any properties from the user input are copied over, overwriting any defaults. And if there is no user input then only the default object is used.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign
Hope that helps!
You'll have to validate the user input, of course! You don't want to overwrite defaults with empty strings or a bunch of NaN
s :pIt turns out I'm an idiot...
I didn't have an Object per se, but something quite similar, but the issue was not even THE FUNCTION ITSELF.
It was this function inputting default values. So no matter how I defined it, whether the user has input something or not, by default it was assuming the values from the HTML
I cannot even describe the frustration of how silly this was.
I updated it to actually do it only if the user "fucks up".
I don't know whether should I cry or not.
Actually, I'm still troubled with some of the logic Q_Q
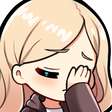