avoid rendering more hooks: running trpc query based on supabase session's user
i have a router
workspace
with query getWorkspace
that takes the current supabase session's user's UUID id and returns details of the workspace they're a member of
but on my /pages/today.tsx
where i need that workspace info, it's throwing Unhandled Runtime Error
Error: Rendered more hooks than during the previous render
22 Replies
Instead, set disabled to true or false
Don't do hooks optionally, never.
sorry, what should i be disabling?
useQuery hook takes options, one of those are called
disabled
, takes in true or false
Oh wait no it's called enabled
lolaight, i modified it to this but since supabase's type im importing for
User
can also be undefined, it's giving me that message. which is why i had the condition before. is there a better way to handle this?
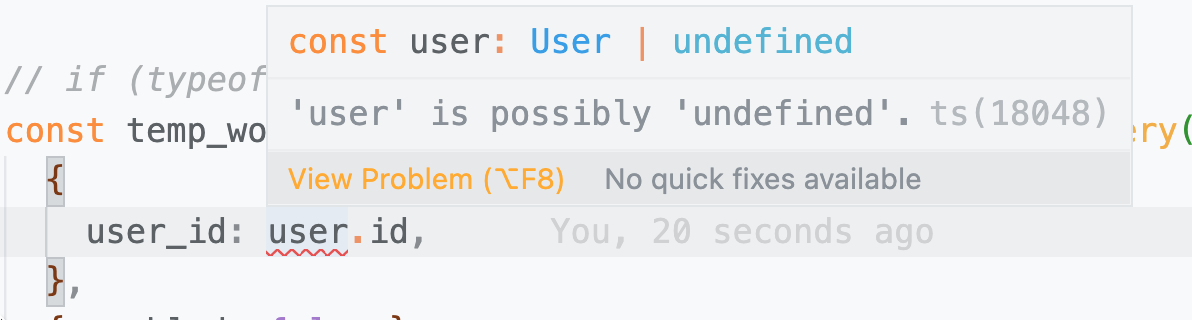
Idk how Supabase's promises work but I'm assuming this would work
Or asserting that
typeof user.id === string
should work toohmm that won't get around the typeerror, since the user state is undefined until the
getUser
runs in useEffect
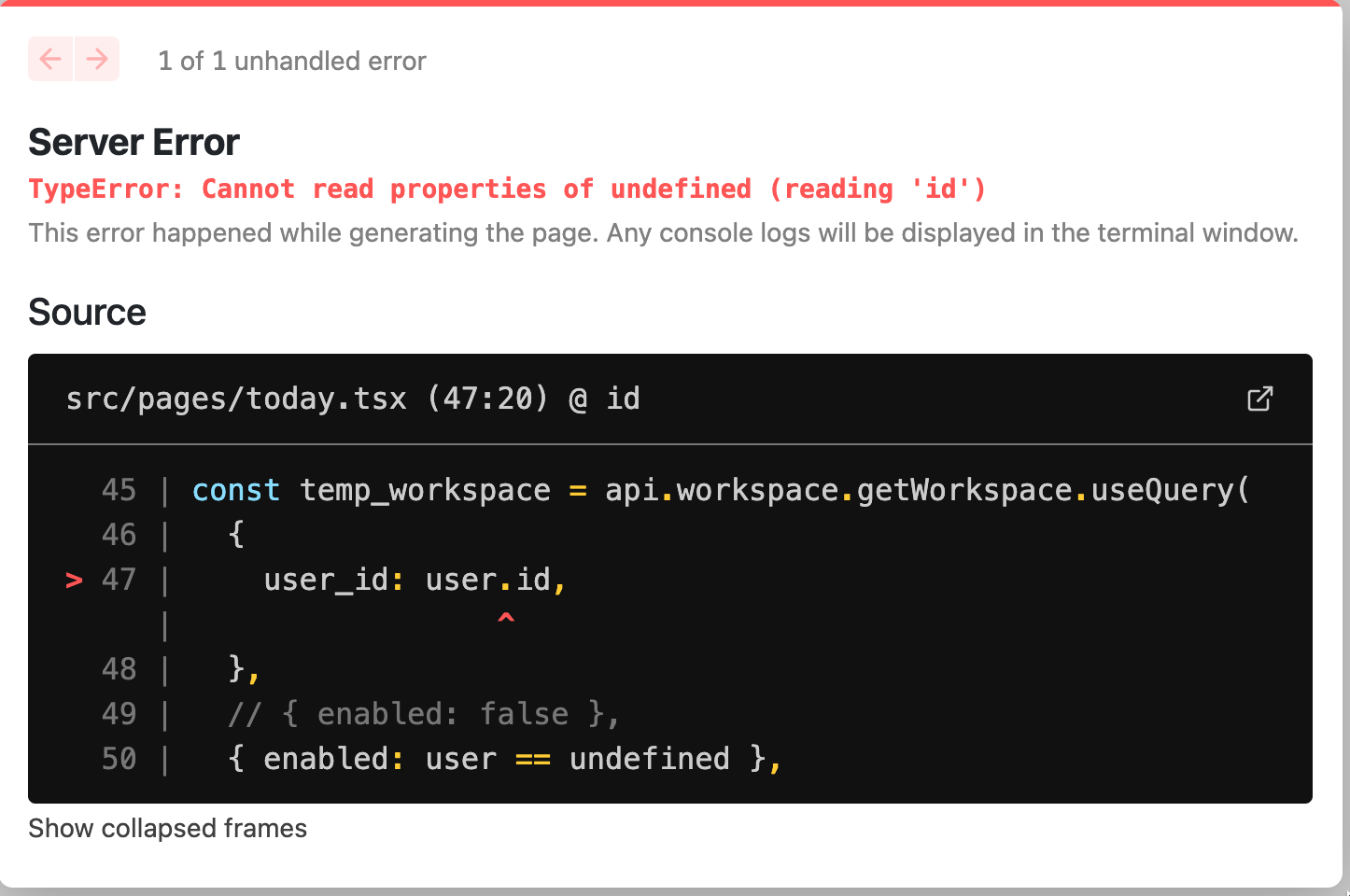
NOT equal undefined
The not is important
whoops i forgot to change it back
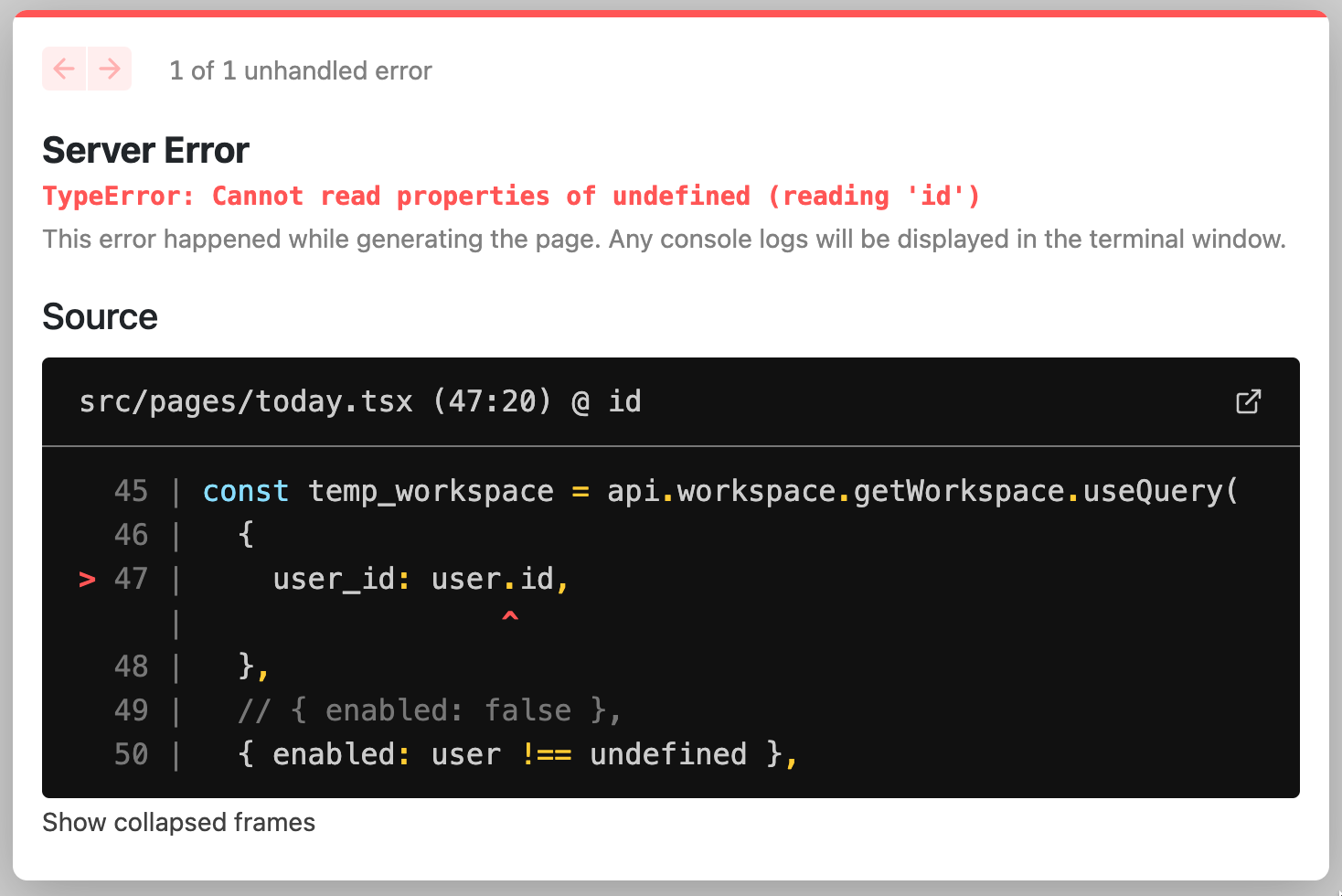
What if you did this
enabled: user && "id" in user
same issue, does order matter for useQuery's args or something?
nvm def not order
I guess you can just do optional chaining
how would i go back to that and avoid the error we started with 😦
Unhandled Runtime Error
Error: Rendered more hooks than during the previous render.
Just do
user?.id
?oh i misunderstood, let me try that
that means i'll have to allow
user_id: z.union([z.string(), z.undefined()]),
in the trpc router's input rightDon't think so
mainly because of this
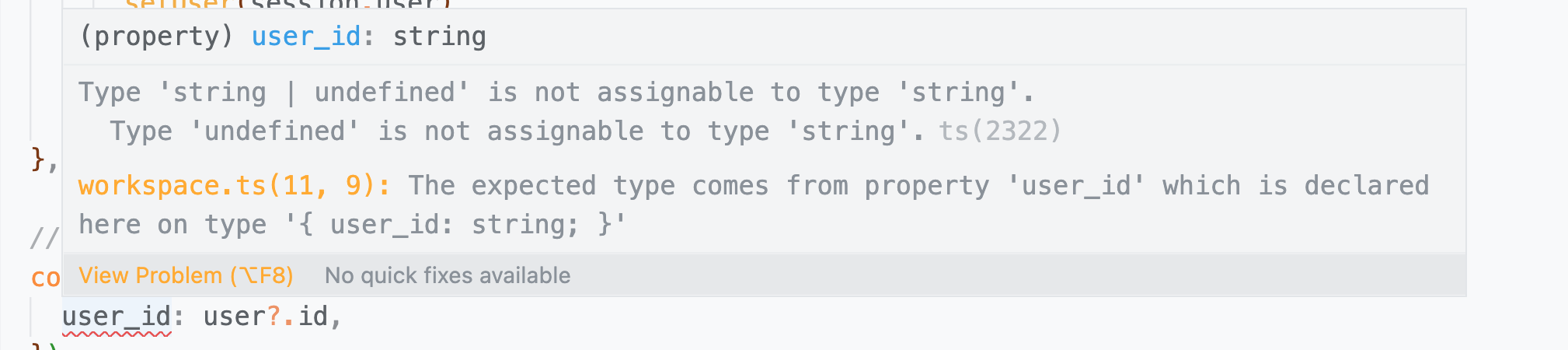
you're also using a
useEffect
remove getUser
from the useEffect, and if you really want to, put it on a useCallBack
the useEffect probably is the one that's running too many timesjust clarifying, remove getUser's implementation and leave the call in the useEffect? or remove the useEffect and its contents all together?
from the docs it looks like you're trying to redo the supabase
useUser
hook?
source: https://supabase.com/docs/guides/auth/auth-helpers/nextjs
Supabase Auth with Next.js | Supabase Docs
Authentication helpers for Next.js API routes, middleware, and SSR.
it could get reduced to that
appreciate this! been trying to debug this but
causes Unhandled Runtime Error
TypeError: Cannot destructure property 'user' of '(0 , _supabase_auth_helpers_reactWEBPACK_IMPORTED_MODULE_1.useUser)(...)' as it is null.
and if i just do , i'll get
useUser: null
in console
and as a failsafe, i brought back the useEffect with getUser()
and it would correctly show my user info since im signed in
/src/pages/today.tsx
and src/pages/_app.tsx
You know you can just call create client outside of a component
No usestate hacks