✅ jwt authentication always returns 401
I'm trying to build a backend with .net & firebase, but I can not get over one specific problem. When I try to implement jwt, calling any endpoint just throws 401 error code (after logging in in swagger ofc). What am I doing wrong?
24 Replies
Startup.cs:
FirebaseAuthenticationClient.cs:
appsettings.Production.json:
Dependencies:
- FirebaseAdmin (2.3.0)
- Microsoft.AspNet.WebApi.Client (5.2.9)
- Microsoft.AspNetCore.Authentication.JwtBearer (7.0.4)
- Microsoft.AspNetCore.OpenApi (7.0.3)
- Swashbuckle.AspNetCore (6.4.0)
So
Login
endpoint returns a valid JWT but subsequent requests return 401?
Are you sure JWT is getting sent with your subsequent requests?Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.hi, sorry for revinving this
but i'm with the same problem (i didn't want to polute and create a new Post)
What's the problem again 😅 ?
fine
basically
i did an endpoint to create the JWT
at the end it returns me a ok look like JWT
but when i use it, it returns 401 Unauthourized
and
Bearer error="invalid_token", error_description="The signature key was not found"
Okay, first what have you added a reference to
Microsoft.AspNetCore.Authentication.JwtBearer
yes
mine ones
that's basically the function that creates my JWT's
How did you add your config the auth services ?
wdym?
i mean, i did just make a simple, Login endpoint, that creates a JWT that allows you to access the other endpoints
btw i'm using .NET 6
not fast api, just a common webapi
Sorry, what I meant is how did add your auth servics, like
.AddJwtBearer
and .AddAuthentication
No like, show the AddJwtBearer and AddAuthentication calls
my whole method that creates the JWT is that>
You aren't calling
AddJwtBearer
at all?hmm... no ?
i was just following a tutorial
(and in the tutorial did work so i thought it would be something wrong in my code)
It won't work without adding JWT auth services, so the tutorial should have that.
where can i insert then?
Do you have startup.cs file?
nop
.net 6 doesn't have one, it's the Program.cs, but works almost the same way i think
Yeah, it's the same, you have to add couples of things
1 - The middleware for authentication and authorization
2 - Add authentication services by calling
AddAuthentication
and then adding AddJwtBearer
i did put both, but i didn't find about how to add the middleware in .NET 6 without using the Startup.cs
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.update> i actually just get a read on a tutorial and find out how to make it work, and worked, thanks
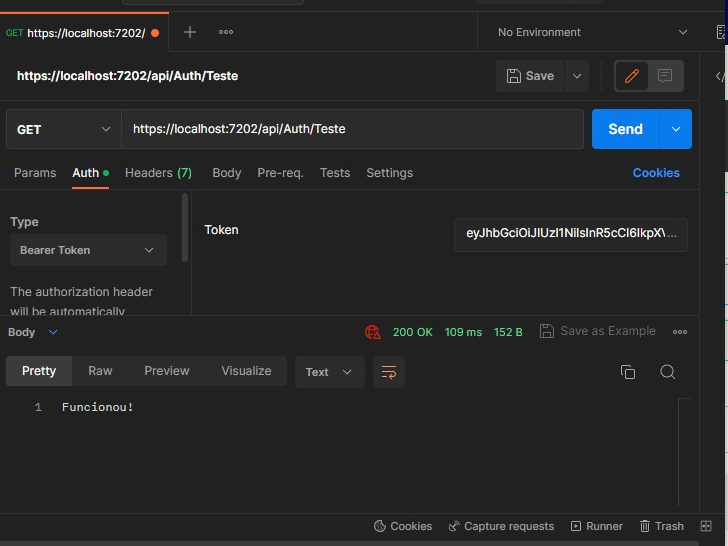