✅ Interesting uses of yield return
I recently needed to generate an arbitrary number of unique random numbers, and after a little thinking I found I could get a really elegant solution by using
yield return
to generate an infinite stream of ints. This made me realise I basically never use this language feature and there's probably a lot of cool applications for it I'm missing. Any tricks/interesting patterns you've written with it?16 Replies
for anyone interested, what I did was this:
(basically just)
yield return is indeed very useful
I'd say it's rarely used in the way they're using it here
damn, I should have thought of that!
What they're most useful for is probably returning collections of things where each item is potentially computationally intensive and where it's useful to let the caller decide how many items it needs.
It's like a task in the sense that the caller can decide to interrupt the enumeration (and therefore execution of the method) at any time.
(hell, Unity uses
yield
for its async stuff)this can give you fewer numbers than amount
I suppose there's no built-in infinite range in linq
it's not true async btw 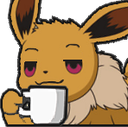
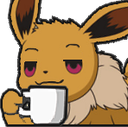
Yeah I noticed as soon as I posted. Would this be a worthwhile proposal?
what do you mean?
Propose an infinite range in Linq to dotnet/runtime
I guess it's no different than introducing
ISystemClock
or similar helpers that you can code in 3 linesWhy do you need an infinite range thing? If you keep asking OP's
GetRandom
for the next value, it will keep giving you one -- an infinite stream of random values. No need to generate an infinite range and then map each element to a random value, or somethingi will note one little discrepancy in my original code i'm curious about
if the amount you 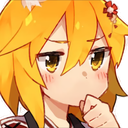
.Take()
is greater than max
, the program will deadlock
eventually i figured out this was because it's obviously impossible to have, say, 50 unique numbers less than 34
but i was surprised i didn't get an exception anywhere
i don't know if there's an intelligent way to order the LINQ methods so that kind of subtle bug is more obvious 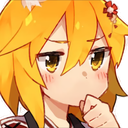
Hmm, I don't think so. The knowledge required to detect that problem is kinda spread out over the whole pipeline.
RIP