tRPC API endpoints?
Hello guys, i've been asked to implement an api route to create, validate and edit password using post methods, I'm allowed to use t3 and provide a better implementation with it...
I'm new to it and i'm trying to wrap my mind around trpc, i have been able to do queries and mutations using it from the front end. but I'm not sure if it's possible to use it in an api endpoint ex: /api/user/create, sending a req.body instead of "input"?
sorry im a bit lost
12 Replies
GitHub
GitHub - jlalmes/trpc-openapi: OpenAPI support for tRPC 🧩
OpenAPI support for tRPC 🧩. Contribute to jlalmes/trpc-openapi development by creating an account on GitHub.
you should not call trpc endpoint directly, calling from a valid client will always better as you can infer input/output
if you really want to call it from a external source, use the openapi plugin
thanks, i've done something at it's "working"
import {
createTRPCRouter,
protectedProcedure,
publicProcedure,
} from "~/server/api/trpc";
import bcryptjs from "bcryptjs";
import { z } from "zod";
export const userRouter = createTRPCRouter({
create: publicProcedure
.meta({ openapi: { method: "POST", path: "/test/create" } })
.input(
z.object({
rut: z.string(),
name: z.string(),
paternallastname: z.string(),
maternallastname: z.string(),
email: z.string(),
phone: z.string(),
address: z.string(),
district: z.string(),
password: z.string(),
})
)
.output(
z.object({
message: z.string(),
person: z.object({
id: z.number(),
rut: z.string(),
name: z.string(),
paternallastname: z.string(),
maternallastname: z.string(),
email: z.string(),
phone: z.string(),
address: z.string(),
district: z.string(),
}),
user: z.object({
id: z.number(),
person_id: z.number(),
hash: z.string(),
}),
})
)
.query(async ({ ctx, input }) => {
const newPerson = await ctx.prisma.person.create({
data: {
rut: input.rut,
name: input.name,
paternallastname: input.paternallastname,
maternallastname: input.maternallastname,
email: input.email,
phone: input.phone,
address: input.address,
district: input.district,
},
});
const hashedPassword = bcryptjs.hashSync(input.password, 10);
const newUser = await ctx.prisma.user.create({
data: {
person_id: newPerson.id,
hash: hashedPassword,
},
});
return {
message: "User created",
person: newPerson,
user: newUser,
};
}),
});
im using that and its creating a user with when posting to /api/test/create, but i'm getting this a response
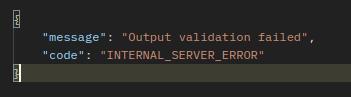
even though its succesfully posting
unless you want to constrain the output type
you dont need to define it


i get this when not passing .output()
open-api trpc requires you to do an output iirc, it's also used to generate the open-api docs 🙂
i understand, but why getting this when i define the output? i dont get where that is coming from
also quick note, POST probably should be a
mutation
instead of a query
, since it's mutating something on the serveryou are right, fixed that