❔ Hello I am new to C#, I dont understand this error:
I have a insert method that adds to queue:
and then in a thread loop:
but I'm getting this error:
35 Replies
So im trying to cast items as a list but I can't figure it out
Ok this seem to work:
is that the right way?
What is your
_insertQueue
declared as?
Never use ArrayList
private Queue _insertQueue = new Queue();
Error: Specified cast is not valid.
yeah its an untyped queue
dont cast to arraylist
you need to use the generic version
Queue<object[]>

Not letting me
ok
you need to update your imports
^
private Queue<object[]> _insertQueue = new Queue<string, string, BsonDocument>();
I added the import
wrong type argument
should be object[] right
you can't have type argument mismatches
also, I dont think queue takes 3 type arguments
which is why Diddy suggested a tuple
(T1, T2, T3)
or a custom classprivate Queue<(string, string, BsonDocument)> _insertQueue = new Queue<(string, string, BsonDocument)>();
this makes sense?
sure
I'd probably use a
record
myself, but this is fineand then :
yeah, seems okay at a glance
this
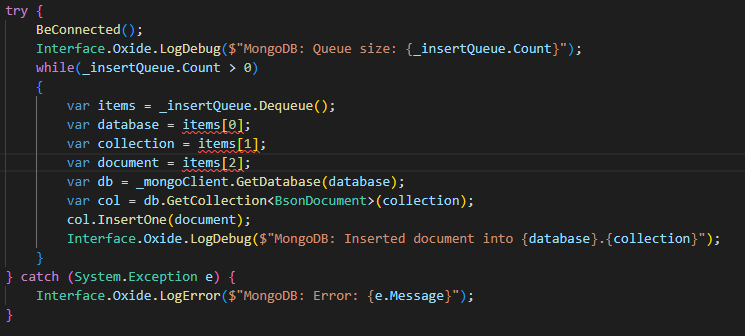
:/
you can't access it with an index accesser
unpack the tuple
var (database, collection, document) = _insertQueue.Dequeue(); ?
var database, collection, document = items
or inline thatNot used to all these types, I come from python :x
same, but a long time ago
time to unlearn your bad habits 🙂
I kinda like it tho, i feel like it makes things more clear
or forces you to write clean code
it is definitely safer (harder to write bugs)
the good thing with types is that after some time, you revisit this code, you don't need to go "uhh, what the hell do I actually write to that queue.. let me look"
you can just look at the type declaration
oh, its a (string database, string collection, BsonDocument document)
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
No clue i just started using mongo and csharp
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Yeah i should grab the database and the collection before the loop obviously
This is pure testing at this point
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Thanks for the help guys!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.