❔ Need help with Node LinkedLists
This is my first time using non built-in Lists so im a bit lost here;
This is the task:
Make a list for each agent month (keypad entry) portable subscription list (subscriber's address, surname,
telephone number, start of period, length of period, publication code, number of publications) and count
the total number of publications
Make a list of the agents who carry more than the average quantity for the given month.
Having troubles with printing out the amount of subscribers each agent has assigned to them, any help would be appreciated :D
These are the subscribers:
123 Main Street, Vilnius, Jonaitis, +370 1234567, 3, 6, LT123, 12, AG-001
456 Oak Street, Kaunas, Petrauskas, +370 7654321, 1, 10, LT456, 4, AG-001
789 Pine Street, Klaipeda, Kazlauskas, +370 9876543, 5, 7, LT789, 6, AG-002
321 Cherry Street, Šiauliai, Petrauskas, +370 4567890, 4, 4, LT111, 3, AG-003
987 Cedar Street, Alytus, Jonaitis, +370 2345678, 2, 8, LT222, 8, AG-004
234 Birch Street, Panevėžys, Kazlauskas, +370 8765432, 7, 1, LT333, 1, AG-002
876 Elm Street, Utena, Petrauskas, +370 5432109, 6, 3, LT444, 5, AG-003
543 Maple Street, Marijampolė, Jonaitis, +370 2109876, 1, 11, LT555, 9, AG-004
210 Walnut Street, Tauragė, Kazlauskas, +370 6789012, 9, 2, LT666, 7, AG-002
678 Pineapple Street, Druskininkai, Jonaitis, +370 3456789, 8, 5, LT777, 3, AG-003
And these are the Agents:
AG-001, Petrauskas, Antanas, 456 Oak Street, Kaunas, +370 7654321
AG-002, Kazlauskas, Andrius, 789 Pine Street, Klaipeda, +370 9876543
AG-003, Jonaitis, Jonas, 321 Cherry Street, Šiauliai, +370 4567890
AG-004, Petrauskas, Petras, 987 Cedar Street, Alytus, +370 2345678
10 Replies
what have you tried so far?
public static void PrintAgentsWithSubscribers(LLAgent agents, LLSubscriber subscribers)
{
Node<Agent> agentNode = agents.Head;
while (agentNode != null)
{
Console.WriteLine("Agent: {0} {1}", agentNode.Data.Surname, agentNode.Data.Name);
Console.WriteLine("Assigned Subscribers:");
Node<Subscriber> subscriberNode = subscribers.Head;
while (subscriberNode != null)
{
if (subscriberNode.Data.AgentCode == agentNode.Data.Code)
{
Console.WriteLine("{0} {1}", subscriberNode.Data.Surname, subscriberNode.Data.Name);
}
subscriberNode = subscriberNode.Next;
}
Console.WriteLine();
agentNode = agentNode.Next;
}
}
Something along the lines of this was my first thought, but .Head is private and im not so sure i can change it to public
you'll need some way to access the first element in your list, if you can't make the head field public there are other ways you can expose it (a property)
Can i do something like this to get it ?
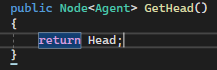
you can but that's not generally how it's done in C#
C# has properties that let you do the same thing more concisely
for example
by the way, PascalCase tends to be for publicly accessible members and not private data so i wouldn't personally name the property like that
you could remove the field altogether and do something like
Properties - C# Programming Guide
A property in C# is a member that uses accessor methods to read, write, or compute the value of a private field as if it were a public data member.
So something along the lines of this should work now
give it a try
in the future you should consider always using properties instead of fields to prevent outside code from changing your objects in ways you might not expect
Will try that, cheers for the help
Looks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.