❔ Looking to turn this GUI integer calculator into a Fraction calculator using the provided class
Final project for school, need to create a GUI fraction calculator. I have a GUI integer calculator already built, and a Fraction class already built. Currently stuck on where to even begin to have the GUI calculate fractions vs. integers.
GUI Code Attached
Fraction Class
63 Replies
what are you trying to make it look like?
this is the current build, and it has to stay in this look per the assignment. I have the slash button in the bottom left to enter the "/" between a numerator and a denominator
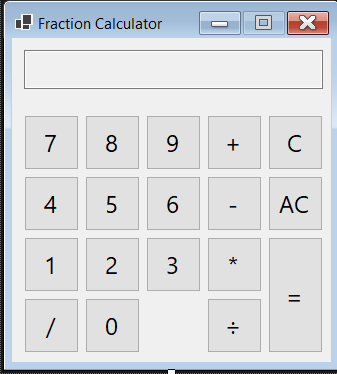
so when you do something like
7 / 3
is it just supposed to display like that?correct
and it does not need to be reduced
I would start with buttonSlash_Click
which looks like it's setting the display to just "/"
and currentValue to 0, which seems undesirable
what should I change it to?
currentValue can't just be an int, because now you're working with more complex things.
You don't want to reset the value to 0, you want to start tracking a fraction where the current value is the numerator (assuming a user typeing
3/4/5
is the same as (3/4)/5
)
and then textBoxDisplay likely should be currentValue + "/"
That should get you started in the right directionso should i change the DISPLAY_MODE to accumulator rather than current_value?
that's up to you. What would accumulator be in that case?
it seems to only matter if
UpdateDisplay
is calledi got rid of currentValue = 0, but the "/" is still deleted when i try to enter the second number
that makes sense
are you using the Fraction class at all yet?
and if not, why not?
no, and i dont know where to implement it. thats really what im stuck on at this point; where do i start using the Fraction class
GUI's throw me off incredibly. I've done great with console programs
that's fine, just think of the textbox as what you would right to the console. It's a single string you want to display
the difference here is in a console you'd have to parse "3/4" and here you're constructing it. When you the second number, what's happening? NumberKeyHit is getting called correct?
so from there, NumberKeyHit is assuming you're taking the currentValue, multiplying by 10, and then adding the number that was hit. With fraction that's an incorrect assumption
ahhh ok
so in NumberHitKey, i should start implementing the Fraction class?
like currentValue = Fraction.numerator + "/" + Fraction.denominator?
you already implemented the fraction class. You just have a few ways of solving this. The main point is that NumberKeyHit has to know if you're currently doing a fraction or an integer
So you need to store the Fraction, and create it at some point. When do you think you should do that?
as im clicking the buttons?
which buttons?
number keys
so number keys needs to check if you already have a fraction, if you need to create one, or if you're working with an integer
sounds like you would want to update NumberKeyHit then, because that gets called when a number key gets hit
ok, would i need to add a new enumeration? something like FRACTION_VALUE or something?
that would work
and frankly i dont even need this to do integers, just fractions
then currentValue should be a fraction shouldn't it? 🙂
ok let me work on this for a sec
could i remove CURRENT_VALUE and ACCUMULATOR entirely, and change them to numerator and denominator?
you could, yes
i dont even know how to implement this now haha
do you have like an example i can see? like i cannot visualize this
I don't have an example offhand. I was just helping you understand the problem. If you're having trouble with the GUi part, maybe try it with just a console app?
Basically each key input would be equivalent to pressing a button. What would you display to the user and how?
it's a bit much, but if the GUI is what is confusing you there's no reason to worry about it until you understand how to store the data as it comes in
that part is where you seem to be getting stuck
yeah all we've done for 16 weeks is console applications
and now the final is a GUI, with no example on how to implement this fraction class into it
do you have a set of tests that would be used to validate the assignment?
other than just running it and seeing if it works, i dont think so
do you have to be able to add fractions? Like
3/4 + 1/4
?yes, the program needs to add, subtract, multiply, and divide fractions
i have a console program that does all of it flawlessly
this is what I would do then
change accumulator and currentValue to Fraction
then the only thing you need to change is:
1. What happens when a user hits a number?
2. What happens when a user hits "/"?
3. Operator likely needs to be unchanged
when a user hits a number, is it a valid fraction? You have Fraction.Parse, which throws if it's not
when a user hits a "/", you know you need one more number before you have a fraction
1 could be ignored, you just need to append the number onto the textbox string. So instead of calling UpdateDisplay everytime you can just do
textBoxDisplay.Text += number
2 would also just add to the textBoxDisplay
string
and then 3 would parse the current string as a fraction. If it fails to parse, display ERROR
that is one of a few ways to solve it. Don't parse the input until you know you're doing an operator
and likely simplifies it, if you have a console app already. It's like the user input "3/4" and then "+" and then "1/4"
Fraction -> Operator -> Fractionalright i changed accumulator and currentvalue to fraction, good so far?

so now, in NumberKeyHit(), currentvalue should be set to? it would still need to be an integer, since the buttons are integers, right?
so i shouldnt change that?
currentValue has to be a Fraction now. You could change so a Fraction doesn't need a denominator, making it an integer
how would that work?
let me take that back, what do you think should happen when you hit a number key?
a number should appear
if you click "1", 1 should appear in the text box
which is the same as
textBoxDisplay.Text += 1
in your programso if i change it to this, i get a ton of errors, so i assume thats wrong
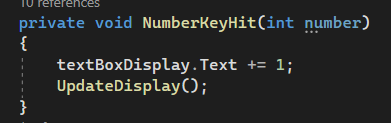
because i have individual methods for each button individually
so then it should be
textBoxDisplay.Text += number
because you passed that into the methodok, now every currentValue object after that method is throwing an error, because they're all set to 0
do i go through and change them all to frac?
if you only need to handle fractions and not integers, then yes
ok, now none of the buttons work lmao
are they showing "0/1" after you hit them?
yes
What does
UpdateDisplay
do to the textbox?
it sets to currentValue.ToString()
right?yes
so you are overwriting your textBox value, which you previously set
so maybe don't call UpdateDisplay 🙂
should i just delete the method all together?
sure
you're taking a different approach than that now
so it's not as needed
might make it simpler for me, if its not there i dont think about it haha
UpdateDisplay isn't a magic function, it only sets the textBox.Text, which is a string
so if it helps to get rid of it, get rid of it
hmm
ok
here's what displays now
actually, the arithmetic doesnt even work anymore
i think that needs to stay
if it needs to stay, then you have to have some other way of getting the numbers into a fraction
the arithmatic is expected to break for now, we're rebuilding the thing from the ground up because I don't know how to help you otherwise. I'm not a teacher 😂. I can help you understand what you're building, or give you the answer, but I can't really help otherwise. Everything in your program is up to you to decide if you want to keep it that way or not
lol ok
i think im just gonna call it for the night, ive been working on this for 8 hours
good luck 🙂
i appreciate your help friend
thanks
I suggest you at least use longs instead of ints. operations on fractions tend to break the int limit
also, you lose information by casting to double in the constructor
ah no sorry, not the constructor, the comparison function
also you should always simplify them if possible
use Euclid's algorithm to find gcd
here's an example I had to make at school https://github.com/AntonC9018/uni_csharp/blob/master/lab2_a/RationalNumber.cs
GitHub
uni_csharp/RationalNumber.cs at master · AntonC9018/uni_csharp
C# from console. Contribute to AntonC9018/uni_csharp development by creating an account on GitHub.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.