Newtonsoft.Json Serialization Exception
Trying to deserialize a List<Highscore> for my project and it throws this exception:
Unhandled exception. Newtonsoft.Json.JsonSerializationException: Error converting value "{"Name":"Willy","Time":316}" to type 'ReactionGame.Highscore'
Code (T is List<Highscore>):
Model:
Anyone know what might be wrong? I'm at a loss.
26 Replies
Ero#1111
REPL Result: Success
Console Output
Compile: 692.423ms | Execution: 158.707ms | React with ❌ to remove this embed.
¯\_(ツ)_/¯
Thanks xD
What did you change exactly?
Nothing
Just showing your that it should work
Bruh 😓
thanks anyways :)
Check your private messages, @𝕭𝖔𝖙𝖙𝖑𝖊_𝕭𝖆𝖗𝖔𝖓.
Ero#1111
REPL Result: Success
Console Output
Compile: 742.622ms | Execution: 179.356ms | React with ❌ to remove this embed.
Still works for a list too
Hm
Might have found it
Seems the json string has been altered somehow
where are you getting this input?
Im sending the data from my game to a web api then using the get method to retrieve it from the site
is the web api strictly a middleman for this or does it do more?
No it is just supposed to store data
then why not just send the data from the game directly to the app?
That is what i am attempting using the post and get endpoints
well no, that's a middleman
this is what the repo looks like roughly
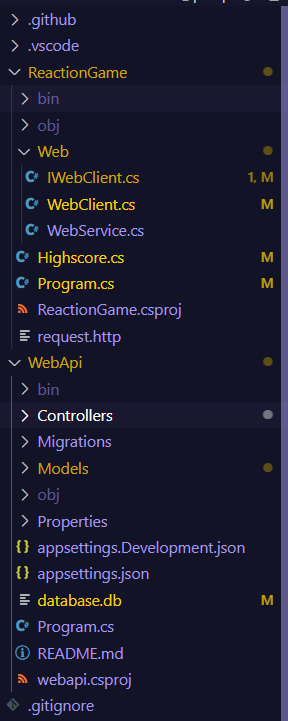
The WebApi parts job is to take the data sent from the reactiongame and store it into a SQLite database
The get method should then get that data and return it to the game
right
well, anyway. that seems like incorrectly formatted json
there shouldn't be quotes after
[
and before ]
ait then i will look into this :)
that looks like a
List<string>
where each string is a json serialized objectyup
also consider using System.Text.Json instead of Newtonsoft
Yeah I'll try that
Thanks ^^
it won't fix this!
but it is still just. better
in every way
Ero#1111
REPL Result: Success
Console Output
Compile: 753.600ms | Execution: 95.491ms | React with ❌ to remove this embed.
Yup