❔ EFCORE DESIGN ONE TO MANY
Can you guys help me to design a correct one to many relationships in this case
The problem
I have this LINQ below
This one will be cycle because it's include the User and the User have ChatMessages like in the image so this will cause recursive cycle when loading the data
My solution (but fail)
On
User.cs
I could remove the below properties
But I end up need it for setup Delete Cascade behavior
Any suggestion ?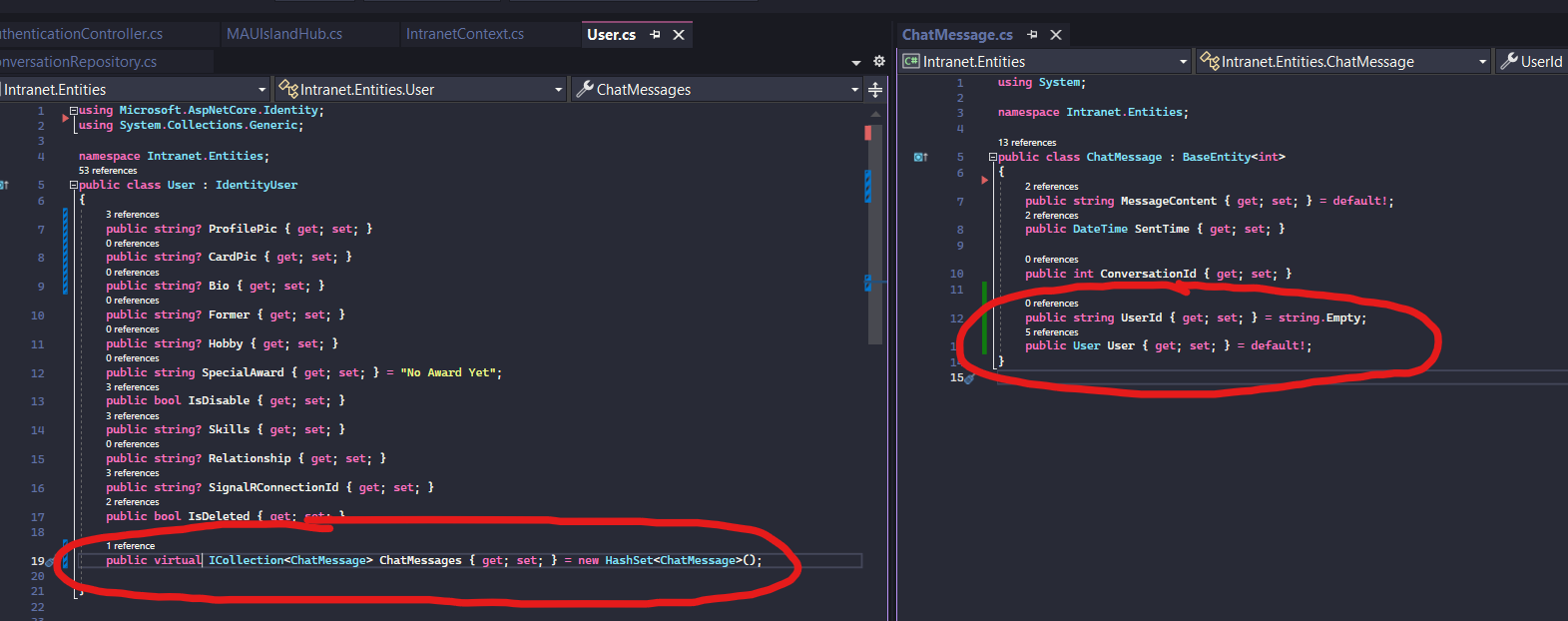
6 Replies
that doesn't result in recursion because a. You haven't explicitly done .Include(ChatMessages).ThenInclude(User).ThenInclude(ChatMessages).ThenInclude(User)... (forever)
and b. because EF doesn't do more queries when it already knows the data it needs (in this case, the original user and/or ChatMessage, which it will put on the ChatMessage/User entity where appropriate, but it won't perform new queries if it already has it). Also c. it will be the same instance (if it's the same User) each time, so you're not going to run out of stack space or anything
The entities it gives you will appear recursive, and if you want to like json serialize them you're gonna have to use serialization options to ignore recursion, but that doesn't mean the SQL query itself is recursive
Who said the sql query is recursive. The json serialization of the object is recursive.
that just means there should be a non-recursive DTO that's being serialized instead of the db entity, not that the db should be restructured. If that's the only problem
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Yes
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.