Struggling to generate new password when user clicks on button.
Hi everyone! I have my code running properly, but I'm stuck on how to generate a NEW password when once the user clicks generate password the second time.
Currently everytime a user clicks generate password it increments each times and messes up the format. I'm missing the next step of the logic to correct this.
https://codepen.io/ezekielswanson/pen/ExegrxB
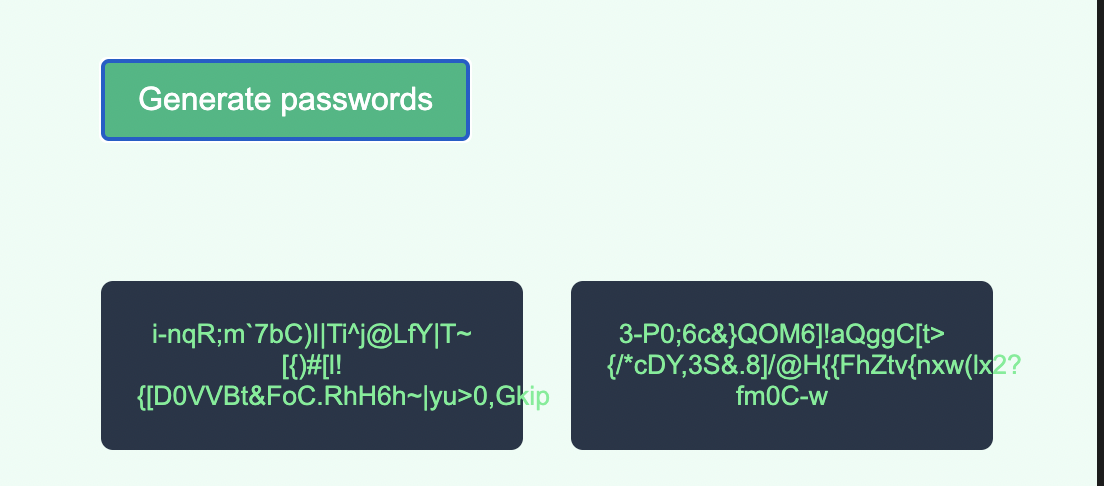
10 Replies
Something like this? Should probably reset both the variables before generating again
Actually above supplied code works in the codepen (I am surprised, I am no wizard in JS)
This works too, but I usually try to keep eventListeners with only functions
Thank you so much for taking time out to answer my question 🙂 This was really helpful!
No worries , glad I could help!
Here's my take on it:
With this method there is no reused string so you don't have to worry about ever-increasing length or about resetting since it's all encapsulated in the function.
And this is the kind of answer I wouldn't be able to think of 😄
Also, I hope that this is just a fun hobby thing for you and you don't expect to actually use it to generate passwords.
Math.random()
is not cryptographically secure. If you want cryptographically secure random numbers you'll want to use crypto.getRandomValues()
. Even then, .getRandomValues()
only works on integers and not floats so you'll need to do some maths to convert the int to a float.
For example, to get 5 cryptographically secure random numbers [0..1):
This creates a Uint32Array
and fills it with cryptographically secure random numbers (but it's an unsigned int, so [0..2^32 -1] ). I then divided it by the max of a uint32, which gives us a rang of zero to not oneAt least I felt smart for a few minutes 😉
Interesting, I did not know that.
Math.random()
is designed to be fast, not secure. Hell, when Math.random()
was designed web browsers couldn't do cryptographically secure numbers
If you're building a dice roller or other random generators for a game it's fine. But if you want to do secure numbers, you need to use crypto
If you want to actually build something like this for production, I'd do some internet searching to find some open source and reviewed examples and check out their codeUntil now I didn't know crypto exists, will certainly look into it. Thanks for the info (i'm sure it'll help op as well)
Also, I'm a newb when it comes to cryptographically secure random numbers. I believe my above formula works (the base is a secure random number so it should) but it's best to consult with real crypto people to verify.
That all being said, here's a quick change to your code to use the cryptographically secure number:
You might see some noticeable performance slowdown with larger passwords as that's creating a new random number each time. If that's the case, doing a refactor where it gets all the random numbers it needs at once should show a noticeable improvement as it's only making one call to the browser's secure crypto source instead of 15+ calls. But performance optimizations can wait until they're needed :p