42 Replies
Working on a zodiac compatibility thing
left side: when the button is clicked, it checks if the zodiac sign for the years are compatible. If so it says it's a match. Else, it's not
how would I go about doing that?
Well, I'd write a method to calculate the zodiac sign for a given date
then another method that takes two zodiac signs and returns a match or a non-match
then you just wire these two methods up together
hmm could I put those years in like an int or something. If int1 matches with int2 it'd say if it's a match or not?
?
I have no idea what the rules for zodiac matching or even calculating the sign for a given date are
wait uhh hold on
are we talking chinese zodiacs that (if I understand things correctly) are year by year?
ie, "year of the tiger" etc
or are we talking western(greek?) zodiacs, like the twins, the fish, the lion etc?
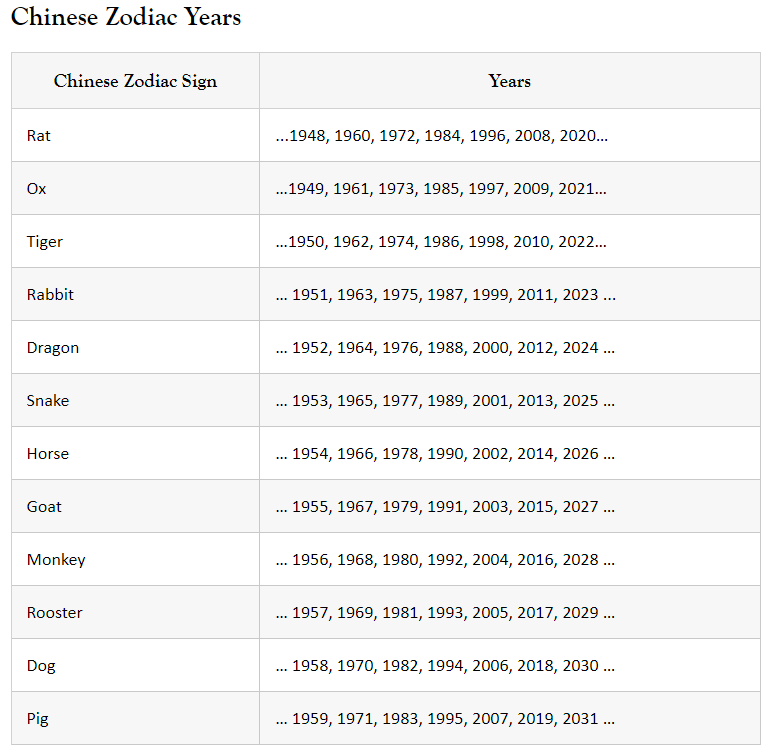
chinese zodiacs I should've clarified sorry
Right, so we only care about the year
yeah
Well, a full list of every year and what sign it corresponds to sounds like a bad idea
better to define a fixed point in time and say "this is the year of the rat"
then you can calculate a difference from the given year and that fixed point, then modulo that to find what "cycle" the year is in
okay, so I think I got something
this test works out - but its using january first as the switch point, aka it goes by the gregorian calendar
im not sure if thats correct for your usecase
here is the code for it, btw
I think I just ended up working on some code spaghetti
then unravel your spaghetti 🙂
but you see how simple this is?
assuming we can look at only the year, that is
Im thinking chinese zodiac signs probably should respect chinese new year, not gregorian new year
just
(ChineseZodiacSign)(date.Year % 12)
, right
ah, you need to find a different starting pointyear 0 was year of the dragon
so if you re-order the enum, that works
I think I just zoned out I don't know where I was going with this

yeah thats the naive approach
and its not a good idea 🙂
You'll want to make a method takes in the year and calculates the correct zodiac sign
that way its re-usable
ah
Okay I'll be honest I'm even more lost now with your method
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeah each sign is just +12 until it happens again
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
all of this is new to me
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
check here.
@Mr. Cres If you're not comfortable with methods, I'd suggest you spend some time focusing on that - its a very important concept
If you want more hands-on help, I suggest you share your code and describe where you are stuck
idk if there's a way to shorten it
you should put all this logic in a dictionary or a json file
No. Just make an
enum
and a single one-line method
as shown abovei don't know how to do that
Like this
You can create your own methods from scratch, you are not limited to only making methods via winforms events
so an easy solution to this would to make a method to convert a year into a zodiac sign
is the simplest version of this
now, this method returns a
ChineseZodiacSign
, so you can create that too. Its not a method, thats its own type, an enum type.
you would NOT place this inside Form1, you would make a new file along side ittwo new files, probably
and a new folder
and then just do
.ToString()
on the result of that ToChineseZodiacSign
method
and then you have your "Rat"
and whatever@Mr. Cres do you understand?
Could you run me through it?
Ero#1111
REPL Result: Success
Console Output
Compile: 708.585ms | Execution: 44.913ms | React with ❌ to remove this embed.
what's there to walk through?
that's literally it
Ero#1111
REPL Result: Success
Console Output
Compile: 644.645ms | Execution: 87.373ms | React with ❌ to remove this embed.
english is not my first language I'm having trouble understanding some parts
so ask about the parts
@Mr. Cres I honestly don't know how to tell you simpler than I already have.
I think I misread the prompt
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.