✅ Operator ?? functions
I came across ?? operators whilst I try to understand solutions like https://leetcode.com/problems/n-ary-tree-preorder-traversal/solutions/3065645/not-fast-but-good-solution-without-any-recursion/
For example there is this line of code `
In this case the ?? operator is ordering the IDE like "hey, the value root.children[0].children can be the value of root.children at the ith position ?
LeetCode
LeetCode - The World's Leading Online Programming Learning Platform
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
9 Replies
The 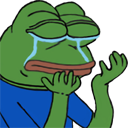
??
operator just means "if that was null then try this"
So var val = nullableType ?? defaultValueThatIsNotNull;
Or if you're feeling adventurous return a ?? b ?? c ?? throw new NullReferenceException();
Although you might not be permitted an exception there let me check that
Looks like you can't use an exception there 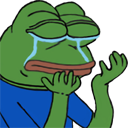
here's what the code actually does
it honestly doesn't make much sense
I disagree.
The code reads
i.e.
I dont know what youre talking about, 

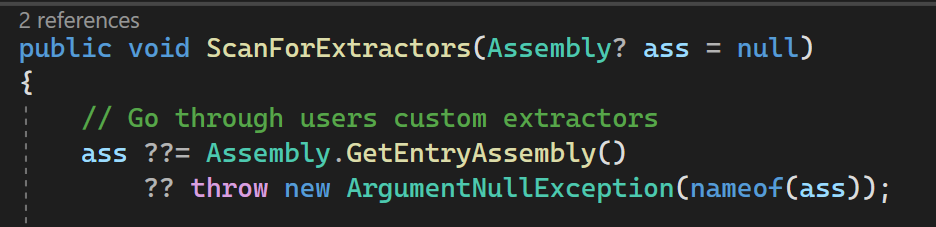
Whoops a dodgy test. Must've been because of the null literal

ya
ah yeah you're right about the add. but it also makes a copy of children, you're wrong there
Ah yes good point it's unpacking and repacking the children how bizarre
There I've edited my original code to fix it
Thank you all for the explanation, I see this operator more clearly than before now 🙂