duplicate methods from .GetMethods()
so above is my code for getting all classes with the group attribute, and then getting their methods (these methods represent commands). as you can see, though, i have to do
.Skip(4)
because one of the commands is getting duplicated 4 more times
. what could be the cause of this? none of the other methods do this.
here's the method i use to grab groups classes.49 Replies
by the way, the groups don't duplicate either
here's the code for my commands that i'm trying to work on
the
UserInfo
command does not duplicate, just the HelloWorld
oh hey extension thinker
ive grown a lot at C# since we last talked
lolCheck what the
GetMethods()
actually returnskk
There might be some things you wouldn't expect
If it's the exact same method repeated four times then that would indeed be very weird
well how i know its
the exact same 4
is because
if you
.ToString()
each method
you can see the parameters and theyre all the exact same,
DiscordMessage message, string[] args
OH SO NOW IT DOESNT WANNA PRINT THEM
š
thats so weird
cuz yesterday my assembly was returning those values
but now when i do this
it prints just 2
whic his the normal amount
hmm
okay so
now with the code i have
it does this.
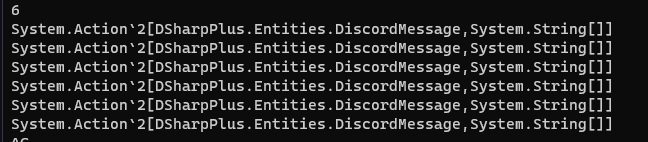
why does it print different values each time..
like the number of values i mean
look
so in the loop
it prints 2 like normal
but when i apply it to that variable
and print the length and the method list
it gives me 6 instead of 2
just the
HelloWorld
one is being duplicated..
but also
on the second line it returns actions rather than tasks ??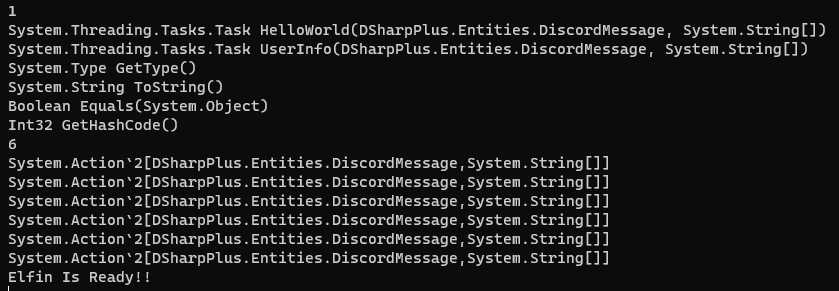
wait
what are you actually printing in the second
i know the issue sorta
that looks like you're printing a method
oh yea i forgot to remove that
its just the
response function
for the cmd
yeah so you're printing that
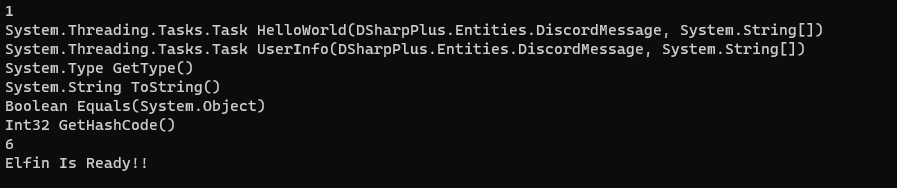
that looks fine
oh im slow
HELP
I JUST REALIZED
š
it was the way i
was printing gthings
that tripped me up š
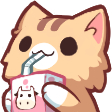
um i have a question though
another one
let me test something first
hold on
okay so,

this prints as expected
right but
what im trying to do is
apply a custom response property
that is basically the command's method call
the 11111 prints but
the method does not invoke
im not sure what i did wrong
no error is thrown..
but it doesnt print anything in the command's method
btw you know D#+ has a commands module, right
i know
i prefer to do things on
my own
for max customizability and
you get my point i hope
but yea idk whats wrong
the docs on
.Invoke
r confusing but i know that i need to pass the parent class
but i dont know if im passing the rest right
heres the full code for that
(just for context)!docs MethodInfo.Invoke
Could not find documentation for your requested term.
the web docs are a bit confusing
š
MethodInfo.Invoke(Object, Object[]) Method (System.Reflection)
Invokes the method or constructor represented by the current instance, using the specified parameters.
The first argument to
Invoke
is the object to invoke the method on (or null
if the method is static). Here you're passing newCommand
as that object.yes
And
newCommand
is a ElfinCommand
, which probably doesn't have the target method, righttarget method?
oh
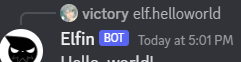
it seems to work when
you change the commands to static methods
i wonder why š¤
thinker227#5176
REPL Result: Failure
Exception: TargetException
Compile: 655.940ms | Execution: 104.722ms | React with ā to remove this embed.
yeah because the
obj
argument is ignored if the method is static
otherwise this will happenhmm
well i mean there seems no reason for the
object to be accounted for
its simply a holder class lol
so that sorta makes sense
well, if it's an instance method, then you need an object to invoke the instance method on
if it's static, then you don't need an object to invoke it on
what exactly is an instance method?
a method which isn't static
ah
so any methods that are non-static are instance methods?
okay i thought so
something that is for a constructed class
gotcha
umm could you help me with one more thing?
sure
its not a problem
exactly its
i just want to be criticized on my code because
it seems messy and odd
so i want to know what i can
do better
post in #code-review
kk ty