type checking error??
import { GetServerSidePropsContext } from "next";
import { getServerAuthSession } from "../server/auth";
import { Session } from "next-auth";
type Callback = (data: { session: Session }) => void;
type RoleRedirects = {
[key: string]: {
[key: string]: string;
};
};
const roleRedirects: RoleRedirects = {
cashier: {
ADMIN: "/admin",
MANAGER: "/manager",
},
manager: {
ADMIN: "/admin",
CASHIER: "/cashier",
},
admin: {
MANAGER: "/manager",
CASHIER: "/cashier",
},
};
export async function roleGuard(
ctx: GetServerSidePropsContext,
cb: Callback,
role: string
) {
try {
const session = await getServerAuthSession(ctx);
if (!session) {
return {
redirect: {
destination: "/",
permanent: false,
},
};
}
const redirectDestination = roleRedirects[role][session.user?.role as string];
if (redirectDestination) {
return {
redirect: {
destination: redirectDestination,
permanent: false,
},
};
}
return cb({ session });
} catch (error) {
return {
redirect: {
destination: "/",
permanent: false,
},
};
}
}
import { GetServerSidePropsContext } from "next";
import { getServerAuthSession } from "../server/auth";
import { Session } from "next-auth";
type Callback = (data: { session: Session }) => void;
type RoleRedirects = {
[key: string]: {
[key: string]: string;
};
};
const roleRedirects: RoleRedirects = {
cashier: {
ADMIN: "/admin",
MANAGER: "/manager",
},
manager: {
ADMIN: "/admin",
CASHIER: "/cashier",
},
admin: {
MANAGER: "/manager",
CASHIER: "/cashier",
},
};
export async function roleGuard(
ctx: GetServerSidePropsContext,
cb: Callback,
role: string
) {
try {
const session = await getServerAuthSession(ctx);
if (!session) {
return {
redirect: {
destination: "/",
permanent: false,
},
};
}
const redirectDestination = roleRedirects[role][session.user?.role as string];
if (redirectDestination) {
return {
redirect: {
destination: redirectDestination,
permanent: false,
},
};
}
return cb({ session });
} catch (error) {
return {
redirect: {
destination: "/",
permanent: false,
},
};
}
}
const redirectDestination = roleRedirects[role][session.user?.role as string];
const redirectDestination = roleRedirects[role][session.user?.role as string];
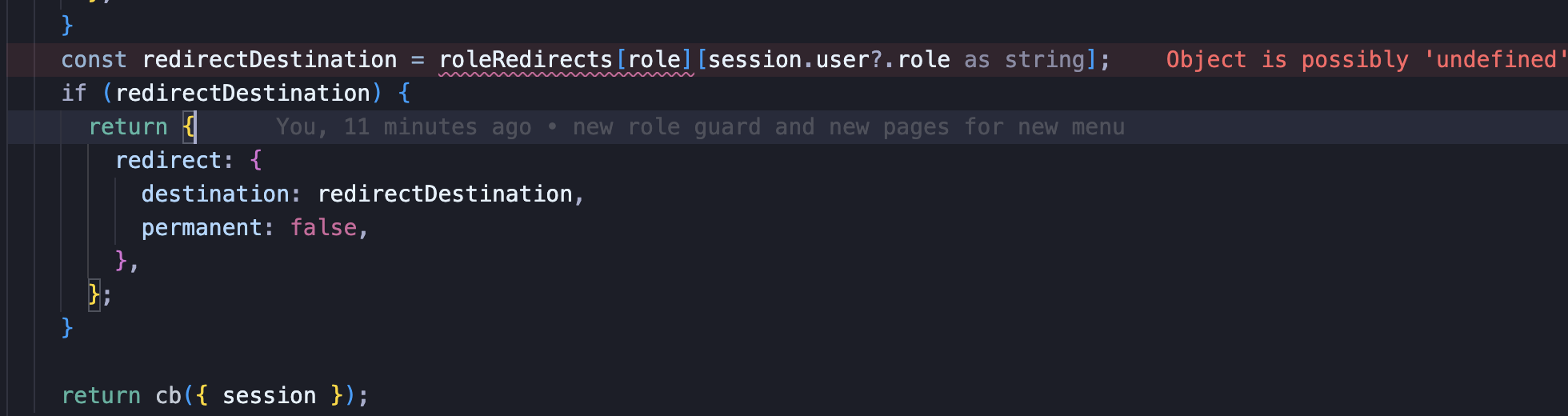
4 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
could also just type the function args more precisely
const role = {
cashier: {foo: "bar"},
manager: {biz: "baz"},
admin: {hello: "world"},
} as const;
type RoleType = keyof typeof role;
function doThingWithRole(roleKey: RoleType) {
const foo = role[roleKey];
}
const role = {
cashier: {foo: "bar"},
manager: {biz: "baz"},
admin: {hello: "world"},
} as const;
type RoleType = keyof typeof role;
function doThingWithRole(roleKey: RoleType) {
const foo = role[roleKey];
}
im trying to use this but it show different error
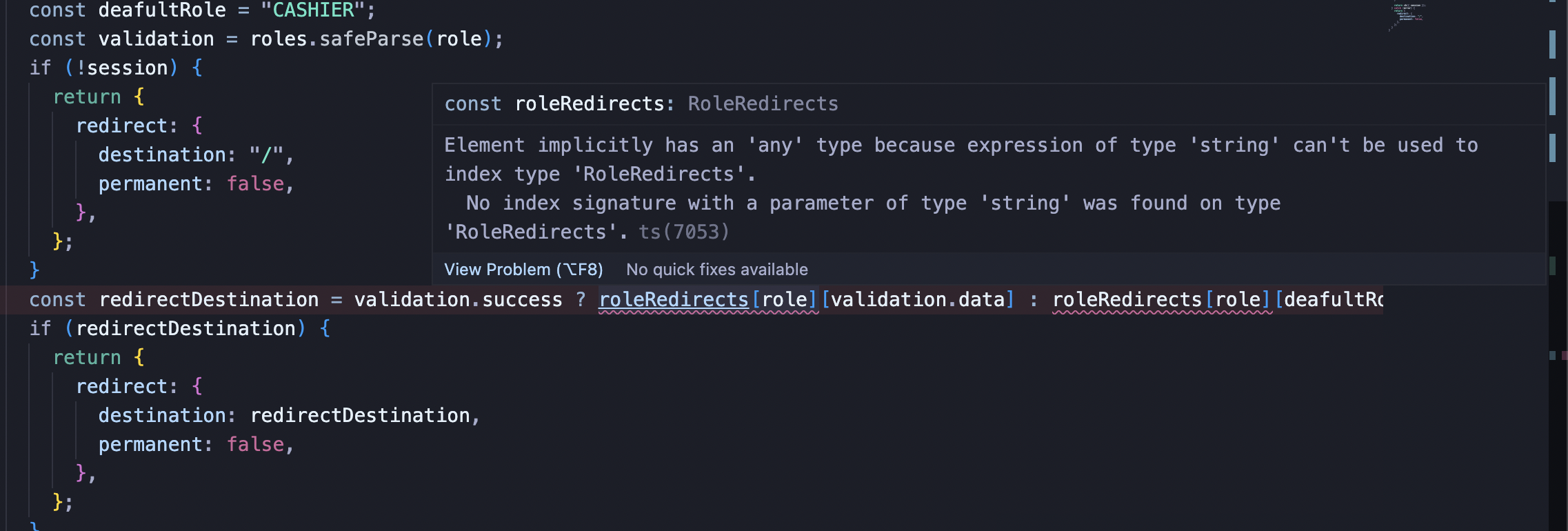
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View