❔ SELECT MAX ID from textbox
Why is this displaying this error?
System.FormatException: 'Incorrectly formatted input string.'
This is my table sql
This is the code im using and what i want is select the max id of apples in the project number that is in the textbox
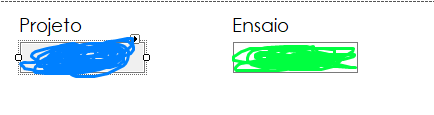
241 Replies
The number that will be in the blue box will be the number of the project that i select, thats done.
But i want the greenbox to show the max id from that project in that textbox, what do i have wrong in my code?
Breaking some best practices here for sure, but to find what is causing that exact error, I suggest using breakpoints
Im going crazy with this, it seams easy to solve but im so tired of watching the same code over and over again
so, set a breakpoint at the top of your
InserirIDEnsaios
and run the debugger
it should break, and you can now step line by line
Im guessing its the new SqlConnection
line thats doing it, but I'm not sureThe error is displaying in this line
novoID = int.Parse(cmd.ExecuteScalar().ToString()) + 1;
I thought it was because it had no data
but i inserted some data and still displaying thisah!
well, its a bit weird that line
you are using
int.Parse
, which WILL throw exceptions if the answer isnt an int and NOTHING BUT AN INT
so maybe store the result, check what it is before parsing?
var result = cmd.ExecuteScalar();
its saying that is 0 but it isnt

at what line is it 0
make sure you are at least on the line after it's been set
also, try to see what happens when you put a
'
in your txtNIDPROJETOS text box :)in the propertie text?
In the textbox ye
Did nothing :/
Just type it in the form
what do you mean, it did nothing
System.Data.SqlClient.SqlException: 'Unclosed quotation mark after the character string '''.
Incorrect syntax near '''.'
if you put a breakpoint on that line and step through it
still the same
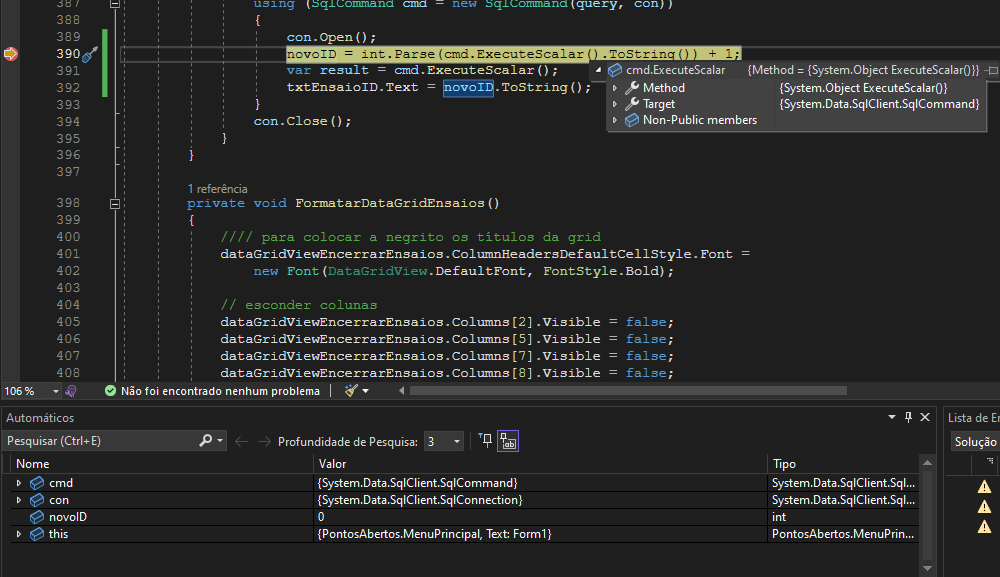
well
read the code
you are executing the command twice, which is a bit weird
and you know its gonna crash on the int.Parse line
This is basicly incrementing +1 to the max id that is in the table
so you put the stuff I suggested to debug this AFTER the line that crashes
i normally use this because my teacher a few years ago he told me to do it like this
What i want is basically read from the textbox, get the number that its in and see whats the max id from that number
I understand
Now please
break up that line that we know is crashing
execute the command as its own step, with saving the scalar return value on its own
THEN you can do the whole .tostring parse thing, if you want
we just want it stored in its own variable so we can easily debug and see what the returned value is. understand?
Im a rookie in programming to be honest i have no clue what to do :/
I always had a default way to do things and now that isn't working because maybe its more complex im a lil scared ngl
9898 is the project name and the 2 is the number of essays in general.
i changed the sql statment and i could open my app.
But its still showing the max id of all essays and not atrributed to the textbox

string query = "select max(nensaio) from tbl_acoes where idProjetos like '" + txtNIDPROJETOS.Text + "%'";
this is what i changed to
also tried this and still not working:
can we go back to the scalar for a sec?
I don't think you actually tried what I suggested
yeah i said i didnt understand
let me put the code how it was
Do you not understand basic variables?
If you don't understand how to create and use variables, you should not be doing GUI and databases, to be honest
No, i was a front-end dev and im comming to make c# applications to get more understanding
okay
I think the best way to get in touch with these kind of stuff is throwing me to the wolves you know?
yes and no
you need a solid foundation first
then come the wolves
I really wanted to finish this at least, i know i need to understand more and more about it
i need to make a research about online courses or something to guide me
this is way more entertaining then front-end
Could you help me finish this?
sure, but you'll need to actually listen and give it some effort
I will 100%
Im motivated to learn and focus , thats why i was trying to make this on my own
but stuck for a weak was making me crazy
i see a huge gap on my knowledge about this 100%
okay so lets revert to the simplest version for now
if you put a breakpoint on the
var result...
line
and step through it
what value does result
have?
also, please note the return type on cmd.ExecuteScalar()
its returning null
so its not getting any result right?
yep
if you also check the calculated value of
query
, and run that in some database program (SSMS?)
do you get a result?1 sec
if i run the query like this:
its showing this
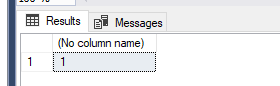
where idProjetos = idProjetos
that doesnt really make sense?
thats like saying where 1 = 1
isntead the last idProjetos i had the number of a project
and that was saying NULL
ofc because i didnt had anything to it
sure, if you had no rows at all you'd get no results
aka a null result
or actually, idk what
MAX
returns for an empty set.. null makes sense thouyes but project number 668 i know that has nensaio registered
and i was focusing on that one and it was showing the same error
okay
so if you run
select max(nensaio) from tbl_acoes where idProjetos = 668;
in SSMSshows NULL :/
but here you can see the last column

this one was added by hand
this is my table tbl_acoes
uhm
well
looks to me like you have only one entry in that table
and its not project id 668
my idProjetos is 3 that is equal to 668
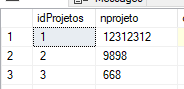
but your query isn't for
nprojeto
you are doing where idProjetos = 668
yeah but the idProjetos is the primary key
yes?
its auto increment
so?
so if i talk about idProjetos
wouldnt it search as nprojetos to?
.. no?
why would it?
well because if i want to get information about the nprojeto 668 i can search it by idProjetos 3
sure
OR
just search by
nprojeto
yes i guess its the same?
so i should be searching for the nprojeto?
and not the idProjeto
wait
so i was saying that
the number 668 was in idProjetos
you should query the field that contains the value you actually want to search for...
and thats why was saying null?
also, legit question here
why dont you name your tables, variables, methods etc in english?
Because im not english, but i will start doing that for sure
makes the code easier to read and understand for everyone
yeah
ur right
I'm not english either, all my code is
but heres a thing
anyways
I would highly suggest having your primary key just be
Id
in my tbl_acoes i dont have the nprojeto in it , i have the idProjetos
Do NOT fill in
we'll fix that later
in your texbox
for every table?
yes
they are always accessed as part of a table anyways
SELECT Projects.Id, Projects.Name from Projects
for example
orso the thing is wrong here is my sql statment
SELECT x.Id, x.Name, y.Id from Projects x, OtherTable y
right?
yes
also, you are querying a number field, but giving a string as your search parameter
and as far as I can tell,
acaoID
is actually your primary keyyeah from table tbl_acoes
thats the only table you have shown us
i always try to distinguish the primary keys of every table
and its the table you are querying
Hm, I understand why you think thats a good idea
but its not 😛
got it
not the problem here however
lets fix your query
for now, lets just skip the
WHERE
all tgether
select max(nensaio) from tbl_acoes;
if you run that in SSMS, do you get a hit?one
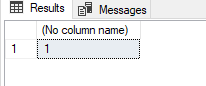
idk why is it saying no column name tho
thats fine
its expected even
since you are not querying a column, you are querying the result from a function
so, show me your C# code
great
run that, with the breakpoint, inspect the value of
result
well result still null :/
hm
thats.. unexpected
do you have a microphone and time to jump on a screen share?
in half an hour right now i have my kid sleeping right next to me it doesn't give me much comfort or either my wife will cut my throat 😅
Understandable.
If you can in 30 minutes we can hop on a call no problem about it
I had a few problems at home can we talk maybe later or tomorrow?
Sorry for the inconvenience
Hi, sorry yesterday was kinda difficult for me to come to the pc so do you wanna have a look at this @Pobiega ?
I stopped here:
so maybe i think i should work on the structure itself
so give me one second i will do it all over again and in english so we can get a better view/idea
well now i have a question, im givinf the same name id for each table
but now i want to make a foreign key to it
and this would be weird because this is my previous structure for table_essays
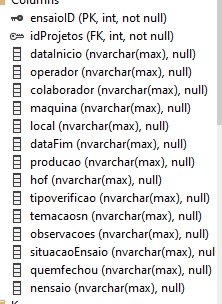
how am i gonna do this?
this was why i was working with different names as PK's
erm, your FKs usually have names like...
"TABLE1_TABLE2_FK"
as in, the actual FK
lets say a
Project
links to an Account
in your Project, you'd have a AccountId
and that is the Id
column in the Accounts
tableok let me see if i can put this for me
So basically i have
Table_Projects
and Table_Essays
So Table_Essays
get the id of the project
so on Table_Essays
i have to add a field called ProjectID
right?yes
And regarding the names, dont prefix the tables like that
Just call it
Projects
and Essays
got it i will change it real quick
👍
so now to create FK's its in relationships right?
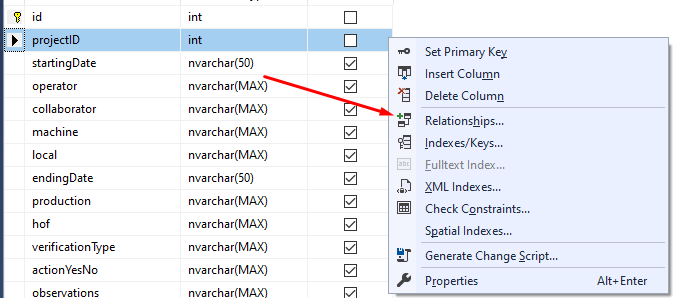
Was a long time since I set tables up manually, but yeah sounds reasonable 🙂
so like this the PK is from Projects and the FK is on Essays table
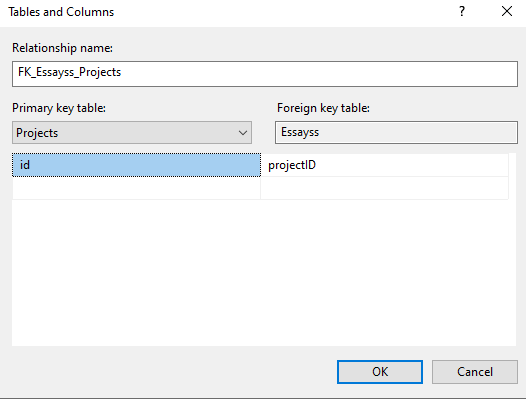
i think i will do it in SQL idk why isnt working 🥲
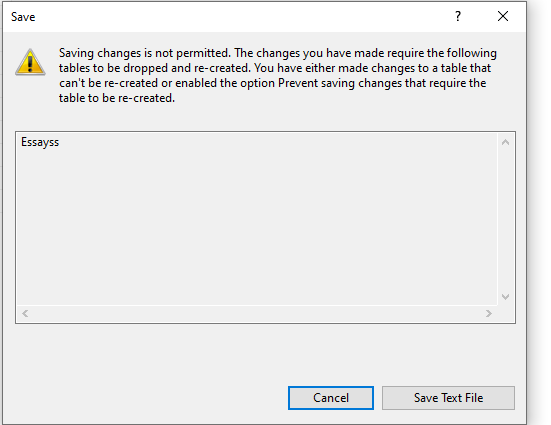
no idea 😛
I'll start my ssms and try
either way i did it like this
sounds about right
well
now i think its the most
confusing part of it
I have 3 Tables:
Projects
Essays
Actions
a project must contain essays, but the essays may and may not have actionsSure
so i need to add a FK on
Actions
of the id
in the Essays
yep
well idk why if i place another int data type in my table it doesnt let me save it
well i see its not the data type
but have no clue why this is showing
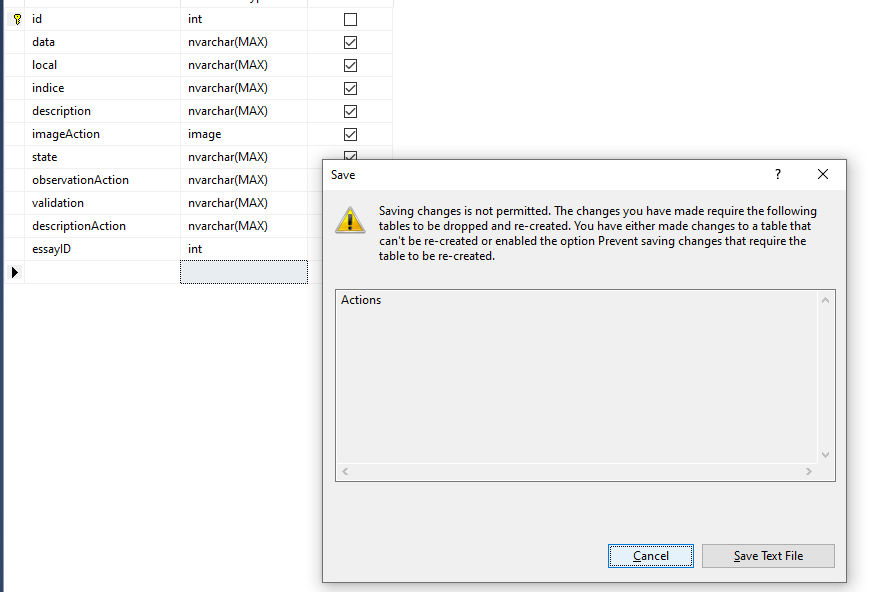
¯\_(ツ)_/¯
it accepts it only as nchar o.O

As said, I don't really use the designer at all
I almost exclusively use Entity framework with code first, and thus migrations
u even create the table as SQL command ?
no, thats all done via migrations
But this is fine for now, as long as you can get it working 🙂
but yeah Im having the same issues with SSMS, any adding of a new column when FKs are present gives that error
very weird
but adding stuff via
ALTER TABLE [x] ADD [y] ...
works fineyeah
this is what i did for table Aactions
but now i have a question
sure
Aactions
can and cannot have Essays
associated, how can i make it if doesnt have an Essay, stays associated with the Project?
Is that on code and the SQL structure is fine or do i need to have a FK from Aactions
to Projects
?So an action is always associated with a project, but the essay link is optional?
the opposite
the essay is always associated
but you said essays are optional
and the action can associate with Essay or Project
i mean actions
sorry
?
actions are optional
not the essays
well if they are optional, doesnt that just mean they dont exist?
like, you can have a project with one or more essays and not a single action
thats fine, and your design already supports that
well good then
remember, relationships are kind of inverted in SQL
a project doesnt "own" essays, essays instead indicate what project they belong to
yes
im gonna make the design of the page real quick
something simple
How about we focus on getting to the point where you can actually query the database from C# first? 🙂
oh yeah
databinding it right?
no, please no.
using server explorer?
no
how then 🤔
something like this
this is a console app that just queries my local database
its as simple as can possibly be, but it works
Oh yeah, i forgot to tell you so im doing the CRUD all over again, since i turned everything and in English , that's why i was saying i was designing the page
sure, but that can wait
lets get the actual query working
oh okok
so i need to create that class
because yesterday, you kept getting null regardless of what you put in your query
this isnt a winforms app thou, its actually a console app
just to get the smallest possible test
ohhh i cant use the resource using because this is C#7.3 and i need to use the version 8.0 or higher
why are you using a really old version of .NET?
if you are limited to 7.3 Im guessing you are using .NET Framework, probably 4.7 or 4.8
well im not limited
i can change to the most updated one
I would encourage you to do so
.net framework is legacy and only getting security fixes at this point
while .NET (currently at version 7) is the future and getting new content
How can i update it here in visual studio 2022?
never done that
if you have VS 2022, you might already have it
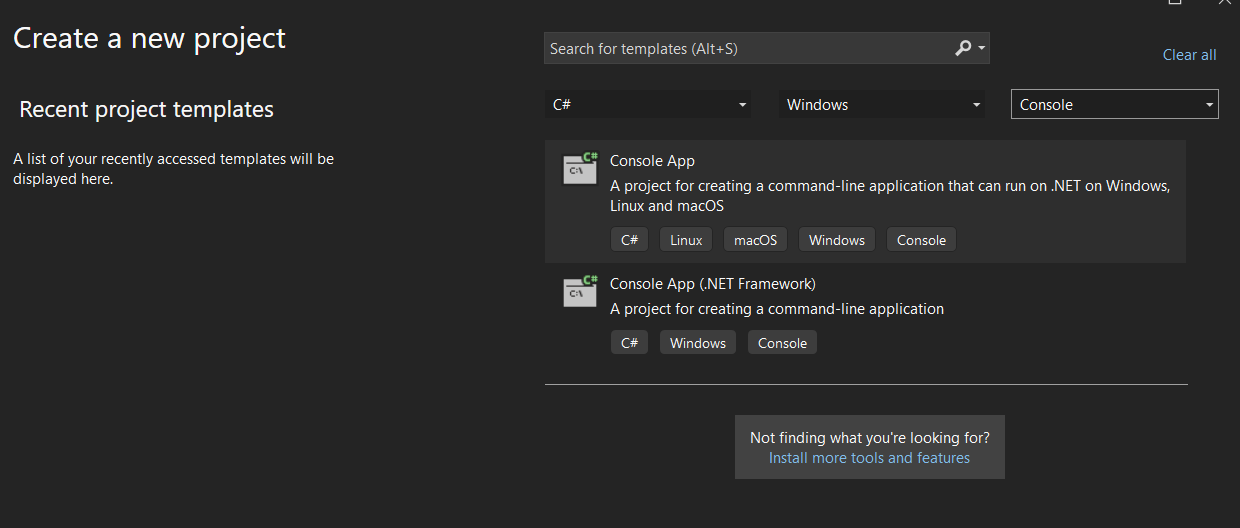
the top one is the modern version, the bottom one is old and legacy
this goes for winforms too
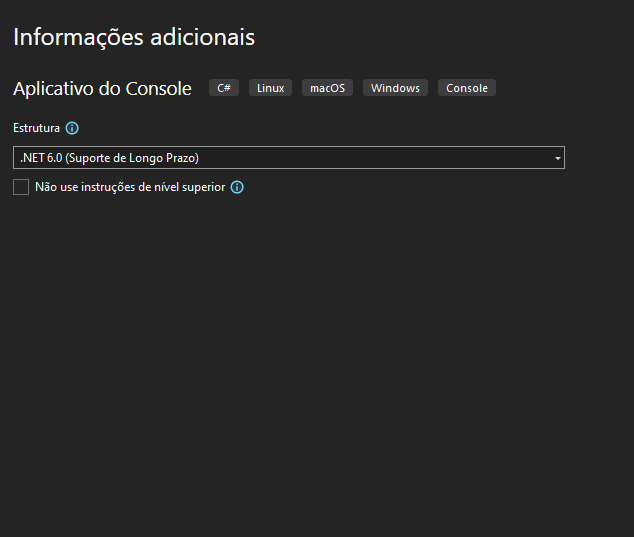
the other option is .NET 7.0
go for 7 🙂
do i check the checkbox?
6 is long term support, 7 is short term (8 comes in november and will be the new LTS)
hang on, I dont speak portuguese
oh sorry
dont use instruction of higher level
"dont use top level statements" 😄
ah
proibably best to check it for now
.NET recently introduced the ability to skip the boilerplate in the main class, and its nice but often confuses people at first
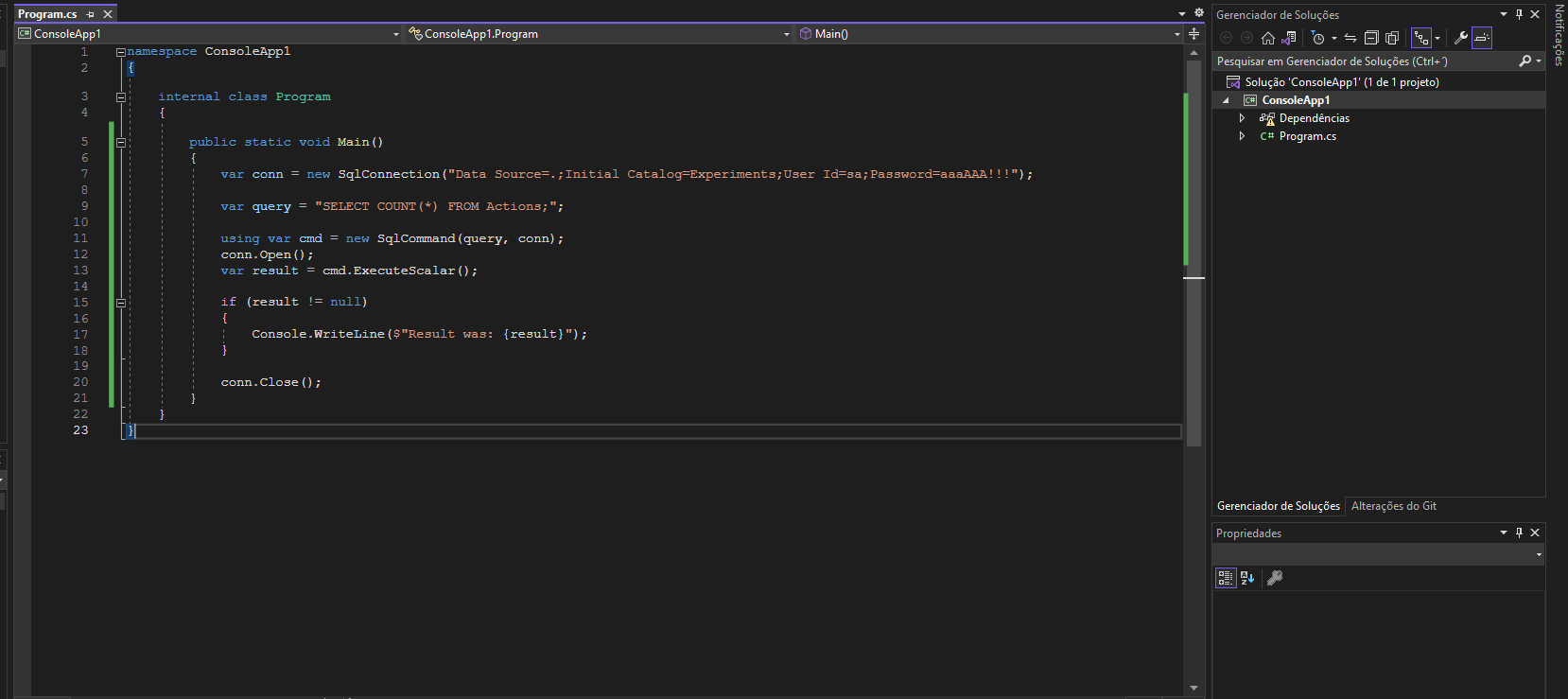
all good 4 now
great!
now, just change the connection string
well
yeah
i need to add the SqlConnection
because its not getting recognized
right, you need to add the NuGet
System.Data.SqlClient
wow winforms does all this automatically
not really
winforms also needs to add the sql client, Im pretty sure
if you have SqlConnection or so it adds the System.Data.SqlClient;
either way its done i will get the connection string
it will do that here too, once you have installed the package
how can i get the conenction string in SSMS?
if you rightclick on "dependencies" in the solution explorer, you should see "manage nuget packages"
thats where you can install it
I have no idea 🙂
got it
i've seen connection strings like this
whats the difference of the
@
behind the double quotes?it means "verbatim string"
it escapes stuff
oh ok
or rather, it doesnt
so
"\n"
and @"\n"
dont mean the same thing
the first means "newline"; the second literally means "backslash-n"okok got it
connection string done
okay
give it a spin
I have a single row in my actions, so I get
1
as my resultResult as 0
thats good, means the query succesfully ran at least
assuming you do indeed have an empty actions table 🙂
yes i have ^^
great!
that means you can now start working on the winforms app 🙂
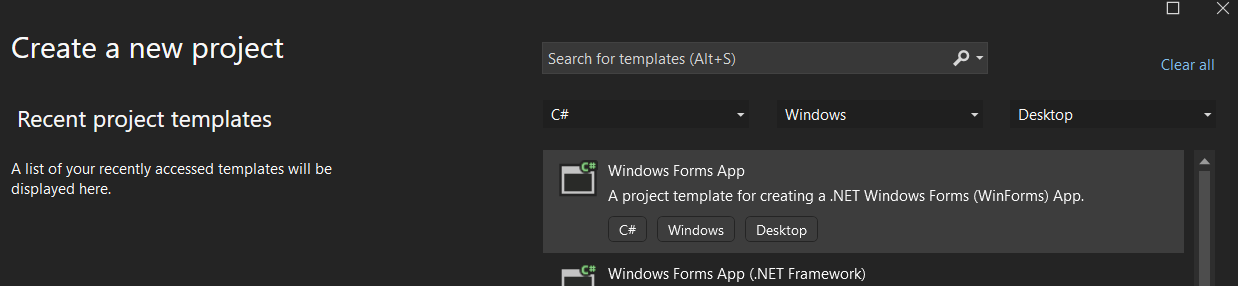
that's what i use yeah
one question before i start
you sure its the .net 7 winforms you are using? 🙂
should i make the login system now or i can do it later?
i would love to make some sort of login system that saves the user data
If you want a login system, you should do a login system
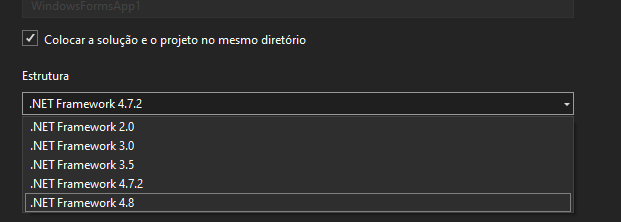
you picked the other one when you made the project
so i go for 4.8?
no, you create a new project and select the non-framework project
🙂
ohhhhhhhhhhhhhh
i see
.NET 7.0
yup!
right?
in the other project i was working with the .net framework
so should i make it now ?
why not
if you want one, now is a good time to make it
because I think future systems might need to integrate with it
kinda nervous about it 😅
like..
When adding a new [something] to the database, also write down who added it. Get data from current logged in user.
i've been following some tutorials on the internet but its a little bit confusing
yeah that would be cool
so first of all i need to make the users table
well table users done
wow
this is way different
i cannot double click the button and give the code for it
do i need to say it as event?
uhm, you should be able to
it goes for the MainMenu_Load
when i double click
works for me
weird
I dragged out a button, double clicked it, got an OnClick event
gonna restart the visual studio
👍
now im getting the event
I'd like to recommend a few things to you btw
first is
Dapper
its a way to easily bind your SQL query results to objects in C#, makes it SOOOOO much easier to work with SQLi've heard that before but never used it
You'll also need to think carefully about how you structure your app, you dont want to be writing your database code inside the Clicked event directly
it would be a lot better to instead just have the button issue a command of some sort to a "different part" of the application and let it handle the actual work
is this good?
Not really 🙂
🥲
its mixing database code with logic and presentation
so you are mixing three things that should not be mixed
wow
i've been doing this for a while now 😅
haha thats fine, we all did when we first learned
but the code quickly gets messy, and you might end up duplicating a lot of logic
yeah
if i show u my last project u would cry
🥲
500 lines or so the majority being sql connections xD
doing this my whole life and now seeing the dumb things this creates jeez
so how would you do this button ?
or whats the proper way to do it
oh boy
oh thats a nono
🤣
first, I would use a "Unit of work" pattern to ensure transactional security
I would likely use EF, but since you are not, I'd use dapper and write repos around it
this one right?

yes
i installed the nuget allready
should i create a class to make my functions there?
yes
i was trying to search anything online or a video i could follow to do this but im lost
something like this
all i get is for websites :/
note that the code above is mostly for demonstration, I just whipped it up right now
yeah i saw that
its missing some asseblys, for the login system i need Syte.Data.SqlClietn;
But i need sum more
well, this code assumes...
thats it
I created the rest myself
how do i use dapper then im fully confused
thats using dapper
the
.Query<T>
method is from dapperyeah but its stil displaying as an error

well you don't have a
DbUser
classthis is a new world for me ngl
I was used to one thing and now it's all very complicated
You know what a class is thou, right?
yeah
but the thing is i've never coded like this before
So the idea of mapping the response from a database query to an object (an instance of a class) shouldt be that weird
idk maybe im just confused, tired , idk i slept 3 hours
Well, to be fair, it is quite the step up from "just write the code that does the thing" to planning and separating out your code
but it makes for better code
true
Do you have time for a screenshare call? I could show you the ideas
and how I go about doing stuff like this
i think im gonna rest a lil bit and we can have a look afterwards not feeling quite good
I leave no guarantees that I have time later 😛
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.