Page breaks when fetched/returned by worker
I have this public share link: https://view.monday.com/3776000595-36cfd13f4d0b049a330a3952bdae0ff9
and this bit of worker code I copied from the examples:
If I go directly to the link in my browser the page loads like I expect but if I instead fetch the same page with a worker and return it, then the page is broken.
Anyone have any idea what I can do to get this page working?
13 Replies
Here's an example worker that is running that code above so you can see what I mean by "broken".
https://soft-glitter-fa33.polished-geek.workers.dev/
What is this Worker supposed to do?
Is it just a proxy?
well eventually the worker should to be able to edit/inject html/styling into the page
but for now I was just getting started so this was just a basic test of fetching and returning it.
Does this look right? https://mondev.goalastair.com/
Ya, that is working
First big issue, your setup means every asset is returned with the
text/html
content-type
. This works fine if it is HTML, but if it isn't, it tries to interpret JS/CSS/JSON as HTML, which causes problems.
Easy to just make it simple:
Right now it just returns directly, but you could also intercept the response later on, to add your own stuff to it.Ya, I started with this much simpler bit of code before I copied that block from the CF example docs.
But I didn't realize I need to fiddle with the hostname to match.
Thanks for the help @HardAtWork 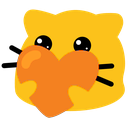
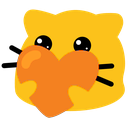
No problem!
I feel like none of the snippets I copy from CF examples ever actually work for me
I went and added the example code for
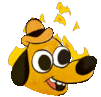
HTMLRewriter()
but it doesn't seem to do anything. And in general the docs for HTMLRewriter()
feels incomprehensible compared to something old school like jQuery I'm used too.
@HardAtWork When searching the discord history, it looked like you help a few other people with HTMLRewriter()
in the past so I was wondering if you have an idea before I made a whole new thread about it.Let me see...
Again this was just the barebones test based off the example, where I'm trying the rewrite the "Powered by..." link at the top right to be
https://soft-glitter-adfgadfgadfgadf.polished-geek.workers.dev/
tuesday.com
instead of monday.com
.https://soft-glitter-adfgadfgadfgadf.polished-geek.workers.dev/
That may be a bit more difficult. Monday seems to be using Client-Side React, which means the initial HTML the Worker receives doesn't actually contain the
Powered by Monday.com
button. Rather it is probably included somewhere within one of the JS bundles that the HTML later requests.I don't think there is a way to render the page within the worker, and even if there was it might not be very preformant or cpu time efficient....
So I guess instead of messing with the html I need to instead inject some javascript that will wait until the page is rendered to start messing with 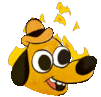
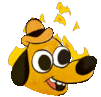