Hi im not sure that my code is working as it should(class in c sharp)
providing code here👇(When i add +1 or +10 to the GetX or GetY isn't it supossed to change?)
35 Replies
When i add +1 or +10 to the GetX or GetY isn't it supossed to change?
$details
When you ask a question, make sure you include as much detail as possible. Such as code, the issue you are facing, and what you expect the result to be. Upload code here https://paste.mod.gg/ (see $code for more information on how to paste your code)
i did
Actually, it doesn't mention title.
But either way, please name your title well, instead of naming it like
it doesn't work and I don't know why
$codeTo post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/i wrote the code
You failed the highlighting
i dont know how else to name it
make sure you write a new line
when you write cs
ok
like this
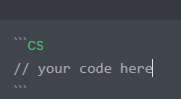
@Buddy delete ur msgs so ppl wonth ave to serch for the code
What is SetX?
and SetY
its up on the code
and the other functions
It is not, you have not posted that.
my code is too long
here
you can use this website if it's too long $paste
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
its ok it works
but ty
but i need help with my question
You are not printing the variables that you have set
ToString is returning them
You must use the newly declared variables
it does not set the values of point1, point2
how can i change them then
without changing the set i mean
You can't, unless you add that to your Point class or make x / y public
so i cant change it in main without changing the set?
GetX
/ GetY
returns a copy of x, it is not the reference of x.
$refvsvaluebut then i add to it the +1
it doesnt affect the actual point?
So based on your current code, the only way to add to X is:
ohh okay ty
how do i call PrintDistance in main?
Point.PrintDistance
as it is a static method
$static
In C#, static allows you to have members (classes, methods, etc) that are not tied to any particular instance and are therefore always, globally, accessible. When applying
static
members, take the following considerations:
• If there are to be multiple instances of a class, do not use static
• If you need to track state, do not use static
• If you are going to have multi threaded workflows, do not use static unless you're aware of the caveats
static
is best used for stateless methods, extension methods/classes and in areas where you understand the pros/cons. Read more here: https://docs.microsoft.com/en-us/dotnet/csharp/language-reference/keywords/staticthank you
but if i try that with IsEqualTrinagnle it doesn't work, is it not a static method?
does bool count as static?
static
keyword count as static