Hi i need some help with classes, im pretty new to this and i have an error with my code.
help would be appreciated(in csharp)
40 Replies
need to explain your problem before anyone can help
can i sharescreen my code on a call?
i dont know how to explain it
no
if you can explain it on a call, you can explain it over 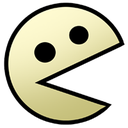
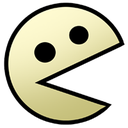
i just want to show my code
not talk
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/
public Point(Point another)
{
this.x = another.GetX;
this.y = another.GetY;
}
this part doesnt work
ok
how does it not work
idk it just syd error
what's the error
"Cannot convert method group 'GetX' to non delegate type 'Double'
ok
you see how in this line:
double dis = Math.Sqrt(Math.Pow(this.x - another.GetX(), 2) + Math.Pow(this.y - another.GetY(), 2));
you're doing another.GetX()
and another.GetY()
?ya
do you see a difference between those and
this.x = another.GetX;
?no
i need two x's
the difference is the
()
after GetX
if you just have GetX
, that's the name of the function
if you have GetX()
, that's calling the function to make it do something and give you a resulti know
i want that tho
in
?
are you sure?
yes
but that's not working
😛
yes that the problem?
is*
when i remove the () it make more errors
yes... so you want to add the
()
i did
and?
so instead of getX i do GetX()
?
yes
ok thank you
if i do GetX in another part of the code will it be the same GetX as the last one?
explain what you mean in a bit more detail please
it will call the same function, but the object it calls it on may be different
does the user need to put the X in the function twice?
i mean, whenever i call the function does the user need to put a new X again?
you don't have to put a new x in anywhere when you call
another.GetX()
?thats the question yes
i don't understand your question - maybe give two examples of what you mean, one with 'putting in the X' and one without
it calls the function twice but do i need to put the x twice too?
i really don't know what you mean by 'put the x'
are you thinking you could just write
.Get()
or something?no no, sorry english isnt my first language idk how to explain it
fair enough, it's difficult
do you mean the fact that there are two
x
s in this.x + another.GetX()
?uhhhh no I don’t think so it’s probably nothing thank you