Having trouble getting files from my NextJS Application
I am building a t3 Typescript Nextjs application and I have a worker which I've set up like this:
I am able to upload images like this in my /api/images/upload route ` But when I want to download them in my api/image/[id] route, then I simply get an empty object. If I make a get request to the same URL from the cloudflare page, I actually get the image how I am supposed to. What am i doing wrong with my request?
I am able to upload images like this in my /api/images/upload route ` But when I want to download them in my api/image/[id] route, then I simply get an empty object. If I make a get request to the same URL from the cloudflare page, I actually get the image how I am supposed to. What am i doing wrong with my request?
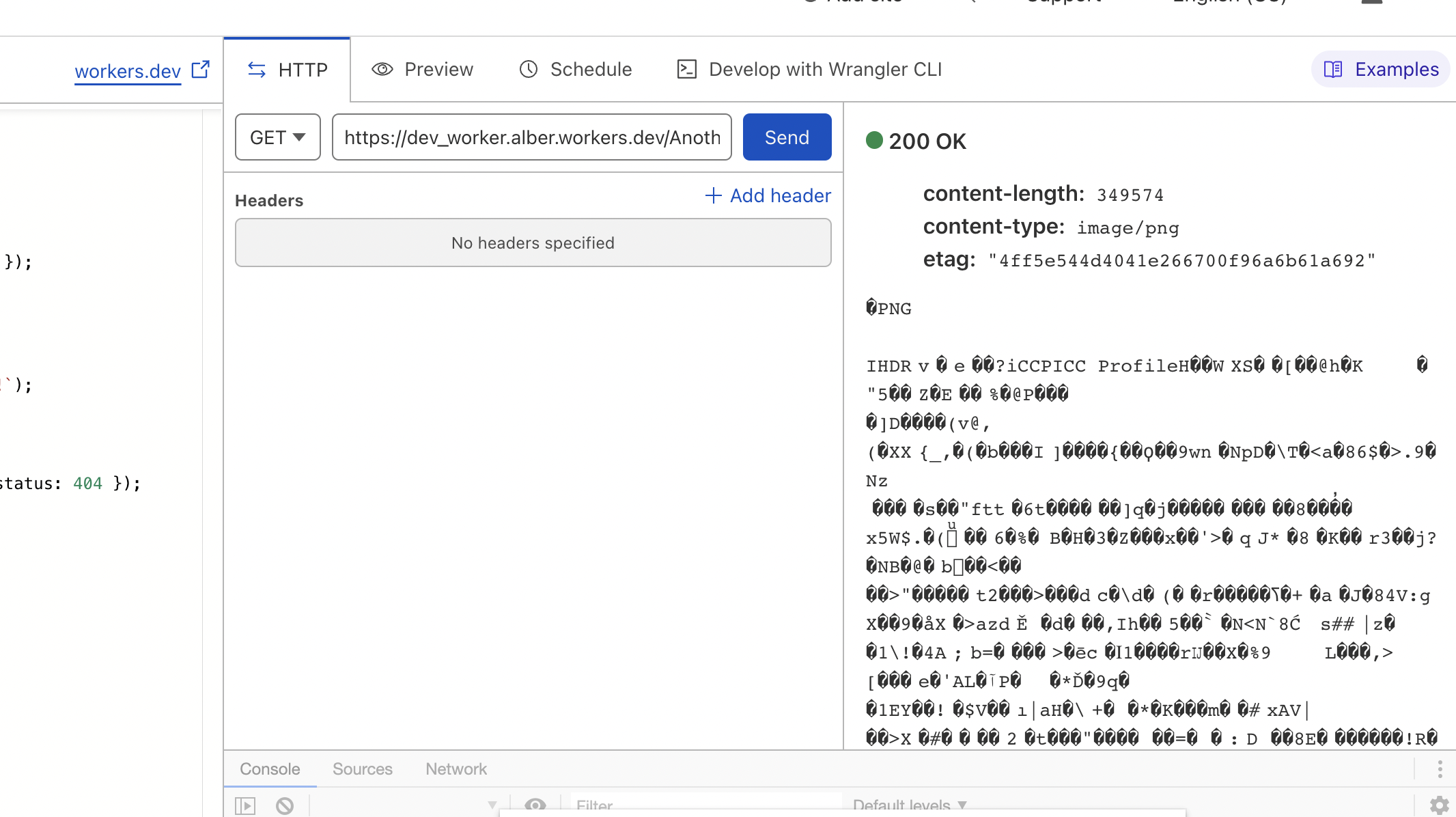
5 Replies
Looks like you might be missing the await on the fetch for the image
I just added an await there and I'm still getting the same {}
That endpoint responds with an image, and you're trying to treat it as JSON?
I was under the assumption that I'd still be able to print its name with object.name if I've gotten it back. Is that wrong? How would I check whether the image has arrived?
Just in case we're talking about different parts, I'm talking about the
api/image/[id] route
you posted, the last code block.
You can't JSON.stringify an image, it'll just throw an error
If you want to check if your image succeeded, just check the status code. You can use the response.ok
property which returns true if the status code is in the success range of 200-299.
You could do something like this, instead
Which would change your code to something like this:
Should work fine. I tested on workers though not next.js
Edit: Thinking about this again, you should probably either respond with the status code it returns (object.status) if not ok
, or have a separate check for if the status code is 404 else respond with errors. Always responding with 404 for any non-200 code is probably going to confuse you at some point in the future when you do have a worker problem or something.
It looks like you posted and deleted a longer message about an issue with the image. Might be better off asking in the next.js discord (I believe there is one), or reposting it again, as I didn't catch the full thing