ā How to structure funcs that calls funcs from static cast
i am getting error CS0120 - https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/compiler-messages/cs0120
example 2 is my situation (and solution), but should i invoke an instance of itself in Main() - to call a func, and i'd need to invoke another instance there to call further funcs? if nothing is passed then how does the contents of main (thus the instance) get handed to func1 to know the instance to call func2...
in my mind these will all be running their own main() instance, is it not recursive? i'm misunderstanding something fundermental here
125 Replies
what line are you getting that error on?
also it doesn't make much sense to create an instance of the same class you're already an instance of

the three rand() calls
i got messing with them because i made rand() static but then the values were held each time
i need them to clear when the func call ends (private?)
how are you running/debugging this?
yeah that's a huge issue
why aren't you using an IDE?
unnecessary bloat
it's... really not?
what?
the whole point of an ide is to properly assist you with coding
my code is very simple i fail to see why i'd need an IDE to get that much working
because there are already compile errors with that code
visual studio is 70 something gb these days isn't it?
that code shouldn't even be allowed to run in the first place
what????
no, obviously not
it was last time i uninstalled it
hahaha
then you installed a bunch of unnecessary components
you can also just get VSCode, Fleet, or Rider
i don't see how this is relevant to CS0120
that error does not get thrown
literally says it 3 times
Ero#1111
REPL Result: Failure
Exception: CompilationErrorException
Compile: 761.116ms | Execution: 0.000ms | React with ā to remove this embed.
come on
if you got a compile error it's probably cause i omitted static on main() lol
just put static back there it'll work
Ero#1111
REPL Result: Success
Console Output
Compile: 701.481ms | Execution: 63.841ms | React with ā to remove this embed.
no error gets thrown
get an IDE
code properly
i dont think that executed anything
and don't use .net framework (jesus christ)
of course it did
because there's no static call
why would there be?
if you truly want to stay IDE-less (do not, i beg you), at least install .net 7 (sdk and runtime) and use the
dotnet run
command instead of csc
you've answered 4 questions i didn't ask for and flat avoided the one question i did ask
the 4 questions you answered had no relevance to the issue at hand
is this not the answer?
there is no error being thrown
oh, must be fine then
also the compile error is on
Append(col);
because col
is an int
, but Append
takes a string
i'll put it real simple
i'm an old fart i do things oldschool, i don't like IDEs, it's not gonna happen, let it go
the question i asked is from static main(), to call a non static func, i must invoke an instance of it - but then if that func calls another func, how does it get the context of what instance, given i had to invoke an instance to call the first func
instance methods can be called by instance methods
there's absolutely nothing stopping them
it's all the same instance after all
but how does it know
it just... does?
some voodoo behind the scenes?
no? it's one instance
all those methods exist in the context of that one instance
of course the methods get compiled together into your binary
the program just knows "oh, this is an instance method"
and can only be called when they're called on an instance of the class
so static main is run on startup because it's static but it's "orphan" because it was not invoked by anything, an instance is created within it (or above it) and then that instance can run, i figure relying on static to run the program was my mistake here
this is a pretty common pattern, actually
you don't actually have any instance data in the class
so technically you could mark everything static
thats what i was doing initially but it seems to hold persistent state between func calls, all the rand() calls returned the same number lol
but it's not uncommon to do
you create a new instance of
Random
every time you call rand
Random
, by default, takes the current time or something for the seed
since the calls are so fast, the seed will be the same every timeoh ffs
if you truly truly don't want to get an ide (you are just plainly wrong here, by the way), please at least do this;
i am sincerely begging you to stop using .net framework
most of its versions are out of support
it's not cross platform (modern .NET is)
and it's so much older and less performant
idk how to even get around that because if i do get it working and it trashes content after each run it won't even know if the instance exists prior, is that the only way to get a random number
i can't do this i'm afraid, my w10 is broken no joke lol
no microsoft store or "extra features" work
so reinstall
not so easy, it's a ML350E G8
windows 10 does NOT like to run on here baremetal
well, it runs fine, it doesn't want to install
https://get.dot.net then
Microsoft
Download .NET (Linux, macOS, and Windows)
Free downloads for building and running .NET apps on Linux, macOS, and Windows. Runtimes, SDKs, and developer packs for .NET Framework, .NET, and ASP.NET.
This release is only compatible with Visual Studio 2022 (v17.4):/
irrelevant
that's a hint for when you use vs at all
the compiler still works?
of course
any ideas where the compiler ends up
why would that matter?
well i need to run the compiler no?
dotnet run
it's added to your PATH, you don't need to look for iti pointed it to the cs file but it wants a whole project structure
oh
i should read what it says that helps
you don't even need to specify the project
you just need to execute
dotnet run
in the project directory
doesn't want to run in the directory either
you did
dotnet new console
?i did not, it's made a csproj file
do you figure perhaps that's the issue
lol yes it's upset with a few things hold on
error CS0101: The nam espace '<global namespace>' already contains a definition for 'TUI'
you must have 2 type declarations with the same name
i don't see how though
neither do i
i don't see anything
since you're not sharing anything
ya hold on i aint so fast
i didn't leave a static anywhere so it should be invoked with TUI tui = new();
i don't know what your project makeup is at this point
that is it entirely
no, you must have a csproj file
this cannot be all of your files
and i moved the code to "program.cs" which it made
oh heck
it was picking up the old cs file aswell
the exe it made doesn't hook the existing console instance
and why would it
that doesn't even make sense
it's its own program
thats how the old compiler works and how i'd expect it to
it's not gonna hook to anything
you built an entirely new executable
you're not running it in some compiler
correct
dotnet run
is gonna run in your console
just... execute it in an open consoleand when i run the exe from console it should
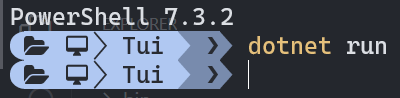
works fine
ya that's what i'm trying but it flashes up a new console window for a few milliseconds which tells me it's making a new terminal, running, and closing, it should hook the existing terminal which called it and end back at the command line prompt no?
no, it should not
that does not make sense
thats how the old one worked :\
when you start discord, it doesn't open in an existing console either, does it
so why should this
it's a completely separate executable
you could send this executable to anyone, and as long as they have the runtime installed, they could run it
https://cqdx.uk/i/mgdM.mp4
you see the difference
one seems to be functional code, the other seems not to be
actually, no
the code you sent here
literally does nothing
so that's just expected
there is no output
no nothing
so obviously it's not going to display anything
hold on sorry
fun
discord wont let me send it but
https://cqdx.uk/i/8WOK.txt
ammended code with output (but still broken)
reverted to last "working" to get this compiler running
oh wait hold on
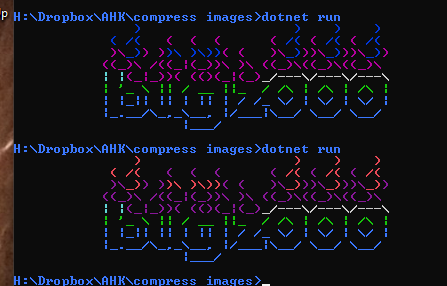
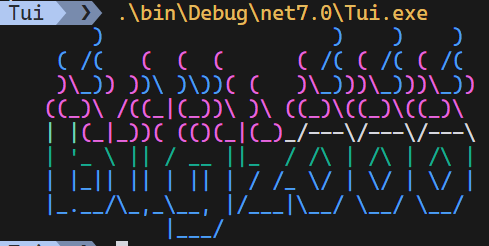
?
well now the rand is updating
š¤
you see that right
no, i don't know what your code does
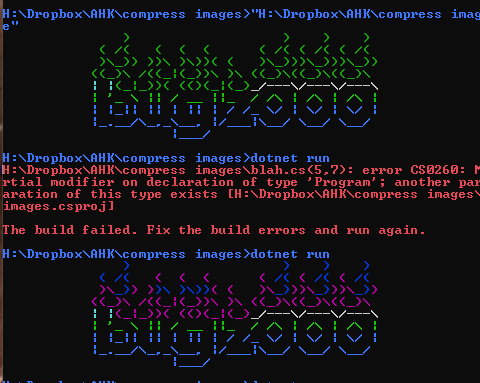
top, solid block color, rand was returning the same each time
bottom, it is not
to be clear, bottom is intended functionality
but i'm curious what changed
besides a newer compiler, because that's some core functionality that's different
UwU? š š
i don't know
but you can use
Random.Shared
there anyway
it's a globally shared instance of Random
don't need to allocate a new one each call
that requires no invoking? it is implicit
it IS implicit neat
and honestly, therefore, that function is kinda pointless
you can just do
int col = Random.Shared.Next(2, 15);
yup haha
a lot of this is boilerplate as i'm moving a big project to C#
porting languages so a lot of this is "mimicing" the functions i made in the other language
required there, not so much here, but for ease of moving i'll do it
fair enough
well it APPEARS to be working, and it hooks the instance too as before
and rand magically works through voodoo means
although i'm still relying on static here
could very well be that they changed the internal implementation of
Random
so perhaps the time seed intervals are smaller
or other stuff
which is why you wanna be up to date on .net versions, better features
your class here doesn't use any instance data
so making everything static is finethat is a valid structure?
have a main class that self invokes and go from there
sure
you'd normally use top level statements here, and just have classes for the different steps
i started like this as it's what i recall from C++ classes but that was nearly 20 years ago
like for example, i would call this
Logo
or something, and have the method that actually does the showing be named Show
or somethingclasses in the academic sense*
mmm well a lot of my console stuff in my other language
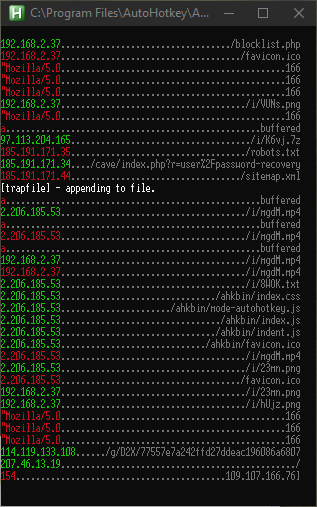
one is running right here infact lol
uses a markup chr(1) to chr(15) to denote what color to set for that part
makes setting the color inline much easier
the append func detects these and substitutes them for whatever "color change" may be, different per language ofc
and that's pretty much as far as i got
so this class is more of a terminal "wrapper" of sorts which i like to include in all my programs
Autohotkey is not an ideal language for hamming distance though so it's about time i shifted to something more capable
yes you can laugh, ahk is easy to use though š
hey i got top layer things working too
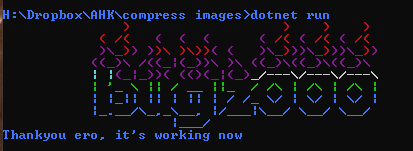
i'd probably do something like this
keep in mind that
Random.Next
's max value is exclusive
so you need 2, 16
if you want it to be able to generate 15aha good call
{c2} is your own markup?
no
that's an interpolated string
$interpolation
String interpolation is the preferred way of building strings in C#. It is easier to read than concatenation.
For example:
can be written as:
OH FK
its expression mode
thats so much easier
(also you never use
c1
/col
in your code)ya c1/col is a big block of nonsense i omitted from testing
its just big ascii art i put in all my consoles it's not important for this
or rather it's the color for it *
i re-make the whole consolecolors array each time i run a line aswell this is much better
you also did
loopCounter++
before adding the thing to the dictionary, so the keys start at 1 instead of 0
which is why i had to do _consoleColors[c - 1]
yes thats because AHK starts at 1 so all my colors are from 1
it was intentional but honestly i could do with updating the color markup a bit
given all the colors are in different places
guess they do start at 0 but nobody uses black
sorry that's an assoc array so colors[1] or colors["black"] would return 0, typeless coding you can do some cursed stuff
double deref is another fun thing lol
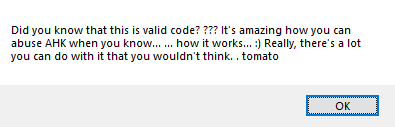
some fun
ugh
ahhaha
it shouldn't exist but it does
you have instead of c12 did you miss it
no it works
nevermind ignore that
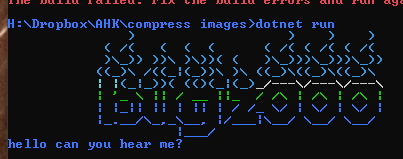
the colors are random it just landed the same by chance lol
those shouldnt' actually be random oddly enough - but it is all working š thankyou ā¤ļø
https://www.youtube.com/watch?v=fjWmSeoLOxc this is the terminal system i'm recreating either way, then i'll port my code to the new platform
thankies for your help ā¤ļø i will no doubt be back at some point with some other silly issue :p but for now i bid you gratitude and farewell
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.