Entity Framework - DbUpdateConcurrencyException due to a trigger on the table
I've been dealing with a problem for a very long time, and I've got no idea how to fix it. I'm a bit of a noob at Entity Framework, so any help appreciated.
After a very long period of trial and error, I found that the table has a trigger on it, which must update some entities. The result is the above exception.
I have no idea how to fix this, my code is extremely simple.
Any help would be appreciated.
11 Replies
My guess is that when
if (routeCard.QtyFinished >= routeCard.BatchQty)
returns false, this error appearsactually it's only when it enters the if statement.
await _myDbContext.SaveChangesAsync();
might have expected atleast one change, but there were noneIf it does not enter the if statement, it's always fine.
Hmmmm, I'm not sure
Maybe you are assigning the same values to it, somehow
nope, definitely not. Checked numerous times.
Are there actual values on
StatusDate
that can represent DateTime.Now
?
This code looks fine to me, I have no clue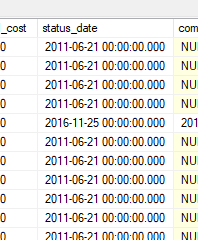
Thing is when I remove the triggers it works
What trigger?
there is a trigger on the table that updates a bunch of stuff, but not this particular table