❔ Callback Url Always Null
Here's the two functions involved
I don't know why but the returnurl is always null.
8 Replies
This is pretty much copied from the docs
well I'm going to assume theres some case sensitivity, so you should probably use
returnUrl
in all the places to be consistentI'll look at that right now
How would that make it function differently when the only use of that variable is in those particular methods?
because it is functioning now
your generating a url here
var redirect = Url.Action("ExternalLoginCallback", "Account", new { ReturnUrl = returnurl });
passing ReturnUrl
as the expected parameter, but string returnurl = null
won't match if it requires case sensitivityReturnUrl
is referencing the ViewModel iirc, and returnurl
is referencing local variable, which is what I changed to returnUrl
as it's what I used the whole way through before this.
so I just changed casing to match rest of controller, left the ReturnUrl
as is, and it's working. 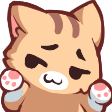
returnUrl
to be passed to it specifically? Here's that function if you wouldn't mind taking a look.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.I understand now. It is expecting
returnUrl
because that was the name in original instance.Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.