ASP NET MVC, can't show Collection in View, VS say it is null
Hello, I'm working on a MVC Project for a film website (IMDb like). I have a User class and a Person class with a many to many relationship and want to show a User table in this Person detail view.
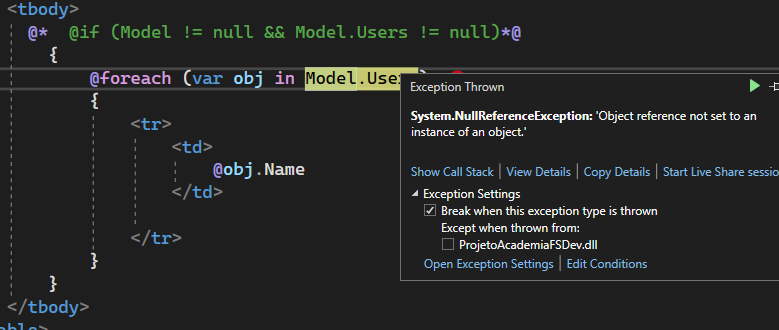
33 Replies
User and Person class is like shown bellow:
Inside my DBContext I have this:
I really don't know where else to search or what to do to correct this
I'm pretty sure my DB is ok and I made the relation correctly, but I simply can't make this work. I created some users and persons in my db and created the relation between those in an intermediary table "UserPeople" that was created by the modelBuilder
Looks like the Model is not passed to the View. Please share Controller code.
@LadyHail thank you for answering, here is the controller code for the view.
In View you need to specify Model type like this:
@model Namespace.Person
I think it was already specified :/
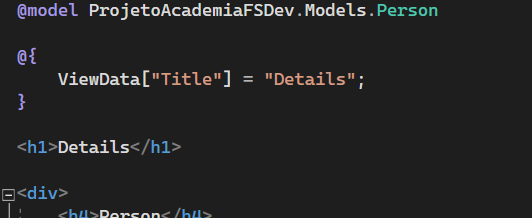
I suggest debugging what's inside person before returning
apparently the User collection inside Person is null when I debug it, but according to the DB, it should not be. Person1 should have 3 users there
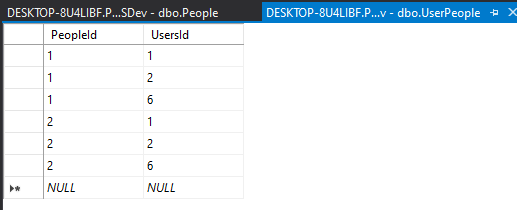
i know these questions may sound a bit dumb, is just that this is my first "serious" project, and I'm kind of lost in some parts of it. Mainly because I don't know what to search to learn what is wrong
Is it Entity Framework Core?
yes
I think you can find it useful. The relationship needs to be specified via intermediate table. https://www.entityframeworktutorial.net/efcore/configure-many-to-many-relationship-in-ef-core.aspx
Configure Many-to-Many Relationships in Entity Framework Core
Learn how to configure a many-to-many relationship using Fluent API in Entity Framework Core.
I had an intermediate table before, and it was giving me the same error
I tried deleting it and making the relation in a different way (this way), but that didn't fixed it :/
I'll make the intermediate table again and try it!
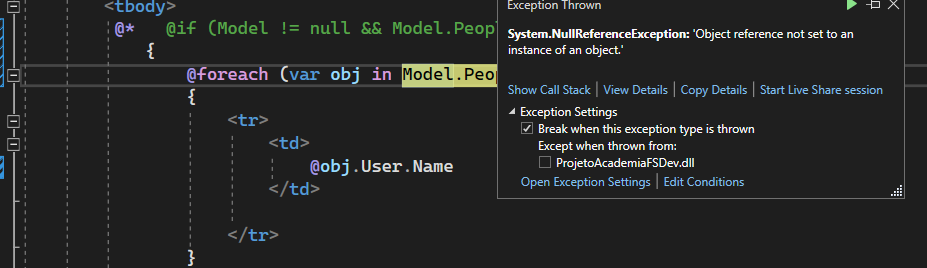
same thing ðŸ˜
I created the intermediate one, dropped my DB, made a new one
DBContexto
The only difference I see is User -> Id. Following docs it should be UserId and same for person.
in the DBContext?
In class definition
In the collections or at the Intermediate class?
In the User class property Id rename to UserId
ok
Same for Person. Rename Id to PersonId.
It shouldn't make a difference, but lets try.
well, it broke my code lol
not building, and cant update db
You need to drop db and recreate
😠😠ðŸ˜
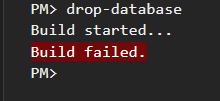
I'll try again, just a minute
I dropped it on MSSMS
still cant update
it is not building and it dont show me any errors too
well, I restored to the last saved version
the original one of the post
isn't it possible that the error is just on the code in the cshtml?
You van try to debug cshtml. But if its null on Controller then it will be null im view
I was thinking if there is another way to write what I want to do inside that foreach
The syntax is valid. I'm pretty sure if you follow the tutorial it'll work
made all the changes, following the tutorial, still the same error
it says that PeopleUsers for this Person is null
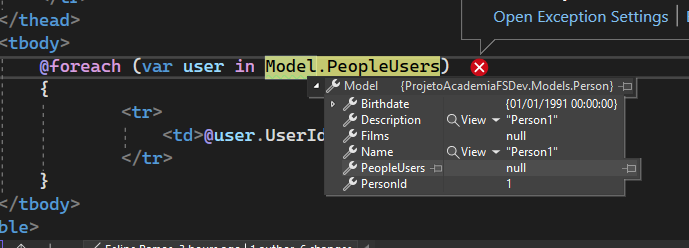
One more idea - in controller before .FirstOrDefault add .Include(x => x.peopleUsers)
i'll try it
IT WORKED, THANK YOU SO MUCH
someone said something similar in the chat, but I couldnt get it to work
you saved my life!
You're welcome