❔ ✅ I can't convert List<BaseClass> to List<T> where T: BaseClass.
I have a
List<List<Component>>
. Component
is a base class, and each item in this outer list is actually a List with different sub-classes of Component
. At run-time I expect it to look like this:
Everything works nicely besides this - I can't find a way to get for example List<SubComponentA>
from the outer list. Here's my current attempt:
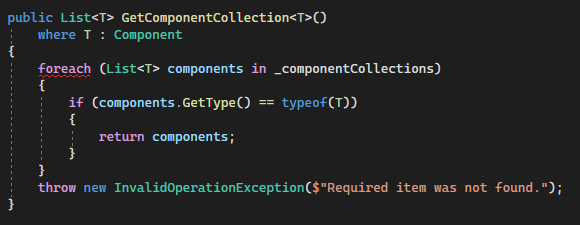

32 Replies
Visual Studio propses this fix:
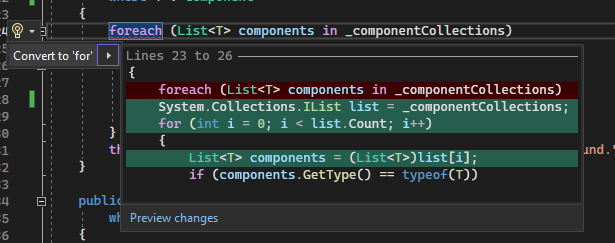
Which gets rid of the error, but still throws an exception at runtime
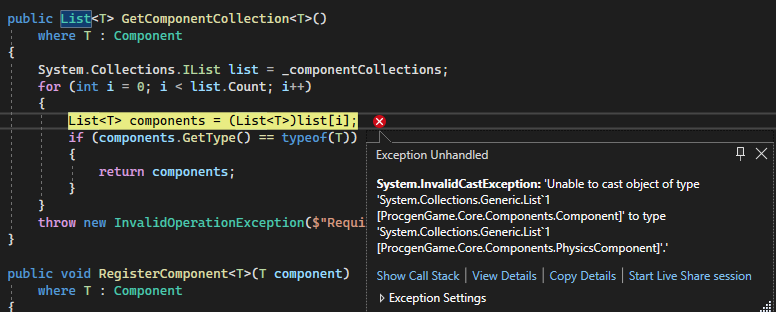
You can't do that because it would allow a
List<SubClass>
to be converted to a List<BaseClass>
and then an instance of BaseClass
added to the list, which is invalid since the List<SubClass>
is expecting all instances to be a SubClass
However, if you just want to loop over the list, you can cast it to an IEnumerable<T>
instead of a List<T>
I would like to be able to use
.Add()
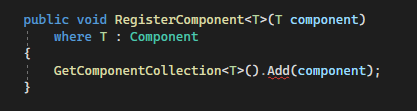
Then you need to make sure that when you originally create the list that it is of the correct type, and store it as just an
IList
in the outer list
Also, using a dictionary is probably better than a list herebtw this still throws an exception, unless you meant something else?
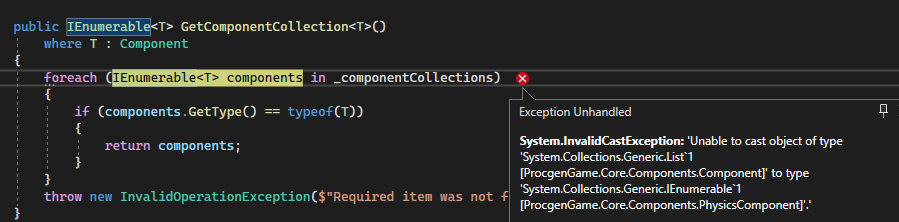
Yeah but then I wouldn't be able to use generics I think
Here's how the method is used

so Types as a key?
I did that before switching to generics
Yup, using the type as the key, and making sure that the
new List<T>()
has the correct type
Unfortunately C# doesn't have a way to do this completely type safely, but as long as you implement your methods correctly it should work fineokay thank you
I'm wondering if im not overengineering this, all I actually need is - a few collections for each subclass of Component, each collection auto-generated for a different subcomponent.
Then a way to get these collections by passing Type or preferably generic T to Read and Add to them.
Everything besides "Get collection" and "Add to collection" is private too.
I ended up with something like this, I'd appreciate anything that can be done to simplify or improve this.
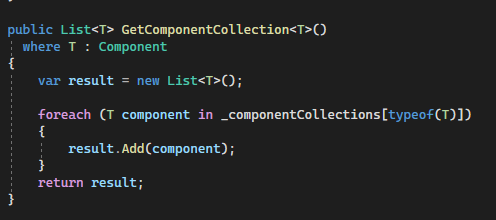
_componentCollections is a Dictionary btw
You can check this: https://learn.microsoft.com/en-us/dotnet/standard/generics/covariance-and-contravariance
Covariance and Contravariance in Generics
Learn about covariance, which allows you to use a more derived type, and contravariance, which allows you to use a less derived type, in .NET generics.
That wouldn't let the caller add to that list
Now I see something. Am I right that this code doesn't preserve the same object reference? I think that's a problem, I wonder if there is a way to preserve that.
I think this doesn't work, no matter what I try
Can you show what you tried?
yeah 1 minute
the problem is that it's a List<BaseClass> to List<T> conversion.
That shouldn't be happening with the code I showed
you have IList right?
Is that a IList or IList<>?
The dictionary stores
IList
yes, but the new List
that adds to the dictionary uses the actual typeI assumed before that you meant the generic
IList<>
, i'll try this instead.
thanks, this works so far. Are there any downsides to this?Why don't you use one list containing a mix of all components?
I think there was one problem here, i'll test this
ToList(), isn't that getting rid of the original reference?
.ToList()
returns a new list, with the same references inside.yeah references inside are the same
but I wanted to be able to use .Add() on that list
so now changes are only preserved in one of the lists i think
not sure exactly what your use case is about, why having a list of lists? you could use an object with different lists as its properties.
Something like this.
Yeah at this moment I'm sure a simple object would be better, but originally I didn't think it'd be this complicated. I just wanted a way to auto-add all components with reflection.
I guess this could be marked as solved as I have a few different ways to do this now
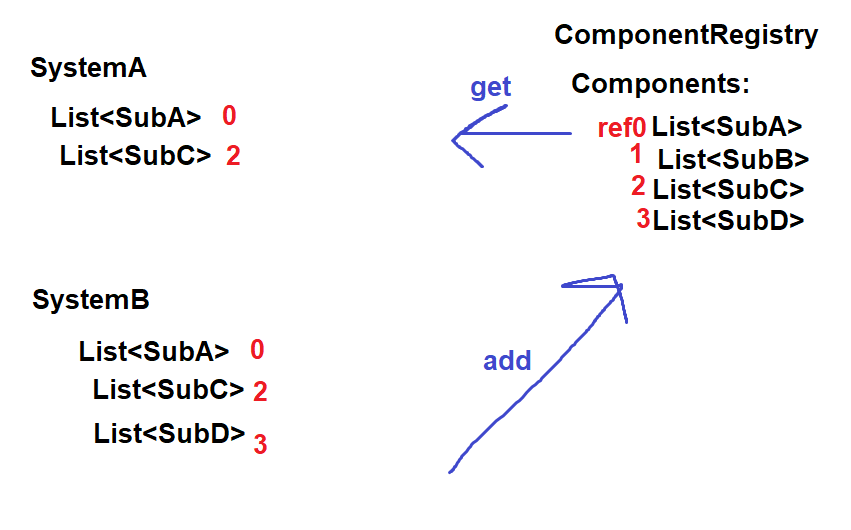
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.